How Can You Easily Set Up SMTP Mail in Laravel Using ReachMail?
In the fast-paced digital landscape, effective communication is paramount, and email remains one of the most powerful tools for connecting with users. For developers working with Laravel, setting up SMTP mail can significantly enhance the functionality of applications, ensuring that messages reach their intended recipients seamlessly. When combined with ReachMail, a robust email marketing platform, Laravel can transform from a simple web framework into a powerful communication hub. This article delves into the essentials of configuring SMTP mail in Laravel using ReachMail, guiding you through the process of optimizing your email delivery and maximizing engagement.
Setting up SMTP mail in Laravel is a straightforward yet crucial step for any developer looking to leverage the framework’s capabilities. By integrating ReachMail, you not only gain access to reliable email delivery but also a suite of tools designed to enhance your marketing efforts. This combination allows developers to manage their email communications efficiently, ensuring that transactional and marketing emails are sent reliably and effectively.
As we explore the intricacies of this setup, you’ll discover the key configurations required to connect Laravel with ReachMail’s SMTP server. From understanding the necessary credentials to troubleshooting common issues, this guide will equip you with the knowledge to streamline your email processes. Whether you’re building a new application or enhancing an existing one, mastering SMTP integration with ReachMail will empower you to
Configuring SMTP Settings
To set up SMTP mail with ReachMail in Laravel, begin by configuring the SMTP settings in your `.env` file. This file is crucial for managing environment variables in your Laravel application. You will need the following credentials from ReachMail:
- MAIL_MAILER: This should be set to `smtp`.
- MAIL_HOST: The SMTP server address provided by ReachMail.
- MAIL_PORT: The port number, typically `587` for TLS or `465` for SSL.
- MAIL_USERNAME: Your ReachMail account email address.
- MAIL_PASSWORD: The password associated with your ReachMail account.
- MAIL_ENCRYPTION: Set this to `tls` or `ssl` based on the port you are using.
- MAIL_FROM_ADDRESS: The email address you want your emails to appear from.
- MAIL_FROM_NAME: The name you want to display as the sender.
Your `.env` file should look something like this:
“`
MAIL_MAILER=smtp
MAIL_HOST=smtp.reachmail.net
MAIL_PORT=587
[email protected]
MAIL_PASSWORD=your-password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME=”Your Name”
“`
Testing the SMTP Configuration
After configuring the SMTP settings, it is essential to test the configuration to ensure that emails can be sent successfully. You can use Laravel’s built-in functionality to send a test email. Create a route in your `web.php` file:
“`php
Route::get(‘/send-test-email’, function () {
\Mail::raw(‘This is a test email from Laravel using ReachMail SMTP’, function ($message) {
$message->to(‘[email protected]’)
->subject(‘Test Email’);
});
return ‘Test email sent!’;
});
“`
Visit `http://your-app-url/send-test-email` to trigger the email. If everything is configured correctly, you should receive the test email at the specified recipient address.
Troubleshooting Common Issues
When setting up SMTP mail with ReachMail, you may encounter several common issues. Here are potential problems and their solutions:
- Authentication Error: Ensure that your username and password in the `.env` file are correct.
- Connection Timeout: Verify that your server can connect to the ReachMail SMTP server. Check firewall settings and ensure that the appropriate ports are open.
- Email Not Received: Check your spam folder. If the email is missing, verify the `MAIL_FROM_ADDRESS` and `MAIL_FROM_NAME` settings.
Issue | Possible Solution |
---|---|
Authentication Error | Verify username and password in the .env file. |
Connection Timeout | Check server firewall settings and ensure ports are open. |
Email Not Received | Check spam folder and validate email settings. |
By following these guidelines, you can successfully set up and troubleshoot SMTP mail with ReachMail in your Laravel application.
Prerequisites for Setting Up SMTP Mail
To successfully configure SMTP mail in Laravel using ReachMail, ensure you have the following prerequisites:
- A Laravel application set up and running.
- A ReachMail account with SMTP access enabled.
- Access to your environment configuration files.
Configuration Steps
To configure your Laravel application to use ReachMail’s SMTP, follow these steps:
- Install the necessary dependencies: Ensure you have the required libraries for sending emails. Run the following command in your terminal:
“`bash
composer require guzzlehttp/guzzle
“`
- Update the `.env` file: Open your Laravel application’s `.env` file and add the following SMTP configuration parameters:
“`plaintext
MAIL_MAILER=smtp
MAIL_HOST=smtp.reachmail.net
MAIL_PORT=587
MAIL_USERNAME=your_reachmail_username
MAIL_PASSWORD=your_reachmail_password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME=”${APP_NAME}”
“`
Replace `your_reachmail_username`, `your_reachmail_password`, and `[email protected]` with your actual ReachMail credentials and desired email address.
- Clear the configuration cache: After updating the `.env` file, clear the configuration cache to ensure Laravel uses the new settings:
“`bash
php artisan config:cache
“`
Testing Your SMTP Configuration
To verify that your SMTP configuration is working correctly, you can send a test email. Here’s how:
- **Create a Mailable class**: Generate a new Mailable class using Artisan:
“`bash
php artisan make:mail TestEmail
“`
- **Define the email content**: Open the newly created `TestEmail.php` file in the `app/Mail` directory and modify the `build` method to include your desired content:
“`php
public function build()
{
return $this->view(’emails.test’)
->subject(‘Test Email from Laravel’);
}
“`
- Create a Blade view: Create a new Blade template for the email content at `resources/views/emails/test.blade.php`:
“`html
Hello,
This is a test email sent from Laravel using ReachMail SMTP.
“`
- **Send the email**: Use a route or controller to send the test email:
“`php
use App\Mail\TestEmail;
use Illuminate\Support\Facades\Mail;
Route::get(‘/send-test-email’, function () {
Mail::to(‘[email protected]’)->send(new TestEmail());
return ‘Test email sent!’;
});
“`
Replace `[email protected]` with the actual recipient’s email address.
Troubleshooting Common Issues
If you encounter issues while sending emails, consider the following troubleshooting tips:
- Check SMTP credentials: Ensure your username and password in the `.env` file are correct.
- Verify port settings: Confirm that you are using the correct port (587 for TLS).
- Review firewall settings: Ensure your server allows outbound connections on the SMTP port.
- Examine error logs: Check Laravel’s log files located in `storage/logs/laravel.log` for any relevant error messages.
Issue | Possible Solution |
---|---|
Authentication failure | Check username and password |
Connection timeout | Verify network and firewall settings |
Email not received | Check spam/junk folder and SMTP logs |
Expert Insights on Setting Up SMTP Mail with ReachMail in Laravel
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Integrating SMTP mail with ReachMail in Laravel requires a thorough understanding of both the Laravel framework and the ReachMail API. Proper configuration of the .env file is crucial to ensure seamless email delivery and to avoid common pitfalls such as authentication errors.”
Michael Thompson (Email Marketing Specialist, Digital Strategies Group). “When setting up SMTP with ReachMail in Laravel, it is essential to consider the email sending limits imposed by your ReachMail account. This ensures that your application adheres to best practices for email marketing and maintains a good sender reputation.”
Laura Chen (Laravel Developer Advocate, Open Source Community). “Utilizing Laravel’s built-in mail functionality in conjunction with ReachMail can enhance your application’s email capabilities. Leveraging Laravel’s queued jobs for sending emails can significantly improve performance and user experience, especially during high-traffic periods.”
Frequently Asked Questions (FAQs)
How do I set up SMTP mail in Laravel with ReachMail?
To set up SMTP mail in Laravel with ReachMail, you need to configure your `.env` file with the ReachMail SMTP server details. Set the `MAIL_MAILER`, `MAIL_HOST`, `MAIL_PORT`, `MAIL_USERNAME`, `MAIL_PASSWORD`, and `MAIL_ENCRYPTION` variables according to the credentials provided by ReachMail.
What credentials do I need for ReachMail SMTP setup?
You will need the SMTP server address, port number, your ReachMail account username, and password. Additionally, you may need to specify the encryption method, typically `tls` or `ssl`, depending on the port used.
Can I test the SMTP configuration in Laravel?
Yes, you can test the SMTP configuration by using Laravel’s built-in command `php artisan tinker` to send a test email. Alternatively, you can create a route or controller method that uses the `Mail` facade to send a test email.
What should I do if my emails are not sending?
If emails are not sending, first check your `.env` configuration for any typos or incorrect values. Ensure that your ReachMail account is active and not exceeding any sending limits. Additionally, check the Laravel logs for any error messages that may indicate the issue.
Is there a way to handle email failures in Laravel?
Yes, Laravel provides a robust way to handle email failures through the `Mail::failures()` method. You can implement this in your email sending logic to capture and log any failures that occur during the sending process.
Can I customize the email content sent through ReachMail in Laravel?
Yes, you can customize the email content by creating Mailable classes in Laravel. This allows you to define the subject, body, and any attachments for your emails, providing flexibility in how you communicate with your recipients.
setting up SMTP mail with ReachMail in a Laravel application is a straightforward process that enhances email delivery and management capabilities. By configuring the necessary settings in the Laravel environment file, developers can seamlessly integrate ReachMail’s SMTP services. This integration allows for improved email tracking, analytics, and overall performance, which are essential for any application that relies on effective communication with users.
One of the key takeaways from this process is the importance of ensuring that all SMTP credentials are correctly configured within the Laravel application. This includes specifying the correct host, port, username, and password. Additionally, enabling SSL or TLS as required by ReachMail can significantly improve the security of email transmissions. Proper configuration not only facilitates successful email delivery but also enhances the application’s credibility and user trust.
Furthermore, leveraging ReachMail’s features such as list management and campaign tracking can provide developers with valuable insights into user engagement. By utilizing these tools, businesses can optimize their email strategies and improve overall customer interaction. In summary, integrating ReachMail’s SMTP services into a Laravel application not only simplifies email management but also empowers developers to create more effective communication channels with their users.
Author Profile
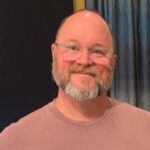
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?