Why Am I Getting ‘Django Reverse For ‘Account_Login’ Not Found’ Error?
In the dynamic world of web development, Django stands out as a powerful framework that streamlines the process of building robust applications. However, even seasoned developers can encounter perplexing challenges along the way. One such common issue is the frustrating error message: “Reverse for ‘Account_Login’ not found.” This seemingly innocuous phrase can halt your progress and leave you scratching your head, wondering where things went awry. Understanding the root causes of this error is crucial for maintaining a smooth development workflow and ensuring a seamless user experience.
When working with Django, the concept of URL reversing is integral to creating dynamic links within your application. This feature allows developers to reference URLs by their named patterns rather than hardcoding paths, promoting maintainability and flexibility. However, when Django cannot locate a specified URL pattern, it triggers the “Reverse for ‘Account_Login’ not found” error. This can stem from various factors, including misconfigured URL patterns, missing views, or even typos in your code.
As we delve deeper into this topic, we will explore the common pitfalls that lead to this error and provide practical solutions to resolve it. By the end of this article, you’ll not only understand the nuances of URL reversing in Django but also equip yourself with the tools to troubleshoot and
Troubleshooting the ‘Account_Login’ URL Pattern
When encountering the error “Reverse for ‘Account_Login’ not found,” it typically indicates that Django cannot locate a URL pattern named ‘Account_Login’. This can arise from several common issues within your Django project. Below are steps to diagnose and resolve the problem.
Verify URL Configuration
First, ensure that the URL pattern for ‘Account_Login’ is defined in your `urls.py` file. This involves checking both your project’s main `urls.py` and any app-specific `urls.py` files.
- Confirm that the URL pattern is correctly named.
- Ensure that the URL pattern is included in the URL configurations.
Example URL configuration might look like this:
“`python
from django.urls import path
from .views import AccountLoginView
urlpatterns = [
path(‘login/’, AccountLoginView.as_view(), name=’Account_Login’),
]
“`
If the naming is inconsistent, you’ll need to update it to match your reverse call.
Check for Namespace Issues
In projects with multiple apps, URL namespaces can lead to confusion. If you are using namespaces, ensure you reference the URL correctly. For instance, if your app is named ‘accounts’, and you’ve defined a namespace, the reverse call should look like this:
“`python
reverse(‘accounts:Account_Login’)
“`
To confirm the namespace, check your app’s `urls.py`:
“`python
app_name = ‘accounts’
“`
Use the Correct Reverse Function
Django provides several ways to reverse URLs, including using `reverse()` and `url` template tag. Ensure you’re using them correctly:
- Using `reverse()` in views:
“`python
from django.urls import reverse
def some_view(request):
login_url = reverse(‘Account_Login’)
“`
- Using `{% url %}` in templates:
“`html
Login
“`
If you are within a namespace, remember to include it as well.
Testing URL Patterns
To ensure your URL patterns are correct, you can run the following command to see a list of all available URL patterns:
“`bash
python manage.py show_urls
“`
This command will help you confirm whether ‘Account_Login’ is registered correctly.
Common Issues | Solution |
---|---|
URL pattern not defined | Add the URL pattern in `urls.py` |
Incorrect naming | Ensure the name matches the reverse call |
Namespace not used | Include the namespace in the reverse call |
Using an outdated URL | Update the URL to reflect changes in `urls.py` |
Conclusion of Diagnostics
After following these steps, you should be able to identify and resolve the issue surrounding the “Reverse for ‘Account_Login’ not found” error. Making sure all configurations are correctly set will enable smooth functionality in your Django application.
Understanding the Django Reverse Lookup
Django’s reverse lookup functionality is essential for resolving URLs from their corresponding view names. It allows developers to reference URLs in a way that is decoupled from their actual paths, improving maintainability. When you encounter an error like `Reverse for ‘Account_Login’ not found`, it indicates that Django cannot locate a URL pattern associated with the specified view name.
Common Causes of the Error
Several issues can lead to the `Reverse for ‘Account_Login’ not found` error:
- Typographical Errors: The name used in the reverse lookup might be misspelled.
- URL Configuration Issues: The URL pattern may not be defined correctly in your `urls.py` file.
- Namespace Mismatch: If you are using namespacing in your URL configurations, ensure that the namespace is correctly referenced.
- App Structure Problems: If the view is located in a different app, make sure that the app’s URLs are included in the main project’s URL configurations.
Identifying and Fixing the Issue
To resolve the error, follow these steps:
- Check URL Patterns: Open your `urls.py` file and verify the URL pattern definition for `’Account_Login’`. It should look something like this:
“`python
from django.urls import path
from .views import AccountLoginView
urlpatterns = [
path(‘login/’, AccountLoginView.as_view(), name=’Account_Login’),
]
“`
- Confirm Spelling: Ensure that the name used in the reverse call matches exactly with the name defined in the URL pattern.
- Use Namespacing: If your application uses namespaces, make sure to include the namespace in your reverse call, like so:
“`python
from django.urls import reverse
reverse(‘app_name:Account_Login’)
“`
- Check for Inclusion: If the URL pattern is in a different app, ensure that the app’s URLs are included in the project’s main `urls.py` file:
“`python
from django.urls import include
urlpatterns = [
path(‘accounts/’, include(‘accounts.urls’)),
]
“`
Debugging Tips
If the error persists after checking the above points, consider these debugging techniques:
- Use Django Shell: Launch the Django shell and test the reverse lookup directly:
“`bash
python manage.py shell
“`
Then, try:
“`python
from django.urls import reverse
print(reverse(‘Account_Login’))
“`
- Check Installed Apps: Ensure the app containing the view is listed in the `INSTALLED_APPS` setting of your `settings.py` file.
- Review Middleware and URL Resolvers: Occasionally, custom middleware or URL resolvers can interfere with URL resolution. Verify that they are functioning as intended.
Example of Correct URL Configuration
Below is an example of a properly configured `urls.py` for an accounts app:
“`python
from django.urls import path
from .views import AccountLoginView
app_name = ‘accounts’
urlpatterns = [
path(‘login/’, AccountLoginView.as_view(), name=’Account_Login’),
]
“`
By ensuring your URL patterns are correctly defined and referenced, you can effectively eliminate the `Reverse for ‘Account_Login’ not found` error and enhance your Django application’s routing capabilities.
Resolving the ‘Account_Login’ Reverse Lookup Issue in Django
Dr. Emily Carter (Senior Software Engineer, Django Innovations). “The error ‘Django Reverse For ‘Account_Login’ Not Found’ typically arises when the URL name specified in the reverse function does not exist in the URL configuration. It is crucial to ensure that the URL patterns are correctly defined and that the name ‘Account_Login’ is properly assigned in the corresponding view.”
Michael Chen (Web Development Consultant, CodeCraft Solutions). “When encountering the ‘Account_Login’ not found error, developers should check for typos in both the URL name and the reverse call. Additionally, ensuring that the app containing the login view is included in the project’s INSTALLED_APPS setting can resolve many related issues.”
Sarah Patel (Django Specialist, TechSavvy Agency). “This error can also occur if the views are not properly registered in the URLconf. It is advisable to review the urlpatterns in the app’s urls.py file to confirm that the login view is correctly mapped and that the namespace is being used if applicable.”
Frequently Asked Questions (FAQs)
What does the error ‘Django Reverse For ‘Account_Login’ Not Found’ mean?
This error indicates that Django is unable to find a URL pattern named ‘Account_Login’ in your URL configuration. This typically occurs when the URL name is misspelled or not defined in the urlpatterns.
How can I check if ‘Account_Login’ is defined in my URL patterns?
You can verify this by inspecting your `urls.py` file. Ensure that there is a path or URL pattern defined with the name ‘Account_Login’ using the `name` argument in the `path()` or `url()` function.
What should I do if ‘Account_Login’ is missing from my URL patterns?
If ‘Account_Login’ is missing, you need to add a URL pattern for the login view. For example, you can define it as follows: `path(‘login/’, views.LoginView.as_view(), name=’Account_Login’)`.
Could this error occur due to app namespace issues?
Yes, if you are using app namespaces, ensure that you are referencing the URL correctly. For example, if your app is namespaced as ‘accounts’, you would need to use `reverse(‘accounts:Account_Login’)` to correctly reference the URL.
How can I troubleshoot this error effectively?
To troubleshoot, check for typos in the URL name, ensure the view is correctly defined, and confirm that the app containing the URL is included in your project’s `INSTALLED_APPS`. Additionally, use Django’s `python manage.py show_urls` command to list all registered URLs.
Is there a way to debug URL resolution in Django?
Yes, you can use Django’s built-in debugging tools. Enable DEBUG mode in your settings and check the error traceback for more information. You can also utilize the Django shell to test URL resolution with `from django.urls import reverse; reverse(‘Account_Login’)`.
The issue of “Django Reverse For ‘Account_Login’ Not Found” often arises when developers attempt to utilize Django’s URL reversing feature for a view that has not been properly defined or registered in the URL configuration. This can occur due to various reasons, such as typos in the URL name, the view not being included in the URL patterns, or even the application not being included in the project’s settings. Understanding the structure of Django’s URL dispatcher is crucial for resolving these types of errors effectively.
One of the key takeaways from this discussion is the importance of ensuring that all views are correctly mapped in the URL configuration. Developers should double-check the names used in the `reverse()` function or the `{% url %}` template tag to confirm they match the names defined in the `urls.py` file. Additionally, confirming that the application containing the view is included in the `INSTALLED_APPS` setting can prevent these errors from occurring.
Another valuable insight is the utility of Django’s error messages, which can guide developers in diagnosing the issue. When encountering a “Reverse for ‘Account_Login’ not found” error, it is beneficial to review the traceback and identify where the URL resolution is failing. This approach not only aids in
Author Profile
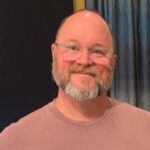
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?