Why Does the Error ‘Object Literal May Only Specify Known Properties’ Keep Popping Up in My Code?
In the world of programming, particularly in TypeScript, developers often encounter a common yet perplexing error: “Object literal may only specify known properties.” This seemingly straightforward message can lead to confusion and frustration, especially for those who are new to the language or transitioning from JavaScript. Understanding this error is crucial for writing robust, type-safe code that adheres to the principles of TypeScript. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and best practices for resolution. By the end, you’ll be equipped with the knowledge to navigate this common pitfall with confidence.
The error “Object literal may only specify known properties” arises when an object literal is defined with properties that are not recognized by the type it is being assigned to. This feature of TypeScript is designed to enhance type safety, ensuring that developers adhere to defined structures and avoid unintentional mistakes. However, while this strictness can be beneficial, it can also lead to frustration when developers are faced with unexpected type-checking issues. Understanding the underlying principles of TypeScript’s type system is essential for effectively managing these errors.
As we explore this topic, we will examine the scenarios in which this error typically occurs, the rationale behind TypeScript’s property
Understanding the Error
The error message “Object literal may only specify known properties” typically occurs in TypeScript when an object literal is assigned to a variable or function parameter that has a specific type, and the object literal includes properties that are not defined in that type. This mechanism is part of TypeScript’s structural typing system, which ensures that objects conform to expected shapes.
When you define an interface or type in TypeScript, you are essentially creating a contract that specifies which properties an object can have. If an object literal contains additional properties not defined in the interface or type, TypeScript raises an error. This helps catch potential bugs early in the development process by enforcing type safety.
Common Causes of the Error
Several scenarios can lead to this error:
- Extra Properties: Including properties in an object literal that are not part of the defined type.
- Mismatched Types: Using properties that are defined but with incorrect types.
- Incorrect Type Definitions: Defining the type or interface incorrectly, leading to confusion about what properties are actually expected.
Examples of the Error
Consider the following example where the error might occur:
“`typescript
interface Person {
name: string;
age: number;
}
const individual: Person = {
name: “Alice”,
age: 30,
hobby: “reading” // Error: Object literal may only specify known properties
};
“`
In this example, the `hobby` property is not defined in the `Person` interface, resulting in the error.
Fixing the Error
To resolve this error, you have several options:
- Remove Extra Properties: Ensure the object literal only contains properties defined in the interface.
- Update the Type Definition: If the additional properties are valid and should be part of the type, update the interface or type definition accordingly.
- Use Type Assertion: When you’re confident about the structure, you can use type assertions, but this should be done cautiously.
Practical Solutions
Here are practical solutions to fix the error:
Action | Code Example |
---|---|
Remove Extra Properties |
const individual: Person = { name: "Alice", age: 30 }; |
Update the Interface |
interface Person { name: string; age: number; hobby: string; // Now valid } const individual: Person = { name: "Alice", age: 30, hobby: "reading" }; |
Use Type Assertion |
const individual = { name: "Alice", age: 30, hobby: "reading" } as Person; // Assumes the structure is valid |
By applying these solutions, you can effectively eliminate the “Object literal may only specify known properties” error and maintain strong type safety in your TypeScript code.
Understanding the Error Message
The error message “Object literal may only specify known properties” occurs in TypeScript when you attempt to create an object with properties that are not defined in its type. This can lead to runtime issues if the object is not properly structured. It is essential to ensure that the object conforms to the specified type.
Common scenarios where this error arises include:
- Incorrect Property Names: Misspellings or incorrect casing.
- Excess Properties: Adding extra properties that are not defined in the interface or type.
- Type Mismatch: Assigning values of a type that does not match the expected type for a property.
Examples of the Error
To illustrate how this error manifests, consider the following examples:
“`typescript
interface User {
name: string;
age: number;
}
// Error: Property ‘gender’ does not exist in type ‘User’.
const user1: User = {
name: “John”,
age: 30,
gender: “male” // Extra property
};
// Error: Type ‘string’ is not assignable to type ‘number’.
const user2: User = {
name: “Jane”,
age: “twenty-five” // Type mismatch
};
// Error: Property ‘name’ is missing.
const user3: User = {
age: 25 // Missing property
};
“`
How to Fix the Error
To resolve the error, follow these best practices:
- Ensure All Properties Are Defined: Verify that all properties in your object match those declared in the type or interface.
- Use Type Assertion: If you are certain that your object is correct and want to bypass the error, you can use type assertion, although this should be done cautiously.
Example of using type assertion:
“`typescript
const user4 = {
name: “Alice”,
age: 28,
gender: “female”
} as User; // Type assertion
“`
- Update Interface Definitions: If you need to include additional properties, modify the interface accordingly.
“`typescript
interface User {
name: string;
age: number;
gender?: string; // Making gender optional
}
“`
Best Practices for TypeScript Object Literals
To avoid the “Object literal may only specify known properties” error, adhere to the following best practices:
- Consistent Naming Conventions: Maintain uniformity in property names to prevent typographical errors.
- Use Type Inference: Leverage TypeScript’s type inference to reduce explicit type declarations where appropriate.
- Utilize TypeScript’s Strict Mode: Enable strict mode in your TypeScript configuration to catch errors early in the development process.
Best Practice | Description |
---|---|
Consistent Naming | Use the same naming conventions throughout. |
Type Inference | Allow TypeScript to infer types automatically. |
Strict Mode | Enable strict checks for better error handling. |
By following these guidelines, you can effectively manage object literals in TypeScript and minimize the risk of encountering this error message.
Understanding the Implications of Object Literal May Only Specify Known Properties
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘Object Literal May Only Specify Known Properties’ serves as a crucial reminder for developers to adhere to defined interfaces. It reinforces the importance of type safety in programming, ensuring that objects conform to expected structures, which ultimately leads to more maintainable and predictable code.”
Michael Chen (Lead Developer, Code Quality Solutions). “This restriction in TypeScript is not just a limitation; it is a powerful feature that encourages developers to think critically about the shape of their data. By enforcing known properties, we can avoid runtime errors and enhance the robustness of our applications.”
Sarah Patel (Technical Architect, Future Tech Labs). “Understanding the implications of the ‘Object Literal May Only Specify Known Properties’ error is essential for building scalable applications. It highlights the necessity of defining clear interfaces and types, which can significantly reduce bugs and improve collaboration among team members.”
Frequently Asked Questions (FAQs)
What does the error “Object Literal May Only Specify Known Properties” mean?
This error indicates that an object literal is being defined with properties that are not recognized by the type definition of the object. This typically occurs in TypeScript when the object is expected to conform to a specific interface or type.
How can I resolve the “Object Literal May Only Specify Known Properties” error?
To resolve this error, ensure that all properties defined in the object literal match the properties specified in the corresponding interface or type. Remove any extraneous properties that are not defined in the type.
What are some common scenarios that trigger this error?
Common scenarios include passing an object literal to a function that expects a specific type, or when defining an object that is supposed to implement an interface but includes additional properties.
Can I use index signatures to bypass this error?
Yes, you can use index signatures in your interface or type definition to allow for additional properties. However, this approach should be used judiciously to maintain type safety and clarity in your code.
Is this error specific to TypeScript?
Yes, this error is specific to TypeScript, which enforces strict type checking. JavaScript does not have this restriction, as it is more flexible with object literals.
What are the implications of ignoring this error?
Ignoring this error can lead to runtime issues, as the object may not behave as expected if it contains properties that are not accounted for in the type definition. This can result in bugs that are difficult to trace and fix.
The error message “Object Literal May Only Specify Known Properties” typically arises in TypeScript when an object literal is being used to initialize a variable or pass arguments to a function, and it contains properties that are not defined in the type of that variable or function. This serves as a safeguard to ensure that only valid and expected properties are included, thereby promoting type safety and reducing runtime errors. Understanding this error is crucial for developers working with TypeScript, as it emphasizes the importance of adhering to defined types and interfaces.
One of the key takeaways from this discussion is the necessity of defining interfaces or types that accurately represent the structure of objects used in the code. By doing so, developers can avoid common pitfalls associated with object literals, such as typos or the inclusion of extraneous properties. This practice not only enhances code quality but also improves maintainability, as it provides clear documentation of expected object shapes.
Additionally, developers should familiarize themselves with TypeScript’s strict property checking features. Utilizing tools like TypeScript’s compiler options can help identify potential issues at compile time rather than at runtime. This proactive approach allows for a more robust development process, ultimately leading to more reliable applications and fewer debugging sessions.
Author Profile
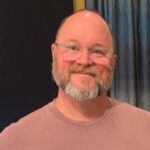
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?