How Can I Start a PowerShell Script from a Batch File?
In the world of Windows automation, the ability to seamlessly integrate different scripting languages can significantly enhance productivity and streamline workflows. One powerful combination that many IT professionals and developers rely on is the pairing of batch files with PowerShell scripts. While batch files have long been a staple for executing simple commands, PowerShell offers a more robust and versatile environment for managing complex tasks and automating system administration. This article delves into the art of starting a PowerShell script from a batch file, unlocking new possibilities for automation and efficiency.
At its core, the process of initiating a PowerShell script from a batch file is about leveraging the strengths of both scripting environments. Batch files, with their straightforward command-line syntax, provide an easy entry point for executing scripts, while PowerShell’s advanced capabilities allow for more sophisticated operations. This synergy not only simplifies the execution of PowerShell scripts but also enables users to create more dynamic and responsive automation solutions.
As we explore the methods and best practices for starting PowerShell scripts from batch files, you’ll discover how to enhance your scripting toolkit. Whether you’re looking to automate routine tasks, manage system configurations, or deploy applications, mastering this integration can empower you to achieve your goals with greater ease and efficiency. Get ready to dive into the practical steps and tips that will elevate your
Creating a Batch File to Execute PowerShell Scripts
To initiate a PowerShell script from a batch file, you need to construct the batch file with specific commands. The general syntax for calling a PowerShell script is as follows:
“`batch
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1”
“`
In this command:
- `powershell` invokes the PowerShell environment.
- `-ExecutionPolicy Bypass` allows the script to run without restriction, which is particularly useful if the execution policy is set to restrict script execution.
- `-File` specifies the path to the PowerShell script that you wish to execute.
When creating your batch file, follow these steps:
- Open Notepad or any text editor.
- Enter the PowerShell command as shown above, replacing `”C:\Path\To\YourScript.ps1″` with the actual path of your PowerShell script.
- Save the file with a `.bat` extension, for example, `RunPowerShellScript.bat`.
Example of a Batch File
Here’s a simple example of what the content of your batch file might look like:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
pause
“`
In this example:
- `@echo off` prevents the commands from being displayed in the command prompt window.
- `pause` keeps the window open until a key is pressed, allowing you to see any output or errors.
Handling Parameters
If your PowerShell script requires parameters, you can pass them directly from the batch file. The syntax is as follows:
“`batch
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
This allows you to customize the execution of your script based on the provided values.
Common Issues and Solutions
When executing a PowerShell script from a batch file, you might encounter several common issues:
Issue | Solution |
---|---|
Script does not run | Check the path and ensure it is correctly formatted. |
Permissions error | Adjust the execution policy or run as administrator. |
Script output not visible | Ensure that the batch file includes a `pause` command. |
Best Practices
To ensure smooth execution and maintainability of your scripts, consider the following best practices:
- Use full paths for scripts to avoid path resolution issues.
- Regularly check and update the execution policy as necessary.
- Implement error handling within your PowerShell scripts to manage exceptions gracefully.
By following these guidelines, you can effectively initiate PowerShell scripts from batch files, leveraging the strengths of both scripting languages for automation tasks.
Creating a Batch File to Execute a PowerShell Script
To start a PowerShell script from a batch file, you need to create a `.bat` file that includes the necessary command to call the PowerShell executable. Here’s how you can do this:
- Open a text editor (like Notepad).
- Write the following command in the text file:
“`batch
@echo off
PowerShell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1”
“`
- `@echo off`: This command disables the display of command lines in the command prompt.
- `PowerShell`: This calls the PowerShell executable.
- `-ExecutionPolicy Bypass`: This allows the script to run without being blocked by execution policies.
- `-File “C:\Path\To\YourScript.ps1″`: This specifies the path to the PowerShell script you want to execute. Replace the path with the actual location of your script.
- Save the file with a `.bat` extension, for example, `RunScript.bat`.
Executing the Batch File
To execute the batch file you created:
- Navigate to the folder containing the `.bat` file using Windows Explorer.
- Double-click the `RunScript.bat` file to run it.
- Alternatively, you can execute it from the Command Prompt by navigating to its directory and typing:
“`cmd
RunScript.bat
“`
Handling Script Arguments
If your PowerShell script requires parameters, you can pass them from the batch file. Modify the command line in the batch file as follows:
“`batch
@echo off
PowerShell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 “Value2”
“`
- Replace `-Param1` and `-Param2` with the actual parameter names expected by your PowerShell script.
Using PowerShell with Different Execution Policies
In situations where you might encounter execution policy restrictions, you can choose from several options:
Execution Policy | Description |
---|---|
Restricted | No scripts can be run. |
AllSigned | Only scripts signed by a trusted publisher can be run. |
RemoteSigned | Scripts created locally can run; remote scripts need signing. |
Unrestricted | All scripts can be run (not recommended for security). |
For temporary changes, use `-ExecutionPolicy Bypass` as shown earlier. However, for permanent changes, consider using PowerShell:
“`powershell
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
“`
Verifying the PowerShell Script Execution
To confirm that the script has executed successfully, you can include logging in your PowerShell script. Here’s an example of logging to a text file:
“`powershell
Start-Transcript -Path “C:\Path\To\LogFile.txt”
Your script code here
Stop-Transcript
“`
This command will record all output from the script into the specified log file, making it easier to debug any issues.
Common Troubleshooting Tips
If you encounter issues while running the batch file, consider the following tips:
- Check the script path: Ensure that the path to the PowerShell script is correct.
- Verify execution policy: Make sure the execution policy allows the script to run.
- Run as administrator: Some scripts may require elevated permissions to execute successfully.
- Review error messages: If the PowerShell script fails, check the error messages for clues.
Expert Insights on Starting PowerShell Scripts from Batch Files
Dr. Emily Carter (Senior Systems Engineer, Tech Innovations Inc.). “When automating tasks in Windows environments, starting a PowerShell script from a batch file can significantly streamline processes. It allows for better integration of legacy systems with modern scripting capabilities, enhancing overall efficiency.”
Michael Tran (IT Automation Specialist, FutureTech Solutions). “Utilizing batch files to initiate PowerShell scripts is a powerful technique for system administrators. It not only simplifies script execution but also enables the use of conditional logic, making it easier to manage complex workflows in a controlled manner.”
Linda Patel (Cybersecurity Consultant, SecureTech Advisors). “From a security perspective, starting PowerShell scripts through batch files can introduce vulnerabilities if not handled correctly. It is essential to implement proper permissions and validation checks to mitigate risks associated with script execution.”
Frequently Asked Questions (FAQs)
How can I start a PowerShell script from a batch file?
You can start a PowerShell script from a batch file by using the command `powershell -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1″`. This command allows the batch file to execute the specified PowerShell script.
What does the ExecutionPolicy Bypass option do?
The ExecutionPolicy Bypass option allows the script to run without being blocked by the current PowerShell execution policy. This is particularly useful when running scripts that are not digitally signed.
Can I pass parameters to a PowerShell script from a batch file?
Yes, you can pass parameters by appending them to the script call. For example: `powershell -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1” -Param1 Value1 -Param2 Value2`.
Is it necessary to specify the full path to the PowerShell script?
While it is not strictly necessary if the script is in the same directory as the batch file, it is a best practice to specify the full path to avoid any issues with the script not being found.
What should I do if the PowerShell script does not execute?
If the script does not execute, check for errors in the command syntax, ensure the script path is correct, verify that the PowerShell execution policy allows script execution, and confirm that you have the necessary permissions to run the script.
Can I run a PowerShell script silently from a batch file?
Yes, you can run a PowerShell script silently by using the `-WindowStyle Hidden` parameter. The command would look like: `powershell -ExecutionPolicy Bypass -WindowStyle Hidden -File “C:\path\to\your\script.ps1″`. This suppresses the PowerShell window during execution.
In summary, starting a PowerShell script from a batch file is a straightforward process that can significantly enhance automation capabilities in Windows environments. By using the `powershell` command within a batch file, users can execute PowerShell scripts seamlessly, allowing for the integration of both scripting languages. This method is particularly useful for system administrators and developers who wish to leverage the strengths of PowerShell while maintaining compatibility with traditional batch file operations.
Key takeaways include the importance of understanding the syntax required to invoke PowerShell from a batch file. Users should be aware of the need to specify the full path to the PowerShell executable and the script file, particularly when dealing with scripts located in directories that may not be included in the system’s PATH variable. Additionally, using the `-ExecutionPolicy Bypass` option can help avoid permission issues when running scripts, ensuring a smoother execution process.
Moreover, users should consider error handling and logging within their scripts to facilitate troubleshooting and improve reliability. By incorporating these practices, the execution of PowerShell scripts from batch files can become a robust solution for automating tasks, managing system configurations, and streamlining workflows in various IT scenarios.
Author Profile
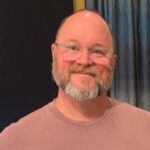
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?