How Can You Extract the Body from SOAP XML?
In the world of web services, SOAP (Simple Object Access Protocol) stands as a stalwart protocol for exchanging structured information. While its XML-based messaging format is robust and widely used, developers often find themselves navigating the intricacies of SOAP envelopes to extract the essential data they need. One common task that arises in this context is how to efficiently retrieve the body from a SOAP XML message. Understanding this process is crucial for anyone working with APIs or web services, as it lays the groundwork for effective communication between systems. In this article, we will delve into the methods and best practices for extracting the body from SOAP XML, empowering you to streamline your development workflow and enhance your applications.
When working with SOAP messages, the structure of the XML can seem daunting at first glance. Each message is encapsulated in an envelope, which contains a header and a body. The body is where the actual data resides, and accessing it is vital for processing the information correctly. Developers often encounter challenges when trying to parse these messages, especially when dealing with various programming languages and libraries that handle XML differently. This article will guide you through the fundamental concepts and techniques that will simplify the extraction of the body from SOAP XML.
As we explore the methods for retrieving the body, we will also highlight
Extracting the Body from SOAP XML
To extract the body from a SOAP XML message, it is essential to understand the structure of SOAP. A SOAP message consists of an envelope that contains a header and a body. The body contains the actual message intended for the recipient.
When parsing SOAP XML, the body is typically accessed using XML parsing techniques. Below are some common methods used in various programming languages to extract the body from a SOAP message.
Using Python with ElementTree
In Python, the `xml.etree.ElementTree` module is commonly used for parsing XML. Below is a sample code snippet to extract the body from a SOAP XML message.
“`python
import xml.etree.ElementTree as ET
soap_message = ”’
root = ET.fromstring(soap_message)
body = root.find(‘{http://schemas.xmlsoap.org/soap/envelope/}Body’)
result = ET.tostring(body, encoding=’unicode’)
print(result)
“`
This code snippet performs the following steps:
- Parses the SOAP XML string into an ElementTree object.
- Finds the `` element using the appropriate namespace.
- Converts the body element back into a string for further processing.
Using Java with JAXB
In Java, the Java Architecture for XML Binding (JAXB) can be used to unmarshal SOAP messages. Below is an example of how to extract the body using JAXB.
“`java
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPEnvelope;
import javax.xml.soap.SOAPMessage;
SOAPMessage soapMessage = …; // Assume this is your SOAP message
SOAPEnvelope envelope = soapMessage.getSOAPPart().getEnvelope();
SOAPBody body = envelope.getBody();
“`
This method involves:
- Creating a `SOAPMessage` object.
- Accessing the SOAP envelope and then the body.
Common Libraries for SOAP Parsing
Different programming languages have libraries tailored for SOAP parsing. Below is a table summarizing some of these libraries:
Language | Library | Usage |
---|---|---|
Python | xml.etree.ElementTree | Basic XML parsing |
Java | JAXB | XML binding and parsing |
C | System.Xml | XML handling with LINQ |
JavaScript | xml2js | Parsing XML to JavaScript objects |
By utilizing these libraries, developers can efficiently handle SOAP XML and extract necessary components such as the body. Each library provides specific functions and capabilities tailored to the respective programming environment.
Extracting the Body from SOAP XML
To retrieve the body from a SOAP XML message, it is essential to understand the structure of SOAP messages. SOAP messages typically consist of an envelope, header, and body. The body contains the actual message being sent.
SOAP Message Structure
A standard SOAP message looks like the following:
“`xml
“`
To extract the body, focus on the `
Methods for Extraction
The extraction process can vary based on the programming language or tool you are using. Below are common methods:
Using Python with `xml.etree.ElementTree`
Python provides a straightforward way to parse XML using the `xml.etree.ElementTree` module. Here’s how to extract the body:
“`python
import xml.etree.ElementTree as ET
Sample SOAP XML
soap_xml = “””
Parse the XML
root = ET.fromstring(soap_xml)
Extract the Body
body = root.find(‘{http://schemas.xmlsoap.org/soap/envelope/}Body’)
print(ET.tostring(body, encoding=’unicode’))
“`
Using Java with JAXP
In Java, you can use the JAXP (Java API for XML Processing) to parse and extract the body:
“`java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
public class SoapExtractor {
public static void main(String[] args) throws Exception {
String soapXml = “
+ “
+ “
+ “
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(soapXml)));
NodeList body = doc.getElementsByTagNameNS(“http://schemas.xmlsoap.org/soap/envelope/”, “Body”);
// Print Body contents
System.out.println(body.item(0).getTextContent());
}
}
“`
Tips for Efficient Extraction
- Ensure that your XML parser is configured to recognize namespaces, as SOAP often uses them.
- Validate the SOAP message structure before attempting to extract the body to prevent runtime errors.
- Consider using libraries or frameworks that simplify XML handling, such as JAXB in Java or lxml in Python.
Common Libraries for XML Parsing
Language | Library/Framework | Purpose |
---|---|---|
Python | xml.etree.ElementTree | Basic XML parsing |
Python | lxml | Advanced XML and HTML parsing |
Java | JAXP | XML parsing and transformation |
C | System.Xml | XML parsing and manipulation |
Utilizing these methods will streamline the process of extracting the body from SOAP XML messages in your applications.
Expert Insights on Extracting Body from SOAP XML
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “Extracting the body from SOAP XML is crucial for effective web service communication. It involves parsing the XML structure to isolate the message content, which can be achieved using various libraries such as JAXB or DOM in Java, ensuring that the data is both accessible and usable.”
Michael Chen (Lead Developer, Cloud Integration Services). “When dealing with SOAP XML, it is essential to understand the envelope structure. The body typically contains the actual message payload, and using XPath expressions can greatly simplify the extraction process, allowing developers to efficiently retrieve the necessary information.”
Sarah Thompson (XML Data Specialist, Data Dynamics). “To effectively get the body from SOAP XML, one must consider the namespaces and potential complexities of the XML schema. Utilizing tools like XSLT can streamline the transformation and extraction process, making it easier to manipulate and use the data in applications.”
Frequently Asked Questions (FAQs)
What is SOAP XML?
SOAP XML is a protocol used for exchanging structured information in web services. It relies on XML to encode messages and typically operates over HTTP or SMTP.
How do I extract the body from a SOAP XML message?
To extract the body from a SOAP XML message, parse the XML structure and locate the `
What tools can I use to parse SOAP XML?
You can use various programming languages and libraries to parse SOAP XML, including Python with `xml.etree.ElementTree`, Java with `javax.xml.parsers`, or tools like Postman and SoapUI for testing.
Is there a specific format for the SOAP body?
Yes, the SOAP body must conform to the XML schema defined by the web service. It typically contains the request or response data structured according to the service’s WSDL (Web Services Description Language).
Can I manipulate SOAP XML bodies programmatically?
Yes, you can manipulate SOAP XML bodies programmatically using libraries in languages such as Python, Java, or C. These libraries allow you to modify, add, or remove elements within the SOAP body.
What should I do if the SOAP body is empty?
If the SOAP body is empty, check the service endpoint and request format. Ensure that the request is correctly formatted and that the service is operational. Review any error messages returned for further insights.
In summary, extracting the body from SOAP XML involves understanding the structure of SOAP messages, which typically consist of an envelope, header, and body. The body contains the actual data being transmitted, and accessing it requires parsing the XML format correctly. Various programming languages and libraries provide tools to facilitate this process, making it easier for developers to interact with web services that utilize SOAP for communication.
Key takeaways include the importance of familiarizing oneself with XML parsing techniques and the specific SOAP standards. Utilizing libraries such as JAXB in Java, or ElementTree in Python, can significantly streamline the extraction process. Moreover, understanding the nuances of the SOAP protocol, including how to handle namespaces and potential errors, is crucial for effective data manipulation.
Overall, mastering the extraction of body content from SOAP XML is essential for developers working with web services. It enhances their ability to integrate and utilize external APIs, ultimately leading to more robust and efficient applications. Continuous learning and practice in this area will yield better results and improve overall proficiency in handling SOAP-based communications.
Author Profile
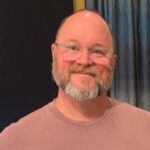
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?