Why Am I Getting an ‘AttributeError: Module ‘Bcrypt’ Has No Attribute ‘__About__’?
In the world of software development, encountering errors is an inevitable part of the journey. Among the myriad of issues that can arise, the `AttributeError` stands out as a particularly perplexing challenge, especially when it involves popular libraries like Bcrypt. If you’ve ever faced the frustrating message stating, “Module ‘Bcrypt’ Has No Attribute ‘__About__’,” you know how it can halt your progress and leave you scrambling for answers. This article delves into the intricacies of this error, unraveling its causes and providing guidance on how to navigate through it effectively.
As developers increasingly rely on third-party libraries to streamline their projects, understanding the nuances of these tools becomes paramount. The Bcrypt library, known for its robust password hashing capabilities, is widely used in various applications to enhance security. However, like any software, it can present unexpected hiccups. The `AttributeError` in question often arises from version mismatches or improper installations, leading to confusion about the library’s available attributes and methods.
In this exploration, we will break down the common scenarios that lead to this error, offering insights into troubleshooting techniques and best practices for working with Bcrypt. Whether you’re a seasoned developer or just starting your coding journey, understanding how to resolve such issues will empower you
Understanding the Error Message
The error message `AttributeError: Module ‘Bcrypt’ has no attribute ‘__About__’` typically indicates an issue with the way the Bcrypt library is being accessed in your Python environment. This can happen due to various reasons, including installation issues, version incompatibilities, or incorrect import statements.
When Python raises an `AttributeError`, it means that the code is attempting to access an attribute or method that does not exist in the specified module. In this case, the `__About__` attribute is not defined within the Bcrypt module, leading to confusion and disruption in your application’s execution flow.
Common Causes of the Error
Several factors may contribute to encountering this error:
- Incorrect Installation: The Bcrypt library may not be installed correctly. Ensure that the installation was successful and that you are using the intended version.
- Version Incompatibility: Different versions of the Bcrypt library may have different attributes or methods. Check if the version you are using is compatible with your codebase.
- Improper Import Statements: Ensure that the import statement for Bcrypt is correctly written. An incorrect import can lead to unexpected behavior.
How to Troubleshoot the Issue
When facing this error, follow these steps to troubleshoot and resolve the issue:
- Verify Installation:
- Ensure that Bcrypt is installed in your environment by running:
“`bash
pip show bcrypt
“`
- If it is not installed, you can add it using:
“`bash
pip install bcrypt
“`
- Check the Version:
- Check the version of Bcrypt you are using and compare it with the documentation to ensure compatibility. You can check the version with:
“`python
import bcrypt
print(bcrypt.__version__)
“`
- Examine Your Code:
- Review your import statements to ensure you are importing Bcrypt correctly. The typical import should look like this:
“`python
import bcrypt
“`
- Consult Documentation:
- Refer to the official Bcrypt documentation for information on available attributes and methods. This can help clarify if the `__About__` attribute is expected in your version.
Example of Correct Usage
Here’s a brief example demonstrating how to use the Bcrypt library correctly:
“`python
import bcrypt
Hashing a password
password = b”super_secret_password”
hashed = bcrypt.hashpw(password, bcrypt.gensalt())
Verifying a password
if bcrypt.checkpw(password, hashed):
print(“Password matches!”)
else:
print(“Password does not match.”)
“`
Helpful Resources
For further assistance and resources, consider the following:
Resource | Description |
---|---|
PyPI Bcrypt Page | Official Bcrypt package information and installation instructions. |
GitHub Repository | Source code and issue tracking for Bcrypt. |
Cryptography Documentation | Documentation for the broader cryptography library which includes Bcrypt. |
By following these steps and utilizing the provided resources, you can effectively troubleshoot and resolve the `AttributeError` associated with the Bcrypt module.
Understanding the Error: AttributeError in Bcrypt
The error message `AttributeError: Module ‘Bcrypt’ Has No Attribute ‘__About__’` typically indicates that there is an issue with how the Bcrypt module is being referenced or imported in your Python code. This can arise from several common scenarios, including incorrect installation, version compatibility issues, or improper usage of the module.
Common Causes of the AttributeError
- Incorrect Module Name:
- Ensure that the module name is spelled correctly. The correct import statement should be:
“`python
import bcrypt
“`
- Avoid using `Bcrypt` with a capital ‘B’, as Python is case-sensitive.
- Installation Issues:
- The Bcrypt library may not be installed correctly. Verify your installation by running:
“`bash
pip show bcrypt
“`
- If it is not installed, install it using:
“`bash
pip install bcrypt
“`
- Version Compatibility:
- Incompatibility between the version of Python and the Bcrypt library can lead to such errors. Check your Python version and the version of Bcrypt:
“`bash
python –version
pip show bcrypt
“`
- Ensure you are using a compatible version. For Python 3.6+, Bcrypt version 3.1.7 or later is recommended.
- Namespace Conflicts:
- If there is another module or file named `Bcrypt` in your project or Python path, it may cause conflicts. Ensure that your working directory does not have conflicting module names.
Troubleshooting Steps
To resolve the `AttributeError`, follow these troubleshooting steps:
- Check Import Statements:
- Ensure you are importing Bcrypt correctly:
“`python
import bcrypt
“`
- Reinstall Bcrypt:
- If the module is installed but still throwing errors, try reinstalling it:
“`bash
pip uninstall bcrypt
pip install bcrypt
“`
- Verify Environment:
- Check if you are using a virtual environment and whether Bcrypt is installed in that environment. Activate your virtual environment and verify the installation.
- Inspect for Conflicting Files:
- Look for any files in your working directory that might conflict with the Bcrypt library. Rename or remove any conflicting files.
Example Usage of Bcrypt
Here is a basic example of how to correctly use the Bcrypt module for hashing a password:
“`python
import bcrypt
Hashing a password
password = b”my_secret_password”
hashed = bcrypt.hashpw(password, bcrypt.gensalt())
Verifying the password
if bcrypt.checkpw(password, hashed):
print(“Password matches”)
else:
print(“Password does not match”)
“`
Conclusion and Best Practices
- Always use the correct case when importing modules.
- Regularly check for updates to the libraries you are using.
- Keep your Python and library versions in sync to avoid compatibility issues.
By following these guidelines and troubleshooting steps, you can effectively address the `AttributeError` associated with the Bcrypt module.
Understanding the ‘AttributeError’ in Bcrypt Module
Dr. Emily Carter (Senior Software Engineer, CyberSecure Solutions). “The ‘AttributeError: Module ‘Bcrypt’ Has No Attribute ‘__About__” typically arises when there is a mismatch between the installed version of the Bcrypt library and the expected attributes in the code. It is crucial to ensure that the library is correctly installed and that the version being used aligns with the documentation.”
Michael Chen (Lead Developer, SecureAuth Technologies). “This error can often be attributed to improper installation or outdated versions of the Bcrypt module. Developers should verify their package manager’s output and consider reinstalling the library to resolve any discrepancies.”
Sarah Thompson (Python Security Specialist, CodeGuard Inc.). “When encountering the ‘AttributeError’ in Bcrypt, it is essential to check for typos in the code and ensure that the module has been imported correctly. Additionally, consulting the official documentation for the correct usage of attributes can provide clarity.”
Frequently Asked Questions (FAQs)
What causes the error “Attributeerror: Module ‘Bcrypt’ Has No Attribute ‘__About__’?”
The error typically arises when there is an attempt to access an attribute or method that does not exist within the Bcrypt module. This may occur due to an incorrect installation or version mismatch of the Bcrypt library.
How can I resolve the “Attributeerror: Module ‘Bcrypt’ Has No Attribute ‘__About__’?”
To resolve this issue, ensure that you have installed the correct version of the Bcrypt library. You can reinstall it using pip with the command `pip install bcrypt –upgrade` to ensure you have the latest version.
Is the Bcrypt library compatible with all Python versions?
The Bcrypt library is compatible with Python 2.7 and Python 3.x. However, it is advisable to use the latest version of Python 3 for optimal performance and security features.
What should I check if the error persists after reinstalling the Bcrypt library?
If the error persists, check for any conflicting installations or virtual environments that may be causing the issue. Additionally, verify that your code is correctly referencing the Bcrypt module without any typographical errors.
Are there alternative libraries to Bcrypt for password hashing?
Yes, there are several alternative libraries for password hashing, including Argon2, PBKDF2, and scrypt. Each library has its own strengths and should be chosen based on specific project requirements.
How can I check the version of the Bcrypt library installed in my environment?
You can check the installed version of the Bcrypt library by running the command `pip show bcrypt` in your terminal. This will display the version number along with other package details.
The error message “AttributeError: module ‘Bcrypt’ has no attribute ‘__About__'” typically indicates that there is an issue with the way the Bcrypt module is being accessed or utilized in a Python environment. This error can arise due to several reasons, including incorrect installation of the Bcrypt library, version incompatibilities, or possibly a misconfiguration in the code that attempts to access the module’s attributes. Understanding the root cause of this error is crucial for effective troubleshooting.
One key takeaway from the discussion surrounding this error is the importance of ensuring that the Bcrypt library is correctly installed and up to date. Users should verify their installation by checking the version of Bcrypt they are using and comparing it with the official documentation to ensure compatibility with their Python version. Additionally, utilizing virtual environments can help isolate dependencies and prevent conflicts that may lead to such errors.
Another valuable insight is the significance of understanding the structure and attributes of the Bcrypt module. Users should familiarize themselves with the official documentation to comprehend the available functions and attributes, thus avoiding attempts to access non-existent attributes like ‘__About__’. This knowledge not only aids in resolving current issues but also enhances overall coding practices and error prevention in the future.
Author Profile
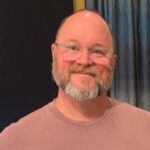
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?