How Can You Build a Website Using Python: A Step-by-Step Guide?
In today’s digital age, having a website is essential for individuals and businesses alike. While many people turn to user-friendly website builders, a growing number are discovering the power and flexibility of programming languages—particularly Python. Known for its simplicity and versatility, Python has emerged as a popular choice for web development, allowing developers to create everything from personal blogs to complex web applications. If you’ve ever wondered how to harness the capabilities of Python to build your own website, you’re in the right place.
Building a website using Python opens up a world of possibilities. With frameworks like Flask and Django, you can streamline the development process while maintaining control over your site’s functionality and design. These frameworks provide robust tools and libraries that simplify tasks such as routing, database management, and user authentication, making it easier for both beginners and seasoned developers to bring their visions to life. Furthermore, Python’s readability and community support mean that you’ll have plenty of resources at your fingertips as you embark on your web development journey.
As you dive deeper into the world of Python web development, you’ll discover not only the technical skills required to build a website but also the creative aspects that make your site unique. From designing an intuitive user interface to implementing interactive features, the process is as much about artistry as it is about coding. Whether you’re
Frameworks for Building Websites with Python
Python offers several frameworks that streamline the web development process, allowing developers to focus on building features rather than dealing with low-level code. Some of the most popular frameworks include:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with an ORM, an admin interface, and various built-in features.
- Flask: A micro-framework that is lightweight and modular, making it ideal for small applications and projects where simplicity is key.
- FastAPI: A modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints.
Choosing the right framework depends on the project requirements, the complexity of the application, and the developer’s familiarity with the technology.
Setting Up a Development Environment
Before you start building your website, it is essential to set up a proper development environment. This typically includes the following steps:
- Install Python: Download and install the latest version of Python from the official website.
- Set Up a Virtual Environment: Use `venv` or `virtualenv` to create an isolated environment for your project.
- Install Required Packages: Use `pip` to install the necessary packages for your chosen framework.
Here is an example of how to set up a virtual environment using `venv`:
“`bash
python -m venv myprojectenv
source myprojectenv/bin/activate On Windows use `myprojectenv\Scripts\activate`
pip install django or flask, fastapi, etc.
“`
Creating a Basic Web Application
Once the environment is set up, you can begin creating your application. Here’s a general outline for building a simple web application using Django:
- Create a Project: Use the command line to create a new Django project.
“`bash
django-admin startproject myproject
cd myproject
“`
- Create an App: Inside the project, create an application.
“`bash
python manage.py startapp myapp
“`
- Define Models: In `models.py`, define the data structures for your application.
- Set Up Views: In `views.py`, create functions to handle requests and return responses.
- Configure URLs: Map URLs to your views in `urls.py`.
Here’s a simple example of what the `urls.py` file might look like:
“`python
from django.urls import path
from . import views
urlpatterns = [
path(”, views.home, name=’home’),
]
“`
Database Integration
Integrating a database is crucial for dynamic web applications. Django, for example, comes with a built-in ORM that supports multiple databases like PostgreSQL, MySQL, and SQLite.
When configuring your database, you will typically define your models, perform migrations, and create a superuser for the admin interface. Below is a sample table structure for a simple blog application:
Field | Data Type | Description |
---|---|---|
id | Integer | Primary key, auto-incremented |
title | String | Title of the blog post |
content | Text | Body of the blog post |
created_at | DateTime | Date the post was created |
This table outlines a basic structure for a blog post, with fields that are common in most content management systems.
Choosing the Right Framework
When building a website using Python, selecting the appropriate framework is crucial. The choice of framework can significantly impact development speed, scalability, and ease of maintenance. Here are some popular frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It includes built-in features for user authentication, database management, and more.
- Flask: A lightweight micro-framework that is easy to set up and offers flexibility. Ideal for small to medium-sized applications where you want more control over components.
- FastAPI: Designed for building APIs quickly and efficiently, FastAPI is based on standard Python type hints and offers automatic generation of OpenAPI documentation.
Framework | Pros | Cons |
---|---|---|
Django | Full-featured, batteries included | Can be heavy for simple apps |
Flask | Lightweight, flexible | Requires additional setup for larger projects |
FastAPI | Fast, asynchronous support | Newer, smaller community |
Setting Up Your Development Environment
To start building with Python, you need to set up your development environment. Follow these steps:
- Install Python: Download and install the latest version of Python from the official website.
- Set Up a Virtual Environment: This helps manage dependencies and keeps your project isolated.
- Use the command: `python -m venv myenv`
- Activate the environment:
- On Windows: `myenv\Scripts\activate`
- On macOS/Linux: `source myenv/bin/activate`
- Install the Framework: Depending on your choice:
- For Django: `pip install django`
- For Flask: `pip install flask`
- For FastAPI: `pip install fastapi[all]`
Creating Your First Application
After setting up your environment, you can create your first application. Here’s a brief overview for each framework:
Django:
- Create a new project: `django-admin startproject myproject`
- Start the development server: `python manage.py runserver`
- Create an app: `python manage.py startapp myapp`
Flask:
- Create a new file named `app.py`.
- Add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the application: `python app.py`
FastAPI:
- Create a new file named `main.py`.
- Add the following code:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
async def read_root():
return {“Hello”: “World”}
“`
- Run the application using Uvicorn: `uvicorn main:app –reload`
Developing Features
Once the basic application is running, you can start developing features. Consider the following components:
- Database Integration: Use ORM (Object-Relational Mapping) tools like Django ORM or SQLAlchemy to manage database interactions.
- Routing: Define routes to manage URLs and link them to functions or views.
- Templates: Use templating engines like Jinja2 (for Flask and FastAPI) or Django templates to serve dynamic content.
- Forms and Validation: Handle user input through forms and validate data before processing.
Deploying Your Website
Deployment is the final step in making your website live. Consider these common platforms:
- Heroku: A cloud platform that supports Python apps and simplifies deployment.
- DigitalOcean: Offers virtual servers where you can manually configure your stack.
- AWS: Provides various services for scalable deployment, although it has a steeper learning curve.
Steps for deployment:
- Prepare your application (ensure requirements.txt is updated).
- Choose a cloud provider and set up your server.
- Transfer your code using Git or FTP.
- Configure your web server (e.g., Nginx or Apache).
- Ensure your application is running and accessible via a web browser.
Expert Insights on Building a Website Using Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Building a website using Python is an excellent choice due to its readability and versatility. Frameworks like Django and Flask streamline the development process, enabling developers to create robust applications efficiently.”
Michael Chen (Web Development Instructor, Code Academy). “When considering how to build a website with Python, it’s essential to understand the importance of choosing the right framework. Django is ideal for larger projects requiring a lot of built-in features, while Flask is better suited for smaller, more flexible applications.”
Sarah Patel (Lead Developer, Digital Solutions Group). “Utilizing Python for web development not only enhances productivity but also fosters a strong community support system. Leveraging libraries and tools such as SQLAlchemy for database management can significantly simplify the backend development process.”
Frequently Asked Questions (FAQs)
What are the basic steps to build a website using Python?
To build a website using Python, you typically follow these steps: choose a web framework (like Django or Flask), set up your development environment, create your project structure, define your routes and views, implement templates for the frontend, connect to a database if needed, and finally deploy your application to a web server.
Which Python web frameworks are recommended for building websites?
Popular Python web frameworks include Django, Flask, FastAPI, and Pyramid. Django is ideal for large applications due to its “batteries-included” approach, while Flask is better suited for smaller projects or microservices due to its lightweight nature.
How do I set up a local development environment for Python web development?
To set up a local development environment, install Python and a package manager like pip. Create a virtual environment using `venv`, activate it, and then install your chosen web framework and any additional libraries required for your project.
Can I use Python for front-end development?
While Python is primarily a back-end language, you can use frameworks like Brython or Transcrypt to write front-end code in Python. However, traditional front-end technologies like HTML, CSS, and JavaScript are still essential for building user interfaces.
What database options are compatible with Python web applications?
Python web applications can work with various databases, including relational databases like PostgreSQL and MySQL, as well as NoSQL databases like MongoDB. ORM libraries like SQLAlchemy or Django’s ORM can simplify database interactions.
How can I deploy my Python website to a live server?
To deploy a Python website, choose a hosting provider that supports Python, such as Heroku, DigitalOcean, or AWS. Prepare your application for production, configure the web server (like Gunicorn or uWSGI), and set up a reverse proxy with Nginx or Apache if necessary.
Building a website using Python involves several critical steps that leverage the language’s versatility and the robust frameworks available. Initially, one must choose an appropriate web framework, with popular options including Flask for lightweight applications and Django for more complex, feature-rich projects. Each framework offers distinct advantages, such as Flask’s simplicity and Django’s built-in functionalities, which can significantly enhance development efficiency.
In addition to selecting a framework, understanding the structure of a web application is essential. This includes the separation of concerns through models, views, and templates, which helps in organizing code and maintaining clarity. Furthermore, integrating databases, such as SQLite or PostgreSQL, is a vital aspect of web development, allowing for dynamic content management and user interactions.
Finally, deploying the website is a crucial phase that requires attention to hosting options, domain registration, and server configurations. Utilizing platforms like Heroku or AWS can streamline this process, enabling developers to focus on building features rather than infrastructure. Overall, mastering these components not only leads to the successful creation of a website but also equips developers with the skills necessary for future projects.
Author Profile
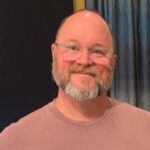
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?