Why Am I Seeing ‘SyntaxError: EOL While Scanning String Literal’ in My Code?
### Introduction
In the world of programming, even the most seasoned developers can find themselves entangled in the web of syntax errors. Among these, the `SyntaxError: EOL while scanning string literal` stands out as a common yet perplexing issue that can halt your coding progress. This error typically arises in languages like Python when the interpreter encounters an unexpected end of line (EOL) while trying to process a string. Whether you’re a beginner just starting your coding journey or an experienced coder debugging a complex script, understanding this error is crucial for writing clean, functional code.
This article delves into the intricacies of the `EOL while scanning string literal` error, exploring its causes and how to effectively resolve it. We will discuss the importance of proper string formatting, the nuances of quotation marks, and the common pitfalls that lead to this frustrating error. By the end, you’ll not only grasp the underlying principles that cause this syntax error but also gain practical tips to prevent it from disrupting your coding workflow in the future.
Join us as we unravel the mystery behind this error and empower you with the knowledge to write error-free code, ensuring your programming experience is as smooth and efficient as possible.
Understanding the Error
The `SyntaxError: EOL while scanning string literal` occurs in Python when the interpreter reaches the end of a line (EOL) while still expecting to find a closing quotation mark for a string. This error typically indicates that you have not closed your string properly, leading Python to believe that the string continues beyond the line where it actually ends.
Common scenarios that lead to this error include:
- Forgetting to close a string with a matching quotation mark.
- Using mismatched quotation marks (i.e., starting with a single quote and ending with a double quote).
- Accidentally pressing Enter before finishing the string.
To illustrate, consider the following code snippets:
python
# Example of forgetting to close a string
print(“Hello, World)
The above will trigger a `SyntaxError` because the closing quotation mark is missing.
Identifying and Fixing the Error
To resolve this error, carefully inspect the line indicated in the error message. Look for:
- Open quotation marks without corresponding closing marks.
- Inconsistencies in quotation styles.
You can fix the example from above by adding the missing quotation mark:
python
# Corrected version
print(“Hello, World”)
Best Practices to Avoid the Error
To minimize the chances of encountering this error, consider the following best practices:
- Always ensure that every opening quotation mark has a matching closing quotation mark.
- Use a consistent quotation style throughout your code (either single or double quotes).
- Utilize an Integrated Development Environment (IDE) or text editor that highlights matching quotation marks.
Examples of the Error
Here are examples that illustrate the `SyntaxError: EOL while scanning string literal` along with their fixes:
Code Example | Error Message | Fix |
---|---|---|
print(“This is a string | SyntaxError: EOL while scanning string literal | print(“This is a string”) |
message = ‘It’s a sunny day | SyntaxError: EOL while scanning string literal | message = ‘It\’s a sunny day’ |
By following these guidelines and carefully checking your code, you can effectively avoid and resolve the `SyntaxError: EOL while scanning string literal` in your Python projects.
Understanding the Error
The `SyntaxError: EOL while scanning string literal` is a common error encountered in Python programming. This error indicates that the Python interpreter reached the end of a line (EOL) while still expecting a closing quotation mark for a string.
### Causes of the Error
Several scenarios can lead to this error:
- Missing Closing Quote: A string that starts with a quote does not have a corresponding closing quote.
- Improper Line Continuation: Strings that are intended to span multiple lines without proper continuation.
- Use of Different Quotes: Mismatched quotes, such as starting a string with a single quote (`’`) and attempting to close it with a double quote (`”`).
- Escape Characters: Using escape characters incorrectly can lead to unexpected behavior, causing the interpreter to misinterpret the string.
Examples of the Error
Here are some typical instances that trigger this error:
python
# Example 1: Missing Closing Quote
string_example = “This string is missing a closing quote
# Example 2: Improper Line Continuation
multi_line_string = “This is a string \
that is improperly continued
In the first example, the string is not terminated correctly. In the second example, the backslash at the end of the first line is intended to continue the string but is not sufficient without proper syntax.
How to Fix the Error
To resolve the `SyntaxError: EOL while scanning string literal`, follow these guidelines:
- Ensure Quotes Match: Always match the opening and closing quotes.
python
# Corrected Example
string_example = “This string is correctly closed”
- Properly Continue Multi-line Strings: Use triple quotes (`”””` or `”’`) for strings that span multiple lines or ensure that each line ends with a continuation character.
python
# Corrected Multi-line String
multi_line_string = “””This is a string
that is correctly continued”””
- Check for Escape Characters: If using backslashes, ensure they are correctly placed and not leading to unintentional line breaks.
Common Situations and Solutions
Situation | Cause | Solution |
---|---|---|
Missing quote | Unmatched quotes | Add the missing quote |
Improper line continuation | Backslash used incorrectly | Use triple quotes or ensure backslash is followed by a newline |
Mismatched quotes | Opening and closing quotes differ | Ensure quotes match |
Misuse of escape characters | Incorrectly placed backslash | Correct placement or use raw strings |
Best Practices to Avoid the Error
To prevent encountering this error in your code, adhere to the following best practices:
- Consistent Quoting: Stick to one style of quotes within a project (either single or double) to maintain clarity.
- Use IDE Features: Leverage the syntax highlighting and linting features of modern Integrated Development Environments (IDEs) to catch mismatched quotes early.
- Readability: Keep strings readable and manageable; if a string is lengthy, consider breaking it into smaller parts or using formatted strings.
- Testing: Regularly run your code in small increments to catch errors early, rather than waiting for larger blocks of code to execute.
By following these practices, you can minimize the occurrence of the `SyntaxError: EOL while scanning string literal` in your Python projects.
Understanding the SyntaxError: EOL While Scanning String Literal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘SyntaxError: EOL while scanning string literal’ typically indicates that a string in your code is not properly closed. This error often arises when a programmer forgets to include the closing quotation mark, leading to confusion in the interpreter about where the string ends.”
James Lin (Python Developer Advocate, CodeCraft Academy). “Encountering this error can be frustrating, especially for beginners. It serves as a reminder to always double-check your string syntax, particularly when dealing with multiline strings or complex concatenations that can easily lead to oversight.”
Maria Gonzalez (Lead Programming Instructor, Future Coders Institute). “Educating new developers about common syntax errors like this one is crucial. Understanding the structure of string literals and the importance of proper closure can significantly reduce debugging time and improve coding efficiency.”
Frequently Asked Questions (FAQs)
What does the error “SyntaxError: EOL while scanning string literal” mean?
This error indicates that a string in your code is not properly closed with a matching quotation mark before the end of the line (EOL). The interpreter reaches the end of the line while still expecting the closing quote.
How can I fix the “SyntaxError: EOL while scanning string literal” error?
To resolve this error, ensure that all string literals in your code are enclosed in matching quotation marks. Check for missing or mismatched quotes and correct them accordingly.
What are common causes of the “SyntaxError: EOL while scanning string literal” error?
Common causes include forgetting to close a string with a quotation mark, using the wrong type of quotation marks, or mistakenly placing a newline character within a string without proper continuation.
Can this error occur in multi-line strings?
Yes, this error can occur in multi-line strings if the string is not properly defined with triple quotes (”’ or “””). If using single or double quotes, ensure that the string is properly concatenated or split across lines.
Is this error specific to Python programming?
While the specific error message “SyntaxError: EOL while scanning string literal” is associated with Python, similar errors can occur in other programming languages when string literals are not correctly formatted.
What tools can help identify this error in my code?
Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, or online linters can help identify syntax errors, including “EOL while scanning string literal,” by providing real-time feedback and highlighting problematic lines.
The error message “SyntaxError: EOL while scanning string literal” is a common issue encountered in Python programming. This error indicates that the Python interpreter has reached the end of a line (EOL) while it was still expecting to find the closing quotation mark for a string. This typically occurs when a string is not properly terminated, leading to confusion in the code’s syntax. It serves as a reminder of the importance of carefully managing string literals in Python to avoid such pitfalls.
One of the primary causes of this error is the omission of the closing quotation mark in a string declaration. For instance, if a programmer starts a string with a single or double quote but forgets to include the corresponding closing quote, the interpreter will throw this error. Additionally, using multi-line strings without the appropriate triple quotes can also lead to this issue. Understanding these common scenarios is crucial for effective debugging and writing clean code.
To prevent encountering this error, developers should adopt best practices such as consistently checking for matching quotation marks and utilizing IDE features that highlight syntax errors. Moreover, employing comments and breaking down complex strings into simpler components can enhance clarity and reduce the likelihood of such errors. By being vigilant and methodical, programmers can significantly minimize the occurrence of the
Author Profile
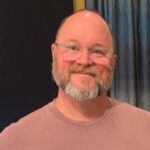
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?