How Can You Effectively Stop an Infinite Loop in Python?
Infinite loops can be a programmer’s worst nightmare. Imagine writing a piece of code that you believe is flawless, only to find that it gets stuck in a never-ending cycle, consuming resources and potentially crashing your program. Whether you’re a seasoned developer or a novice just starting your coding journey, encountering an infinite loop is a common pitfall that can lead to frustration and wasted time. In this article, we’ll explore the causes of infinite loops in Python, the signs to watch for, and most importantly, effective strategies to stop them in their tracks.
Understanding how to manage infinite loops is crucial for maintaining the efficiency and reliability of your code. Often, these loops arise from logical errors or oversight in the loop’s exit conditions. By recognizing the symptoms of an infinite loop early on, you can save yourself from the headache of debugging and optimize your code for better performance.
In the following sections, we will delve into practical techniques to halt these loops, including using debugging tools and implementing proper loop constructs. Whether you need to terminate a runaway process or prevent it from occurring in the first place, arming yourself with the right knowledge will empower you to tackle infinite loops confidently and effectively. Let’s dive deeper into this essential aspect of Python programming and ensure your coding experience is as smooth
Identifying Infinite Loops
To effectively stop an infinite loop in Python, it is crucial first to identify the loop. An infinite loop occurs when the terminating condition is never met. Common signs of an infinite loop include:
- The program does not produce any output or response.
- The CPU usage remains at 100% for the application.
- The application becomes unresponsive to user inputs.
To diagnose the issue, inspect your loop structure. A typical loop can be structured as follows:
“`python
while condition:
code block
“`
Ensure that the `condition` is correctly defined and that it will eventually evaluate to “.
Using Break Statements
One effective method to terminate an infinite loop is by using a `break` statement. This statement allows you to exit the loop when a certain condition is met. Here’s an example:
“`python
while True:
user_input = input(“Enter a number (or ‘exit’ to quit): “)
if user_input.lower() == ‘exit’:
break
Additional processing
“`
In this example, the loop will continue until the user types “exit”, effectively preventing it from running indefinitely.
Implementing Timeout Mechanisms
Timeout mechanisms can also help prevent infinite loops. By setting a maximum time for the loop to execute, you can force it to stop after a specified duration. For instance:
“`python
import time
start_time = time.time()
timeout = 5 seconds
while True:
if time.time() – start_time > timeout:
print(“Loop timed out.”)
break
Additional processing
“`
This loop will terminate after 5 seconds, regardless of its internal condition.
Debugging Tools
Utilizing debugging tools can also aid in identifying and resolving infinite loops. Python provides several tools for debugging:
- PDB (Python Debugger): Insert `import pdb; pdb.set_trace()` in your code to set breakpoints.
- Print Statements: Use print statements to monitor variable states and loop iterations.
- Logging: Implement logging to track the flow of execution.
Here’s an example of using print statements to debug:
“`python
count = 0
while count < 5:
print(f"Current count: {count}")
count += 1
if count == 3:
print("Exiting loop early for debugging.")
break
```
Proper Loop Structure
Ensuring that your loop is correctly structured will significantly reduce the chances of running into infinite loops. Here’s a comparison of proper and improper loop structures:
Loop Structure | Comments |
---|---|
while x < 10: |
Proper; will terminate once x reaches 10. |
while True: |
Improper; needs a break condition to avoid infinite execution. |
for i in range(5): |
Proper; terminates after 5 iterations. |
By adhering to structured programming principles and implementing the strategies outlined, you can effectively manage and stop infinite loops in Python.
Identifying Infinite Loops
To effectively stop an infinite loop in Python, first identify its presence. Infinite loops typically occur due to:
- Incorrect loop conditions
- Misconfigured loop control variables
- Absence of break statements
Here are common signs to look for:
Signs of Infinite Loops | Description |
---|---|
High CPU usage | The program consumes excessive CPU resources. |
Unresponsive program | The application becomes unresponsive or freezes. |
No output or progress | Expected output is not generated. |
Stopping Infinite Loops in Development
When developing in an interactive environment, you can halt an infinite loop through several methods:
- Keyboard Interrupt: Press `Ctrl + C` in the terminal or command prompt to stop the execution.
- IDE Stop Button: Use the stop or terminate button in your Integrated Development Environment (IDE).
- Task Manager: On Windows, you can end the Python process via Task Manager. On macOS or Linux, use the `kill` command.
Debugging Infinite Loops
To debug and prevent infinite loops, consider the following strategies:
- Print Statements: Add print statements within the loop to monitor variable values and loop conditions.
- Use a Debugger: Utilize debugging tools (such as `pdb` in Python) to step through the code and inspect the state of variables.
- Write Unit Tests: Create unit tests to validate the logic of loops before executing them in the main program.
Best Practices to Avoid Infinite Loops
Implement these best practices to reduce the risk of creating infinite loops:
- Set Loop Limits: Define a maximum number of iterations for loops, and include a condition to break if that limit is reached.
- Validate Input: Ensure loop control variables are set and modified correctly through user input or other sources.
- Clearly Define Exit Conditions: Use clear and concise exit conditions to avoid ambiguity in loop logic.
Example of an Infinite Loop and Its Resolution
Consider the following example of an infinite loop:
```python
count = 0
while count < 5:
print("Count is:", count)
Missing increment of count
```
To resolve this, ensure the loop control variable is updated:
```python
count = 0
while count < 5:
print("Count is:", count)
count += 1 Incrementing count to avoid infinite loop
```
Using Timeout Mechanisms
In scenarios where loops are expected to run for a limited time, implementing a timeout can be beneficial:
```python
import time
start_time = time.time()
timeout = 5 seconds
while True:
if time.time() - start_time > timeout:
print("Loop timed out.")
break
Loop logic here
```
This code snippet will exit the loop if it runs longer than the specified timeout duration.
Expert Strategies for Halting Infinite Loops in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). "To effectively stop an infinite loop in Python, one must first identify the conditions that lead to the loop. Utilizing debugging tools like Python's built-in debugger, pdb, can help trace the execution flow and pinpoint where the loop fails to terminate."
Michael Tran (Lead Python Developer, Code Solutions Ltd.). "Implementing a timeout mechanism is crucial in preventing infinite loops. By setting a maximum iteration count or a time limit, developers can ensure that their code will exit gracefully even if the loop conditions are not met."
Sarah Kim (Professor of Computer Science, University of Technology). "Understanding the logical structure of your code is key to avoiding infinite loops. Regular code reviews and employing static analysis tools can help catch potential infinite loops during the development phase, before they become a runtime issue."
Frequently Asked Questions (FAQs)
What is an infinite loop in Python?
An infinite loop in Python occurs when a loop continues to execute indefinitely without a terminating condition being met. This can lead to unresponsive programs and excessive resource consumption.
How can I identify an infinite loop in my code?
You can identify an infinite loop by monitoring the program's behavior during execution. If the output does not change and the program becomes unresponsive, it is likely stuck in an infinite loop. Additionally, using debugging tools or print statements can help trace the loop's execution.
What are some common causes of infinite loops in Python?
Common causes include incorrect loop conditions, failure to update loop variables, or logical errors that prevent the loop from reaching a terminating condition. For example, using a condition that always evaluates to true will create an infinite loop.
How can I stop an infinite loop while debugging?
You can stop an infinite loop during debugging by interrupting the program execution. In most development environments, you can do this by pressing `Ctrl + C` in the terminal or using the stop button in the IDE.
What strategies can I use to prevent infinite loops in my code?
To prevent infinite loops, ensure that loop conditions are correctly defined and that loop variables are updated appropriately within the loop. Implementing a maximum iteration count or using break statements can also help avoid infinite loops.
Can I use a timeout mechanism to stop infinite loops?
Yes, you can implement a timeout mechanism using threading or asynchronous programming. By setting a time limit for loop execution, you can forcefully terminate the loop if it exceeds the specified duration, thereby preventing an infinite loop scenario.
In Python, an infinite loop occurs when a loop continues to execute indefinitely without a terminating condition being met. To effectively stop an infinite loop, developers can employ several strategies. These include using control statements such as `break` to exit the loop when a specific condition is satisfied, implementing a timeout mechanism, or utilizing exception handling to catch and respond to unexpected behavior. Understanding the structure of loops and the conditions under which they operate is essential for preventing infinite loops from occurring in the first place.
Another critical approach to managing infinite loops is the use of debugging tools and techniques. By incorporating print statements or logging, developers can gain insights into the loop's execution flow, making it easier to identify the cause of the infinite loop. Additionally, utilizing integrated development environments (IDEs) that provide debugging capabilities can facilitate a more efficient diagnosis and resolution of looping issues.
Ultimately, preventing infinite loops requires a combination of careful coding practices, thorough testing, and an understanding of the logic behind loop constructs. By being vigilant about loop conditions and employing debugging strategies, developers can minimize the risk of encountering infinite loops in their Python programs. This proactive approach not only enhances code reliability but also contributes to overall software quality.
Author Profile
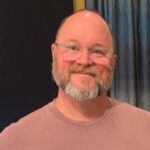
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?