Why Do We Use If/Else Statements in JavaScript? Unpacking Their Importance and Functionality
In the world of programming, decision-making is a fundamental aspect that drives the functionality of applications. Among the myriad of tools available to developers, if/else statements stand out as a cornerstone of logical flow in JavaScript. These powerful constructs allow programmers to dictate how their code responds to varying conditions, enabling dynamic and interactive web experiences. But why do we rely on if/else statements so heavily in JavaScript? Understanding their purpose and functionality can unlock a deeper appreciation for how our favorite websites and applications operate.
At its core, the if/else statement is a mechanism that allows JavaScript to evaluate conditions and execute code based on the outcome of those evaluations. This capability is essential for creating responsive applications that can adapt to user input, environmental factors, or any number of variables. By structuring our code with these conditional statements, we can create logic that mimics human decision-making, making our programs not just functional, but intuitive and engaging.
Moreover, the versatility of if/else statements extends beyond simple binary choices. They can be nested, combined with logical operators, and even integrated with other control structures to create complex decision trees. This flexibility empowers developers to craft intricate workflows and manage multiple scenarios seamlessly. As we delve deeper into the intricacies of if/else statements
Conditional Logic in Programming
If/else statements are fundamental to controlling the flow of a program. They enable developers to implement conditional logic, allowing for different outcomes based on varying inputs or conditions. This ability to branch execution paths based on specific criteria is crucial for creating dynamic and responsive applications.
When a condition evaluates to true, the code within the if block is executed. If the condition is , the code within the else block is executed, if it exists. This mechanism allows for a clear and organized way to handle multiple scenarios within the code.
Syntax and Structure
The basic syntax of an if/else statement in JavaScript is straightforward. Here is the structure:
“`javascript
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is
}
“`
For more complex scenarios, developers can use else if statements to test multiple conditions in sequence:
“`javascript
if (condition1) {
// code for condition1
} else if (condition2) {
// code for condition2
} else {
// code if none of the conditions are true
}
“`
Practical Uses of If/Else Statements
If/else statements can be applied in various scenarios, including but not limited to:
- User Input Validation: Checking if user inputs meet certain criteria (e.g., password strength).
- Feature Toggles: Enabling or disabling features based on user roles or settings.
- Routing Logic: Determining which function or component to execute based on user interactions.
- Error Handling: Managing exceptions and displaying appropriate error messages.
Use Case | Example |
---|---|
User Authentication | if (userLoggedIn) { showDashboard(); } else { showLogin(); } |
Age Verification | if (age >= 18) { allowAccess(); } else { denyAccess(); } |
Feature Availability | if (isProUser) { enableProFeatures(); } else { showStandardFeatures(); } |
Advantages of Using If/Else Statements
The use of if/else statements offers several advantages:
- Clarity: They provide a clear structure for decision-making in code, enhancing readability.
- Flexibility: Developers can easily modify conditions or add additional branches as requirements evolve.
- Efficiency: If/else statements can optimize performance by avoiding unnecessary computations when conditions are checked sequentially.
if/else statements are a cornerstone of programming logic in JavaScript, facilitating the creation of interactive and responsive applications. Their ability to handle multiple conditions makes them indispensable in various programming scenarios.
Purpose of If/Else Statements
If/else statements in JavaScript serve as a fundamental control structure that allows developers to execute different blocks of code based on specific conditions. This capability is crucial for creating dynamic and responsive applications. The primary purposes include:
- Conditional Execution: Execute different code paths based on whether a condition evaluates to true or .
- Flow Control: Direct the flow of execution in a program, enabling complex decision-making processes.
- Error Handling: Provide mechanisms to handle potential errors or unexpected conditions in a controlled manner.
Syntax and Structure
The basic syntax of an if/else statement in JavaScript is straightforward:
“`javascript
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is
}
“`
For more complex scenarios, additional conditions can be included using `else if`:
“`javascript
if (condition1) {
// Code for condition1
} else if (condition2) {
// Code for condition2
} else {
// Code if neither condition is true
}
“`
Use Cases for If/Else Statements
If/else statements are versatile and can be applied in various scenarios, including but not limited to:
- User Input Validation: Checking if user inputs meet specific criteria (e.g., checking if an email format is valid).
- Feature Toggles: Enabling or disabling features based on user roles or application state.
- Routing Logic: Determining which page or component to render based on user actions.
Use Case | Description |
---|---|
User Authentication | Validate credentials and grant access accordingly |
Form Submission | Validate form data before processing it |
Game Logic | Control game flow based on player actions |
Nesting If/Else Statements
If/else statements can be nested, allowing for more granular decision-making processes. However, excessive nesting can lead to code that is difficult to read and maintain. Here’s an example:
“`javascript
if (condition1) {
if (condition2) {
// Code for condition1 and condition2
} else {
// Code for condition1 but not condition2
}
} else {
// Code for not condition1
}
“`
Best Practices
To maintain clean and efficient code when using if/else statements, consider the following best practices:
- Keep Conditions Simple: Avoid overly complex conditions that can obscure the logic.
- Use Early Returns: In functions, consider using early returns to reduce nesting.
- Consistent Formatting: Maintain consistent indentation and spacing for better readability.
Common Mistakes to Avoid
Several pitfalls can lead to bugs or confusion when using if/else statements:
- Missing Curly Braces: Omitting braces can lead to unexpected behavior, especially in nested statements.
- Using Assignment Instead of Comparison: Confusing `=` (assignment) with `==` or `===` (comparison) can lead to logical errors.
- Neglecting Edge Cases: Failing to account for all possible input scenarios may cause unintended outcomes.
By understanding and effectively utilizing if/else statements, developers can enhance the functionality and interactivity of their JavaScript applications, ensuring they respond appropriately to various conditions and inputs.
The Importance of If/Else Statements in JavaScript Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “If/else statements are fundamental to controlling the flow of a program. They allow developers to implement decision-making logic, enabling applications to respond dynamically to user inputs and other conditions.”
Michael Chen (JavaScript Instructor, Code Academy). “Understanding if/else statements is crucial for any JavaScript developer. They serve as the backbone for conditional execution, allowing for the creation of more interactive and user-friendly web applications.”
Sarah Patel (Lead Frontend Developer, Web Solutions Group). “The use of if/else statements enhances code readability and maintainability. They provide a clear structure for decision-making processes, which is essential in complex applications.”
Frequently Asked Questions (FAQs)
Why do we use if/else statements in JavaScript?
If/else statements are used in JavaScript to implement conditional logic, allowing the program to execute different blocks of code based on whether a specified condition evaluates to true or .
How does the syntax of if/else statements work?
The syntax consists of the `if` keyword followed by a condition in parentheses, and a block of code in curly braces. An optional `else` can follow to define an alternative block of code if the condition is .
Can we chain multiple conditions using if/else statements?
Yes, multiple conditions can be chained using `else if` statements, allowing for more complex decision-making processes within the code.
What is the difference between if/else statements and switch statements?
If/else statements evaluate conditions in a sequential manner, while switch statements provide a more efficient way to compare a single expression against multiple possible values, making them more suitable for certain scenarios.
Are there any performance considerations when using if/else statements?
In general, if/else statements are efficient for a small number of conditions. However, for a large number of conditions, switch statements or other data structures may offer better performance and readability.
Can if/else statements be nested in JavaScript?
Yes, if/else statements can be nested within one another to create more complex conditional logic, enabling the handling of multiple layers of conditions effectively.
If/else statements in JavaScript serve as fundamental control structures that enable developers to implement decision-making capabilities within their code. By evaluating conditions and executing specific blocks of code based on the outcome, these statements facilitate dynamic behavior in applications. This capability is essential for creating interactive and responsive user experiences, as it allows the program to react differently under varying circumstances.
The versatility of if/else statements is evident in their ability to handle multiple conditions through nested and chained structures. This flexibility empowers developers to create complex logic flows that can accommodate a wide range of scenarios. Additionally, the use of logical operators within these statements enhances their functionality, allowing for more nuanced decision-making processes. As a result, if/else statements are integral to writing efficient and effective JavaScript code.
In summary, the importance of if/else statements in JavaScript cannot be overstated. They are essential for controlling the flow of execution based on specific conditions, enabling developers to craft applications that respond intelligently to user input and environmental changes. Mastery of these control structures is crucial for anyone looking to excel in JavaScript programming and create robust, interactive web applications.
Author Profile
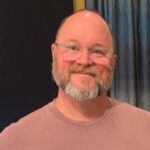
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?