How Can You Fix the ‘Illegal Start of Expression’ Error in Java?
If you’ve ever dived into the world of Java programming, you may have encountered the perplexing error message: “Illegal start of expression.” This seemingly cryptic notification can halt your coding progress and leave you scratching your head in confusion. Understanding this error is crucial for any Java developer, whether you’re a novice just starting your coding journey or a seasoned programmer looking to refine your skills. In this article, we will unravel the mystery behind this common issue, equipping you with the knowledge to identify, troubleshoot, and ultimately fix it with confidence.
At its core, the “Illegal start of expression” error typically arises from syntax issues within your Java code. It can be triggered by a variety of factors, such as misplaced brackets, incorrect use of keywords, or even simple typographical errors. These seemingly minor mistakes can lead to significant roadblocks in your development process, making it essential to understand how to spot and rectify them effectively.
As we delve deeper into this topic, we will explore the common scenarios that lead to this error, providing you with practical insights and tips to enhance your coding practices. By the end of this article, you’ll not only be able to fix the “Illegal start of expression” error but also gain a greater appreciation for the nuances of Java syntax
Common Causes of Illegal Start of Expression
The “Illegal Start of Expression” error in Java can arise from several common coding mistakes. Understanding these causes can help in quickly identifying and rectifying the issue.
- Missing or Incorrect Punctuation: Often, this error occurs due to missing semicolons or commas. For example, forgetting a semicolon at the end of a statement will lead to this error.
- Misplaced Curly Braces: If your opening and closing braces do not match, it can confuse the Java compiler, leading to this error.
- Improper Use of Keywords: Using Java keywords incorrectly or out of context can trigger this error. For instance, using `public` before a variable declaration outside of a class context.
- Incorrect Method Declaration: If a method is declared incorrectly, such as omitting the return type, the compiler can produce this error.
Examples of Illegal Start of Expression
Here are some code snippets that illustrate common scenarios leading to the “Illegal Start of Expression” error:
“`java
public class Example {
int x = 5 // Missing semicolon
int y = 10;
void method() {
System.out.println(x + y);
}
}
“`
“`java
public class Example {
void method() {
int a = 10;
if (a > 5) { // Missing closing brace for if statement
System.out.println(“Greater than 5”);
}
}
“`
In these examples, the missing semicolon and the misplaced brace create situations where the Java compiler cannot process the code correctly, resulting in the error.
How to Fix the Error
To resolve the “Illegal Start of Expression” error, consider the following steps:
- Review Syntax: Check for missing punctuation, such as semicolons, commas, and closing braces.
- Check Keywords: Ensure all Java keywords are used correctly and in the appropriate context.
- Method Declarations: Confirm that method declarations include return types and are correctly structured.
- Use an IDE: Integrated Development Environments (IDEs) can provide real-time feedback and highlight syntax errors, making it easier to spot issues.
Common Errors | Potential Fixes |
---|---|
Missing semicolon | Add a semicolon at the end of the statement. |
Misplaced braces | Ensure that opening and closing braces match and are correctly placed. |
Incorrect use of keywords | Review the context of the keywords and adjust as necessary. |
Improper method declaration | Include a return type and ensure proper syntax. |
By carefully reviewing your code and employing these strategies, you can effectively resolve the “Illegal Start of Expression” error and improve the overall quality of your Java programming.
Common Causes of Illegal Start of Expression
The “Illegal Start of Expression” error in Java typically arises from syntax issues. Understanding the common causes can help in diagnosing and fixing the problem efficiently.
- Missing Semicolons: Java statements must end with a semicolon. Omitting this can lead to confusion in the parser.
- Incorrect Bracket Placement: Mismatched or misplaced curly braces `{}`, parentheses `()`, or square brackets `[]` can disrupt the flow of code.
- Unexpected Tokens: Using keywords or operators incorrectly or placing them in unexpected positions can trigger this error.
- Variable Declaration Issues: Declaring a variable without a proper type or using it in an incorrect context will result in an error.
- Improper Use of Access Modifiers: Access modifiers such as `public`, `private`, and `protected` must precede data types in variable declarations and method signatures.
How to Identify the Error
To effectively identify where the “Illegal Start of Expression” error occurs, follow these steps:
- Read Compiler Messages: The Java compiler provides line numbers and error messages that can help pinpoint the issue.
- Check Surrounding Code: Examine the lines before and after the flagged line for potential syntax errors.
- Use an IDE: Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse highlight syntax errors in real-time, making it easier to spot issues.
- Simplify the Code: If the error is difficult to locate, simplify the code by commenting out portions to isolate the problematic segment.
Examples of Illegal Start of Expression
Understanding specific examples can clarify what constitutes an illegal start of expression.
Example Code | Error Explanation |
---|---|
int a = 5 // Missing semicolon | Missing semicolon causes the compiler to misinterpret the following line. |
if (a > 5 { // Incorrect bracket placement | Mismatched parentheses; opening brace should follow the closing parenthesis. |
public void method() int x = 10; // Incorrect declaration | Missing a semicolon between method declaration and variable declaration. |
private class MyClass {} // Access modifier error | Access modifier cannot precede class declaration in this context. |
Best Practices to Avoid This Error
To minimize the occurrence of “Illegal Start of Expression” errors, consider the following best practices:
- Consistent Indentation: Maintain consistent indentation for better readability and to help identify blocks of code.
- Code Comments: Use comments to document complex sections, making it easier to trace logic and syntax.
- Regular Code Reviews: Engage in peer code reviews to catch errors that may be overlooked.
- Write Small Methods: Break code into smaller methods to reduce complexity and potential errors.
- Utilize Linting Tools: Incorporate linting tools that analyze code for potential issues and enforce coding standards.
By adhering to these practices, developers can significantly reduce the likelihood of encountering syntax-related errors in Java.
Expert Insights on Resolving Illegal Start of Expression in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Illegal Start of Expression’ error in Java often arises from syntax issues such as missing semicolons or incorrect placement of braces. It is crucial to review your code for these common pitfalls to ensure proper structure and flow.”
Michael Chen (Java Development Specialist, CodeCraft Academy). “To effectively fix the ‘Illegal Start of Expression’ error, developers should utilize an IDE with syntax highlighting features. This can help identify misplaced keywords and brackets that lead to confusion in the code.”
Sarah Thompson (Lead Java Instructor, Advanced Coding Bootcamp). “Understanding the context of your code is essential. Often, this error can be traced back to a misplaced variable declaration or a method that lacks proper syntax. A thorough line-by-line review is recommended to pinpoint the exact cause.”
Frequently Asked Questions (FAQs)
What does “Illegal Start of Expression” mean in Java?
“Illegal Start of Expression” is a compilation error in Java that occurs when the Java compiler encounters code that does not conform to the expected syntax. This often results from misplaced symbols, missing keywords, or incorrect structure in the code.
What are common causes of the “Illegal Start of Expression” error?
Common causes include missing semicolons, misplaced brackets, incorrect variable declarations, or using reserved keywords improperly. Syntax errors, such as forgetting to close a parenthesis or using an incorrect data type, can also trigger this error.
How can I identify the source of the error in my code?
To identify the source of the error, carefully review the line number indicated in the error message. Check for syntax issues, such as missing punctuation or incorrect placement of keywords. Using an Integrated Development Environment (IDE) can also help highlight syntax errors.
What steps can I take to fix the “Illegal Start of Expression” error?
To fix the error, examine your code for syntax mistakes. Ensure all statements are correctly terminated with semicolons, verify that brackets and parentheses are properly matched, and check for correct variable declarations. Refactoring the code for clarity can also help.
Can IDEs help prevent “Illegal Start of Expression” errors?
Yes, many IDEs provide real-time syntax checking and code suggestions, which can help prevent “Illegal Start of Expression” errors. They often highlight potential issues as you type, allowing you to correct mistakes before compiling the code.
Is it possible to encounter this error in Java frameworks or libraries?
Yes, this error can occur in Java frameworks or libraries if the code you write does not adhere to the expected syntax of the framework. Always refer to the documentation for the specific framework to ensure proper usage and syntax.
In Java programming, encountering the “Illegal Start of Expression” error is a common issue that can arise from various coding mistakes. This error typically indicates that the Java compiler has identified a syntax problem in the code that prevents it from being properly parsed. Common causes include misplaced or missing punctuation, incorrect use of keywords, and improper placement of code blocks. Understanding these potential pitfalls is essential for developers seeking to write clean and functional Java code.
To effectively resolve this error, developers should carefully review their code for syntax issues. This includes checking for missing semicolons, ensuring that parentheses and braces are correctly matched, and verifying that variable declarations are appropriately formatted. Additionally, it is crucial to be aware of Java’s reserved keywords and to avoid using them inappropriately within expressions. By paying close attention to these details, programmers can significantly reduce the likelihood of encountering this error.
In summary, the “Illegal Start of Expression” error serves as a reminder of the importance of syntax in Java programming. By developing a keen eye for detail and adhering to Java’s syntax rules, developers can enhance their coding skills and improve the overall quality of their applications. Continuous practice and code reviews can further aid in identifying and correcting such errors, leading to more efficient and
Author Profile
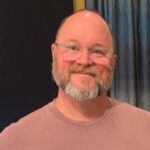
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?