How Can a Function in Python Return More Than One Value?
In the world of programming, particularly in Python, the ability to return multiple values from a single function can be a game-changer. Imagine crafting a function that not only performs a calculation but also provides various outputs, all neatly packaged in one call. This powerful feature enhances code readability, efficiency, and flexibility, allowing developers to streamline their workflows and reduce redundancy. As you delve into the intricacies of Python functions, you’ll discover how this capability can elevate your coding practices and simplify complex tasks.
Returning multiple values in Python is not just a convenience; it’s an integral part of the language’s design that encourages clean and effective coding. By leveraging tuples, lists, or dictionaries, Python functions can deliver a wealth of information in a single return statement. This approach not only minimizes the need for multiple function calls but also allows for more intuitive data handling, making it easier to manage related outputs.
As you explore this topic further, you’ll uncover practical examples and scenarios where returning multiple values can significantly enhance your programming experience. Whether you’re developing a simple application or tackling more complex projects, understanding how to effectively utilize this feature will empower you to write more dynamic and responsive code. Get ready to unlock the full potential of your Python functions and transform the way you approach programming challenges!
Returning Multiple Values from a Function
In Python, functions can return more than one value using various methods. The most common approach is to return a tuple, but other data structures like lists or dictionaries can also be utilized. This feature enhances the flexibility of functions, allowing them to provide multiple outputs in a clean and efficient manner.
When a function returns multiple values, they can be unpacked into separate variables in a single statement. For example:
“`python
def calculate_statistics(values):
total = sum(values)
mean = total / len(values)
return total, mean
total, mean = calculate_statistics([10, 20, 30])
“`
In this example, the function `calculate_statistics` returns both the total and the mean of a list of numbers. The returned values are easily unpacked into the variables `total` and `mean`.
Using Tuples to Return Multiple Values
Tuples are immutable sequences in Python, making them ideal for returning multiple values. When a function returns a tuple, it is enclosed in parentheses, and the values can be accessed by their index if needed.
Example:
“`python
def get_coordinates():
return (10, 20)
x, y = get_coordinates()
“`
In this case, the function returns a tuple containing two values, which are unpacked into the variables `x` and `y`.
Returning Lists
Another way to return multiple values is by using lists. This method is particularly useful when the number of returned items may vary or when they need to be manipulated further.
Example:
“`python
def generate_numbers(n):
return [i for i in range(n)]
numbers = generate_numbers(5)
“`
The function `generate_numbers` returns a list of integers, allowing for further operations like sorting or filtering.
Returning Dictionaries
Dictionaries can also be used to return multiple values, especially when the values are associated with specific keys. This method improves code readability by providing context for each returned value.
Example:
“`python
def get_user_info(name, age):
return {‘name’: name, ‘age’: age}
user_info = get_user_info(‘Alice’, 30)
“`
Here, the function returns a dictionary with keys ‘name’ and ‘age’, making it clear what each value represents.
Comparison of Return Types
The following table summarizes the advantages and use cases for each return type:
Return Type | Advantages | Use Case |
---|---|---|
Tuple | Immutable, concise | Fixed number of related values |
List | Mutable, variable length | Dynamic number of items, sequences |
Dictionary | Key-value pairs, readable | Named values for clarity |
By understanding how to effectively return multiple values from functions, developers can create more robust and organized code. Each return type has its specific advantages and should be chosen based on the context and requirements of the task at hand.
Returning Multiple Values from a Function
In Python, functions can return multiple values using several techniques. The most common and straightforward method is to return values as a tuple. When you return multiple values, they are automatically packed into a tuple, which can then be unpacked by the caller.
Using Tuples to Return Multiple Values
When a function returns multiple values, Python packages them into a tuple. Here’s how it works:
“`python
def calculate_statistics(numbers):
total = sum(numbers)
average = total / len(numbers)
return total, average
result = calculate_statistics([10, 20, 30, 40])
print(result) Output: (100, 25.0)
“`
In this example, the `calculate_statistics` function returns both the total and average of a list of numbers. The caller can unpack these values easily:
“`python
total, average = calculate_statistics([10, 20, 30, 40])
print(f’Total: {total}, Average: {average}’) Output: Total: 100, Average: 25.0
“`
Returning Lists or Dictionaries
While tuples are a common choice, lists and dictionaries can also be used to return multiple values, especially when the returned data is more complex or requires named access.
Returning a List:
“`python
def get_scores():
return [90, 85, 88]
scores = get_scores()
print(scores) Output: [90, 85, 88]
“`
Returning a Dictionary:
“`python
def get_student_info():
return {‘name’: ‘Alice’, ‘age’: 21, ‘grade’: ‘A’}
student_info = get_student_info()
print(student_info[‘name’]) Output: Alice
“`
Using a dictionary provides clarity, especially when the function returns a significant amount of data.
Using Named Tuples for Better Readability
For cases where readability and maintainability are critical, using named tuples can be advantageous. Named tuples allow you to access the returned values by name instead of index.
“`python
from collections import namedtuple
def get_coordinates():
Point = namedtuple(‘Point’, [‘x’, ‘y’])
return Point(10, 20)
point = get_coordinates()
print(point.x, point.y) Output: 10 20
“`
Named tuples enhance code clarity, making it easier to understand what each value represents.
Returning Values as a Data Class
Python 3.7 introduced data classes, which are a more modern approach to returning multiple values in a structured manner. Data classes provide a way to define classes that are primarily used to store data with less boilerplate code.
“`python
from dataclasses import dataclass
@dataclass
class Student:
name: str
age: int
grade: str
def get_student():
return Student(‘Bob’, 22, ‘B’)
student = get_student()
print(student.name, student.age, student.grade) Output: Bob 22 B
“`
Using data classes not only improves readability but also includes built-in methods for comparison and representation.
Summary of Techniques
Technique | Description |
---|---|
Tuple | Simple and efficient for returning multiple values. |
List | Useful for returning an ordered collection of values. |
Dictionary | Provides named access to returned values for better clarity. |
Named Tuple | Enhances readability by allowing access through named fields. |
Data Class | A modern approach to encapsulate multiple related values. |
Each of these methods can be employed based on the context and complexity of the data being returned, allowing for flexible and readable code.
Expert Insights on Returning Multiple Values in Python Functions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the ability of a function to return multiple values is not only a powerful feature but also enhances code readability and maintainability. By using tuples, lists, or dictionaries, developers can encapsulate related data and make their functions more versatile.”
Michael Chen (Lead Data Scientist, AI Solutions Group). “Returning multiple values from a function in Python allows for efficient data handling, especially in data analysis and machine learning applications. This feature simplifies the process of unpacking results and reduces the need for complex data structures.”
Sarah Patel (Python Instructor, Code Academy). “Understanding how to effectively return multiple values is crucial for any Python programmer. It not only streamlines workflows but also encourages a more functional programming approach, which can lead to cleaner and more efficient code.”
Frequently Asked Questions (FAQs)
Can a function in Python return multiple values?
Yes, a function in Python can return multiple values by separating them with commas. These values are returned as a tuple.
How do I unpack multiple return values from a function?
You can unpack multiple return values by assigning them to separate variables. For example, `a, b = my_function()` will assign the first value to `a` and the second to `b`.
What happens if I return more values than variables to unpack them?
If you return more values than variables, Python will raise a `ValueError` indicating that there are not enough values to unpack.
Can I return different data types from a function?
Yes, a function can return different data types simultaneously. For instance, you can return an integer, a string, and a list in a single return statement.
Is it possible to return a list or dictionary containing multiple values?
Yes, you can return a list or dictionary containing multiple values. This allows for more complex data structures to be returned from a function.
What is the advantage of returning multiple values from a function?
Returning multiple values enhances code readability and functionality by allowing a single function to provide comprehensive results, reducing the need for multiple function calls.
In Python, functions possess the capability to return multiple values simultaneously, a feature that distinguishes it from many other programming languages. This is accomplished through the use of tuples, lists, or dictionaries, allowing developers to package multiple outputs in a single return statement. By returning multiple values, Python enhances the flexibility and efficiency of function design, enabling the encapsulation of related data and minimizing the need for multiple return statements.
One of the key advantages of returning multiple values is the simplification of code. Instead of creating complex data structures or relying on global variables, developers can easily manage related outputs. This approach not only improves code readability but also fosters better organization, making it easier to maintain and debug. Furthermore, this feature encourages the use of unpacking, which allows for intuitive assignment of returned values to individual variables, thereby enhancing the overall coding experience.
In summary, the ability of Python functions to return more than one value is a powerful tool that promotes efficient coding practices. It allows for better data management and contributes to cleaner, more maintainable code. As developers continue to leverage this feature, they can create more sophisticated applications that effectively handle multiple outputs without unnecessary complexity.
Author Profile
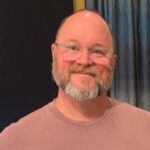
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?