How to Resolve the ‘Cannot Find Module ‘Webpack/Lib/Ruleset’ Error in Your Project?
In the ever-evolving landscape of web development, tools and frameworks are constantly being updated, leading to both exciting innovations and potential pitfalls. One such pitfall that many developers encounter is the cryptic error message: “Cannot Find Module ‘Webpack/Lib/Ruleset’.” This seemingly innocuous line can send even seasoned developers into a spiral of confusion and frustration. Understanding the root causes of this error is essential for anyone looking to streamline their development process and ensure a smooth workflow.
As developers increasingly rely on Webpack to bundle their JavaScript applications, the complexities of its configuration can sometimes lead to unexpected challenges. The error message often signifies issues related to module resolution, version mismatches, or misconfigurations within the Webpack setup. This can be particularly daunting for those who are new to the framework or who have recently upgraded their dependencies. By delving into the intricacies of this error, developers can not only troubleshoot effectively but also gain a deeper understanding of Webpack’s architecture and functionality.
In this article, we will explore the common causes of the “Cannot Find Module ‘Webpack/Lib/Ruleset'” error, offering insights and solutions to help you overcome this hurdle. Whether you’re a novice just starting out or an experienced developer looking to refine your skills,
Understanding the Module Resolution in Webpack
The error message “Cannot Find Module ‘Webpack/Lib/Ruleset'” often indicates that the Webpack configuration is incorrectly set up or that there are issues with module resolution. Webpack’s module resolution process is crucial for loading and bundling JavaScript files and their dependencies correctly.
When working with Webpack, it’s essential to ensure that all modules are correctly installed and paths are correctly referenced. Here are a few common causes and solutions for this error:
- Incorrect Module Installation: Ensure that all required dependencies are installed. You can check your `package.json` file for any missing packages.
- Case Sensitivity: File paths in imports are case-sensitive. Verify that the path used in your import statement matches the actual file structure.
- Webpack Configuration: Review the Webpack configuration file (`webpack.config.js`). Ensure that the rules and paths specified are accurate.
- Node Modules Corruption: Sometimes, the `node_modules` directory may become corrupted. Deleting it and running `npm install` or `yarn install` again can resolve this.
Common Fixes for Module Resolution Issues
When encountering module resolution issues in Webpack, consider the following fixes:
- Reinstalling Node Modules:
- Run the following commands:
“`bash
rm -rf node_modules
npm install
“`
- Or, if you are using Yarn:
“`bash
rm -rf node_modules
yarn install
“`
- Check Webpack Version Compatibility: Ensure that the version of Webpack you are using is compatible with the plugins and loaders specified in your configuration. Mismatched versions can lead to unexpected errors.
- Using Absolute Paths: Instead of relative paths, consider using absolute paths in your imports to reduce confusion. This can be achieved by configuring the `resolve.modules` in your Webpack config.
- Debugging with Verbose Logging: Enable verbose logging in Webpack to get more insights into the module resolution process. Add the following to your Webpack config:
“`javascript
stats: {
colors: true,
reasons: true,
verbose: true,
}
“`
Module Resolution Strategies in Webpack
Webpack offers various strategies for resolving modules, which can be configured in the `resolve` section of your `webpack.config.js`. Below are some common strategies:
- Extensions: Specify which file extensions Webpack should resolve automatically.
- Alias: Create aliases for directories to simplify imports.
- Modules: Define which directories Webpack should search when resolving modules.
Here’s how you might configure these options in `webpack.config.js`:
“`javascript
resolve: {
extensions: [‘.js’, ‘.jsx’, ‘.ts’, ‘.tsx’],
alias: {
components: path.resolve(__dirname, ‘src/components/’),
},
modules: [path.resolve(__dirname, ‘src’), ‘node_modules’]
}
“`
Resolution Option | Description |
---|---|
Extensions | File types Webpack will resolve automatically. |
Alias | Shortcuts for importing modules, improving readability. |
Modules | Directories to search for modules, prioritizing specified paths. |
By ensuring that your Webpack configuration is correctly set up and that all modules are properly installed, you can effectively troubleshoot and resolve the “Cannot Find Module ‘Webpack/Lib/Ruleset'” error.
Common Causes of Module Not Found Errors
Module not found errors, such as `Cannot Find Module ‘Webpack/Lib/Ruleset’`, can arise from various issues during the configuration or runtime of a Webpack project. Understanding these causes can help in troubleshooting effectively.
- Incorrect Path Specification: The specified path for the module may be incorrect or improperly referenced. This can occur due to:
- Typos in the module name or path.
- Incorrect casing, as file paths can be case-sensitive in some environments.
- Missing Dependencies: The required module may not be installed in your project. This can happen if:
- The module was never installed.
- The installation failed or was interrupted.
- Version Mismatch: If your project relies on a specific version of Webpack or associated plugins, an update might lead to discrepancies. Possible issues include:
- Deprecated modules or APIs.
- Incompatible versions of Webpack and its loaders or plugins.
- Node Modules Corruption: Sometimes, the `node_modules` directory may become corrupted. This can lead to various errors, including module resolution failures.
- Configuration Errors: Misconfigurations in the Webpack configuration file (`webpack.config.js`) can also lead to module not found errors. Common mistakes include:
- Incorrectly set `resolve` options.
- Missing or misconfigured rules in the module section.
Troubleshooting Steps
To resolve the `Cannot Find Module ‘Webpack/Lib/Ruleset’` error, consider the following troubleshooting steps:
- Check Module Installation:
- Run `npm install webpack` or `yarn add webpack` to ensure that Webpack is installed correctly.
- Verify that the module exists in `node_modules`.
- Verify Path and Case Sensitivity:
- Double-check the path you are using in your import statement.
- Ensure the casing matches the actual file and directory names.
- Reinstall Node Modules:
- Delete the `node_modules` directory and run `npm install` or `yarn` to reinstall all packages. This can resolve issues caused by corrupted installations.
- Check Webpack Configuration:
- Open `webpack.config.js` and review the `resolve` settings. Ensure that they are correctly configured to locate your modules.
- Look for any rules that might inadvertently exclude the module.
- Consult Documentation:
- Review the official Webpack documentation for any breaking changes or migration guides if you have recently updated Webpack.
Example Configuration Snippet
Here is an example of a basic Webpack configuration that ensures proper module resolution:
“`javascript
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
resolve: {
extensions: [‘.js’, ‘.jsx’, ‘.json’],
modules: [
path.resolve(__dirname, ‘src’),
‘node_modules’
],
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: ‘babel-loader’,
},
},
],
},
};
“`
This configuration ensures that Webpack can find and correctly resolve the necessary modules. Adjust the paths and loaders as per your project requirements.
Additional Considerations
When addressing module resolution issues, keep the following in mind:
- Environment Compatibility: Ensure that the development environment matches the required setup for Webpack.
- Cross-Platform Issues: If the project is being shared across different operating systems, ensure paths are universally compatible.
- Version Control: Regularly check your `package.json` and `package-lock.json` or `yarn.lock` files for consistency in package versions.
By following these strategies, you can effectively diagnose and resolve the module not found error in your Webpack projects.
Resolving Module Import Issues in Webpack
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The error message ‘Cannot Find Module ‘Webpack/Lib/Ruleset” typically indicates that the specified module is either not installed or incorrectly referenced in your configuration. It is essential to ensure that your Webpack version is compatible with the modules you are trying to use.”
Michael Chen (Lead Developer, Frontend Innovations). “In many cases, this error arises from a misconfiguration in the Webpack setup. Double-check your ‘webpack.config.js’ file for any typos or incorrect paths, and ensure that all required dependencies are properly installed in your project.”
Sarah Thompson (DevOps Specialist, Agile Tech Solutions). “If you encounter the ‘Cannot Find Module’ error, consider clearing your node_modules directory and reinstalling your packages. This often resolves issues related to module resolution and ensures that all dependencies are correctly linked.”
Frequently Asked Questions (FAQs)
What does the error “Cannot Find Module ‘Webpack/Lib/Ruleset'” indicate?
This error indicates that the Webpack module specified cannot be located within the project. It typically arises due to incorrect module paths or missing dependencies.
How can I resolve the “Cannot Find Module ‘Webpack/Lib/Ruleset'” error?
To resolve this error, ensure that the module is correctly installed in your project. You can reinstall Webpack or check your configuration files for any incorrect paths.
Is ‘Webpack/Lib/Ruleset’ a deprecated module?
Yes, ‘Webpack/Lib/Ruleset’ has been deprecated in favor of newer configurations and modules in Webpack. It is advisable to consult the latest Webpack documentation for updated practices.
What are common reasons for module not found errors in Webpack?
Common reasons include incorrect file paths, missing dependencies, outdated Webpack versions, or misconfigured Webpack settings that do not point to the correct module locations.
How can I check if the required module is installed?
You can check if the required module is installed by reviewing your project’s `package.json` file or by running the command `npm list webpack` in your project directory.
What steps should I take if reinstalling Webpack does not fix the issue?
If reinstalling Webpack does not resolve the issue, consider clearing the npm cache, checking for conflicting packages, and reviewing your Webpack configuration for any misconfigurations.
The error message “Cannot Find Module ‘Webpack/Lib/Ruleset'” typically indicates a problem with the Webpack configuration or the installation of Webpack itself. This issue often arises when the module is either missing from the project or not properly referenced in the configuration files. Developers encountering this error should first verify that Webpack is correctly installed in their project and that the specific module path is accurate within the configuration settings.
Additionally, it is crucial to ensure that the version of Webpack being used is compatible with the other dependencies in the project. Incompatibilities can lead to missing modules or altered paths that may not align with the current project structure. Developers should also check for any updates or changes in the Webpack documentation that might affect how modules are imported or utilized, as Webpack evolves frequently with new releases.
Lastly, if the problem persists, examining the project’s node_modules directory can provide insights into whether the required module exists. Running commands such as `npm install` or `yarn install` may resolve issues related to missing dependencies. Engaging with community forums or consulting the official Webpack GitHub repository can also be beneficial for troubleshooting and finding solutions related to this specific error.
Author Profile
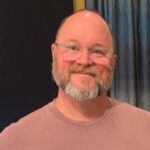
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?