How Can I Use Dplyr Select Without Relying on Column Indexes?
In the world of data manipulation, R’s `dplyr` package stands out as a powerful tool that simplifies the process of transforming and analyzing data frames. One of the most commonly used functions within `dplyr` is `select()`, which allows users to choose specific columns from a dataset. While many users are familiar with selecting columns by their index, there’s a whole realm of possibilities when it comes to selecting columns by their names or other criteria. This article delves into the nuances of using `dplyr`’s `select()` function without relying on column indices, empowering you to write cleaner, more intuitive code.
Understanding how to effectively select columns by name opens up a world of flexibility in data manipulation. Instead of counting columns or remembering their positions, you can use descriptive names that make your code more readable and maintainable. This approach not only enhances clarity but also reduces the likelihood of errors, especially in datasets where the structure may change over time. By leveraging the power of `dplyr`, you can streamline your data analysis workflows and focus more on deriving insights rather than wrestling with column indices.
Moreover, `dplyr` offers a variety of selection helpers and functions that allow for dynamic and conditional column selection. This means you can easily target columns
Dplyr Select Functionality
In the `dplyr` package, the `select()` function is a powerful tool for subsetting columns within a data frame. While many users commonly utilize column names or indices for selection, there are alternative methods available that enhance flexibility and readability. Understanding these methods can streamline data manipulation tasks and improve code clarity.
Selecting Columns by Name
The most straightforward way to select columns is by their names. This method is intuitive and minimizes the likelihood of errors associated with column indexing. For example:
“`R
library(dplyr)
Selecting specific columns by name
selected_data <- data %>%
select(column1, column2, column3)
“`
This code snippet selects `column1`, `column2`, and `column3` from the `data` data frame, creating a new data frame called `selected_data`.
Selecting Columns with Helpers
`dplyr` offers several helper functions to select columns based on patterns, types, or positions, allowing for more dynamic selections.
- Selecting Columns by Pattern: Use the `starts_with()`, `ends_with()`, or `contains()` functions to select columns that match specific text patterns.
“`R
Select columns that start with “sales”
sales_data <- data %>%
select(starts_with(“sales”))
“`
- Selecting Columns by Type: The `where()` function allows users to select columns based on their data type.
“`R
Select all numeric columns
numeric_data <- data %>%
select(where(is.numeric))
“`
- Selecting Columns by Position: Use the `everything()` function to select all columns or combine it with other selectors to refine results.
“`R
Select all columns except the first two
refined_data <- data %>%
select(-1:-2, everything())
“`
Combining Selection Methods
Combining different selection methods can yield powerful results. The following example demonstrates how to select columns based on both names and patterns simultaneously:
“`R
Select specific columns and those that contain “score”
combined_data <- data %>%
select(column1, contains(“score”))
“`
This method enhances the specificity of your selections while maintaining clarity.
Example Table of Selection Functions
The table below summarizes some useful `dplyr` selection functions:
Function | Description |
---|---|
select() | Select columns by name or index. |
starts_with(prefix) | Select columns that start with a specified prefix. |
ends_with(suffix) | Select columns that end with a specified suffix. |
contains(string) | Select columns that contain a specified string. |
where(predicate) | Select columns based on a condition (e.g., type). |
Utilizing these selection techniques can greatly enhance your data manipulation capabilities in R, allowing for clearer and more efficient data analysis workflows.
Using `dplyr::select()` with Column Names
In `dplyr`, the `select()` function is commonly used to choose specific columns from a data frame. While many users may be familiar with selecting columns by their index, selecting by column names is often more readable and less prone to errors.
Selecting Columns by Name
To select columns using their names, you can provide the column names directly as arguments in the `select()` function. Here is the syntax:
“`R
library(dplyr)
data_frame <- data.frame(
A = 1:5,
B = letters[1:5],
C = rnorm(5)
)
selected_data <- data_frame %>%
select(A, C) Selects columns A and C
“`
Tips for Selecting Columns by Name
- Quoting Column Names: If your column names contain spaces or special characters, use backticks to quote them.
“`R
df <- data.frame(`Column 1` = 1:5, `Column 2` = letters[1:5])
selected_df <- df %>%
select(`Column 1`)
“`
- Selecting Multiple Columns: You can list multiple column names separated by commas, or use `c()` to combine them.
“`R
selected_data <- data_frame %>%
select(c(“A”, “C”))
“`
Using Helper Functions
`dplyr` provides several helper functions that facilitate selection based on patterns or conditions in column names:
- `starts_with()`: Select columns that start with a specific string.
- `ends_with()`: Select columns that end with a specific string.
- `contains()`: Select columns that contain a specific string.
Example
“`R
selected_data <- data_frame %>%
select(starts_with(“A”)) Selects columns starting with “A”
“`
Excluding Columns
To exclude certain columns by name, use the negative sign (`-`) before the column name.
“`R
filtered_data <- data_frame %>%
select(-B) Excludes column B
“`
Example Table of Selection Functions
Function | Description |
---|---|
`select()` | Select specified columns by name |
`starts_with()` | Select columns that begin with a specified string |
`ends_with()` | Select columns that end with a specified string |
`contains()` | Select columns that contain a specified string |
`-` | Exclude specified columns |
Advanced Selection Techniques
For more complex selections, you can combine multiple selection functions. For instance, selecting columns that start with “A” and excluding specific columns can be done as follows:
“`R
final_data <- data_frame %>%
select(starts_with(“A”), -C) Selects columns starting with “A” but excludes column C
“`
This approach allows users to maintain clarity and flexibility when manipulating data frames, enhancing both performance and readability in R programming.
Expert Insights on Dplyr Select Beyond Column Indexing
Dr. Emily Chen (Data Scientist, Analytics Innovations Inc.). “Using `dplyr`’s `select()` function without relying on column indices enhances code readability and maintainability. It allows for more intuitive selection based on column names or patterns, which is particularly beneficial in data analysis workflows where datasets may change over time.”
Mark Thompson (Senior R Programmer, Data Solutions Group). “When working with large datasets, selecting columns by name rather than index prevents errors that can arise from changes in column order. This practice is essential for ensuring that your code remains robust and adaptable to evolving data structures.”
Lisa Patel (Statistical Analyst, Insightful Data Services). “The ability to use `dplyr` to select columns dynamically, such as with the `starts_with()` or `ends_with()` functions, allows analysts to create flexible data manipulation scripts. This approach is not only efficient but also aligns with best practices in data science for reproducibility and clarity.”
Frequently Asked Questions (FAQs)
What is the purpose of the `select` function in dplyr?
The `select` function in dplyr is used to choose specific columns from a data frame based on their names rather than their index positions. This allows for more readable and maintainable code.
How can I select columns by name using dplyr?
You can select columns by name using the `select` function with the column names directly as arguments. For example, `select(data_frame, column1, column2)` will return only the specified columns.
Can I use partial matching to select columns in dplyr?
Yes, you can use the `contains()`, `starts_with()`, and `ends_with()` functions within `select` to partially match column names. For example, `select(data_frame, starts_with(“prefix”))` selects all columns that start with “prefix”.
Is it possible to exclude certain columns while selecting others?
Yes, you can exclude columns by using the negative sign (`-`) before the column name. For instance, `select(data_frame, -column_to_exclude)` will return all columns except the specified one.
How do I select columns based on their data type in dplyr?
You can use the `select_if()` function to select columns based on their data type. For example, `select_if(data_frame, is.numeric)` will return only the numeric columns from the data frame.
Can I rename columns while selecting them in dplyr?
Yes, you can rename columns during selection using the `rename()` function. For example, `select(data_frame, new_name = old_name)` allows you to select and rename a column simultaneously.
In the realm of data manipulation using the dplyr package in R, the ability to select columns without relying solely on their index positions is a significant advantage. The select function allows users to specify column names directly, which enhances code readability and maintainability. This approach not only reduces the risk of errors associated with changing column orders but also makes the code more intuitive for others who may work with the dataset in the future.
Moreover, dplyr’s select function offers a variety of helper functions that facilitate more complex selection criteria. For instance, users can select columns based on patterns in their names or by using logical conditions. This flexibility allows for efficient data wrangling, especially in large datasets where identifying the correct columns by name can save time and effort compared to counting indices.
Ultimately, leveraging dplyr’s capabilities to select columns by name rather than by index enhances the overall efficiency of data analysis workflows. It encourages best practices in coding by promoting clarity and reducing the likelihood of mistakes. As data analysis continues to evolve, mastering these techniques will be invaluable for analysts and data scientists alike.
Author Profile
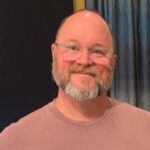
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?