Does Python Have Interfaces? Exploring the Concept and Its Alternatives
Does Python Have Interfaces?
In the ever-evolving landscape of programming languages, Python stands out for its simplicity and versatility. As developers seek to create robust and maintainable code, the concept of interfaces often comes into play. But does Python, known for its dynamic typing and flexible structure, truly embrace the idea of interfaces in the same way that statically typed languages like Java or C# do? This question opens the door to a deeper exploration of how Python manages abstraction, enforces contracts, and promotes code reusability.
At first glance, Python may not have formal interfaces as seen in other languages. However, it offers powerful alternatives that achieve similar goals. Through the use of abstract base classes (ABCs) and duck typing, Python allows developers to define expected behaviors without rigid constraints. This flexibility encourages creativity and innovation, enabling programmers to implement their own versions of interfaces while leveraging Python’s dynamic nature.
As we delve deeper into this topic, we will explore how Python’s approach to interfaces can enhance code organization and collaboration among developers. We will also examine practical examples and best practices that illustrate how to effectively implement interface-like structures in Python, ensuring your code remains clean, efficient, and adaptable in an ever-changing programming environment.
Understanding Interfaces in Python
Python does not have a formal interface concept like some other programming languages, such as Java or C#. However, it supports a similar mechanism through the use of abstract base classes (ABCs) and duck typing. This allows developers to create a structure that enforces specific methods to be implemented in derived classes, effectively functioning as an interface.
Abstract Base Classes
Abstract base classes are part of the `abc` module in Python, which enables the definition of abstract methods that must be implemented by any subclass. An abstract class can contain both abstract methods (methods that have no implementation) and concrete methods (methods that do have an implementation).
To define an abstract class, one needs to:
- Import the `ABC` class and the `abstractmethod` decorator from the `abc` module.
- Create a class that inherits from `ABC`.
- Use the `@abstractmethod` decorator to declare abstract methods.
Here’s a simple example:
python
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
return “Bark”
class Cat(Animal):
def sound(self):
return “Meow”
In this example, `Animal` is an abstract base class with an abstract method `sound`. Both `Dog` and `Cat` classes must implement the `sound` method, or they will raise a `TypeError` when instantiated.
Duck Typing
Duck typing is a programming style associated with dynamic languages like Python, where the type or class of an object is less important than the methods it defines or the way it behaves. The principle behind duck typing is that if an object behaves like a certain type, it can be treated as that type.
For example:
python
class Duck:
def quack(self):
return “Quack!”
class Person:
def quack(self):
return “I’m quacking like a duck!”
def make_it_quack(thing):
print(thing.quack())
duck = Duck()
person = Person()
make_it_quack(duck) # Outputs: Quack!
make_it_quack(person) # Outputs: I’m quacking like a duck!
In this case, both `Duck` and `Person` have a method `quack`, and the function `make_it_quack` can accept either type without needing to check their types explicitly.
Benefits of Using Interfaces
Using abstract base classes and duck typing offers several advantages:
- Flexibility: Developers can create classes that adhere to a specific interface without being bound to a specific class hierarchy.
- Code Reusability: Abstract base classes promote code reuse by allowing shared implementation details while enforcing a contract on the subclasses.
- Improved Readability: Clearly defined interfaces enhance the understanding of the system’s architecture and the relationships between components.
Comparison of Abstract Base Classes and Duck Typing
Feature | Abstract Base Classes | Duck Typing |
---|---|---|
Type Checking | Static type checking at class level | Dynamic type checking based on method presence |
Implementation Enforcement | Requires implementation of abstract methods | No enforcement; relies on convention |
Use Case | When strict interfaces are needed | When flexibility is prioritized |
In summary, while Python does not feature interfaces in the traditional sense, it provides powerful alternatives that encourage flexible and maintainable code.
Understanding Interfaces in Python
Python does not have built-in interface constructs like some other programming languages, such as Java or C#. However, it provides several ways to implement interface-like behavior, primarily through abstract base classes (ABCs) and the use of duck typing.
Abstract Base Classes
Abstract Base Classes are part of the `abc` module in Python. They allow developers to define abstract methods that must be implemented by subclasses. This is how you can enforce a certain structure in your classes, mimicking interface behavior.
- Defining an ABC:
- Import the `ABC` class and `abstractmethod` decorator from the `abc` module.
- Create a class that inherits from `ABC`.
- Use the `@abstractmethod` decorator to define methods that must be overridden.
python
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
return “Bark”
class Cat(Animal):
def sound(self):
return “Meow”
In this example, `Animal` serves as an interface with an abstract method `sound`. Both `Dog` and `Cat` are required to implement this method.
Duck Typing
Python employs a philosophy known as duck typing, which allows for flexibility in type usage. Instead of enforcing strict type checks, Python checks for the presence of methods and properties at runtime.
- Principle of Duck Typing:
- “If it looks like a duck and quacks like a duck, it must be a duck.”
- Classes do not need to explicitly declare that they implement an interface, as long as they provide the required methods.
python
class Bird:
def sound(self):
return “Chirp”
def make_sound(animal):
print(animal.sound())
dog = Dog()
bird = Bird()
make_sound(dog) # Output: Bark
make_sound(bird) # Output: Chirp
In the example above, the `make_sound` function accepts any object that has a `sound` method, demonstrating the flexibility provided by duck typing.
Protocols in Python
With the introduction of PEP 484, Python supports the concept of “protocols,” which are a more formal way to define interface-like structures using type hints. This is particularly useful in type checking with tools like `mypy`.
– **Defining a Protocol**:
- Use the `Protocol` class from the `typing` module.
- Specify the required methods.
python
from typing import Protocol
class Soundable(Protocol):
def sound(self) -> str:
…
def make_sound(animal: Soundable) -> None:
print(animal.sound())
Any class implementing the `sound` method will satisfy the `Soundable` protocol, enabling type checking without the need for inheritance.
Comparison of Approaches
Here is a brief comparison of the different methods for implementing interface-like behavior in Python:
Feature | Abstract Base Classes | Duck Typing | Protocols |
---|---|---|---|
Requires Inheritance | Yes | No | No |
Runtime Type Checking | Yes | No | Yes |
Static Type Checking | No | No | Yes |
Use of Decorators | Yes | No | No |
Each method has its own advantages and use cases, allowing developers to choose the most appropriate mechanism based on their needs.
Expert Insights on Python’s Interface Capabilities
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While Python does not have interfaces in the same way that languages like Java do, it utilizes a concept called ‘duck typing.’ This allows for a flexible approach to object-oriented programming, where the suitability of an object is determined by the presence of certain methods and properties, rather than the object’s type itself.”
Michael Chen (Lead Developer, Open Source Projects). “In Python, abstract base classes (ABCs) can be used to create a form of interface. By using the `abc` module, developers can define abstract methods that must be implemented in derived classes, thereby enforcing a contract similar to traditional interfaces found in statically typed languages.”
Lisa Patel (Python Instructor, Code Academy). “The lack of formal interfaces in Python encourages a more dynamic and flexible coding style. This flexibility can lead to more innovative solutions, as developers are not constrained by rigid type systems. However, it also places the responsibility on developers to ensure that their classes adhere to expected behaviors.”
Frequently Asked Questions (FAQs)
Does Python have interfaces?
Python does not have a formal interface construct like some other programming languages. However, it supports interfaces through abstract base classes (ABCs) defined in the `abc` module, allowing you to create methods that must be implemented in derived classes.
How do you create an interface in Python?
You can create an interface in Python by defining an abstract base class using the `ABC` class from the `abc` module. You then define abstract methods using the `@abstractmethod` decorator, which must be implemented by any subclass.
Can you implement multiple interfaces in Python?
Yes, Python supports multiple inheritance, allowing a class to implement multiple interfaces by inheriting from multiple abstract base classes. This enables the class to provide implementations for all required methods from each interface.
What is the purpose of using interfaces in Python?
Interfaces in Python promote a design principle known as “programming to an interface, not an implementation.” This encourages loose coupling and enhances code maintainability by defining clear contracts for what methods a class must implement.
Are there any built-in interfaces in Python?
Python’s standard library includes several abstract base classes that serve as interfaces, such as `Iterable`, `Iterator`, and `Sized`. These can be used to enforce specific behaviors in user-defined classes.
How do interfaces improve code quality in Python?
Interfaces improve code quality by ensuring that classes adhere to a defined structure, facilitating easier testing, and promoting code reusability. They also enhance collaboration among developers by providing clear expectations for class behavior.
In Python, the concept of interfaces is not explicitly defined as it is in some other programming languages like Java or C#. Instead, Python employs a more flexible approach through the use of abstract base classes (ABCs) and duck typing. ABCs allow developers to define a set of methods that must be created within any subclass, effectively creating a contract that enforces certain behaviors without rigidly dictating how those behaviors should be implemented.
Moreover, Python’s dynamic typing system embraces the principle of duck typing, which means that the suitability of an object is determined by the presence of certain methods and properties rather than the object’s type itself. This flexibility allows for a more fluid implementation of interfaces, enabling developers to focus on what an object can do rather than what it is. As a result, Python promotes a more intuitive and less restrictive programming style that aligns well with its philosophy of simplicity and readability.
Key takeaways from this discussion include the understanding that while Python does not have formal interfaces, it provides powerful tools such as abstract base classes and duck typing to achieve similar outcomes. Developers can leverage these features to create robust, maintainable code that adheres to the principles of polymorphism and encapsulation. Ultimately, Python’s approach to interfaces reflects its
Author Profile
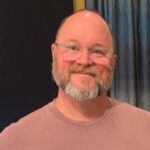
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?