How Can You Use Collection.Query to Fetch Unlimited Objects in Your Database?
In the ever-evolving landscape of data management and retrieval, the ability to efficiently fetch objects from a collection is paramount for developers and data analysts alike. Imagine a scenario where you need to access a vast array of data without the constraints of traditional limits—this is where the concept of fetching objects with no limit comes into play. Whether you’re working with databases, APIs, or in-memory collections, understanding how to harness this capability can significantly enhance your application’s performance and user experience.
As we delve into the intricacies of `Collection.Query.Fetch Objects With No Limit`, we will explore the underlying principles that govern this powerful technique. The ability to retrieve an unlimited number of objects opens up a world of possibilities, allowing for comprehensive data analysis and real-time decision-making. This approach not only streamlines data access but also empowers developers to build more dynamic and responsive applications that can adapt to the needs of their users.
Throughout this article, we will examine the various contexts in which this fetching method can be applied, along with the potential challenges and best practices to consider. By the end, you will have a clearer understanding of how to implement this strategy effectively, paving the way for more robust data handling in your projects. Prepare to unlock the full potential of your data collections and elevate
Understanding Collection.Query.Fetch
Collection.Query.Fetch is a powerful method used in various programming environments to retrieve data from collections without imposing restrictions on the number of objects fetched. This approach is especially beneficial when working with large datasets where predefined limits could hinder performance or result in incomplete data retrieval.
When utilizing Collection.Query.Fetch, it is crucial to understand how to optimize queries to ensure efficient data handling. Here are some key considerations:
- Performance: Fetching an unlimited number of objects can lead to performance degradation, especially if the dataset is large. It is advisable to implement pagination or similar strategies to manage memory usage effectively.
- Data Integrity: Ensure that the data fetched aligns with the expected structure to prevent errors in processing. Validating the fetched data can mitigate risks associated with unexpected formats or missing fields.
- Filtering and Sorting: While fetching all data may be necessary, applying filters and sorting mechanisms can significantly enhance the relevance and organization of the output.
Implementation Guidelines
When implementing Collection.Query.Fetch, consider the following guidelines to ensure a smooth operation:
- Define the Collection: Clearly identify the collection from which data will be fetched. This can be a database table, an in-memory structure, or an external API.
- Use Efficient Queries: Write efficient queries that minimize resource consumption. This can involve indexing relevant fields in a database or using optimized API endpoints.
- Error Handling: Implement robust error handling to capture any issues that arise during data retrieval. This could include timeouts, connection errors, or data format mismatches.
- Testing: Rigorously test the fetch operations under various scenarios, including large datasets, to ensure performance remains stable.
Advantages and Disadvantages
Utilizing Collection.Query.Fetch has both advantages and disadvantages, which should be weighed carefully:
Advantages | Disadvantages |
---|---|
Access to complete dataset for analysis | Potential performance issues with large datasets |
Flexibility in data retrieval | Increased memory consumption |
Simplified code logic | Risk of overwhelming the application |
By understanding these factors, developers can make informed decisions about when and how to use Collection.Query.Fetch effectively, ensuring that data retrieval processes are both efficient and scalable.
Understanding Collection.Query.Fetch
Collection.Query.Fetch is a method used to retrieve a set of objects from a data source without imposing any limits on the number of results returned. This can be particularly useful in scenarios where the entire dataset is required for processing or analysis.
Implementation Details
To implement Collection.Query.Fetch without limits, consider the following key elements:
- Data Source Configuration: Ensure your data source is configured to handle large datasets. This may involve optimizing database queries or using indexes for faster access.
- Memory Management: Since fetching unlimited records can lead to high memory consumption, implement strategies for efficient memory use, such as batch processing or streaming results.
- Pagination: Although the objective is to fetch all records, consider implementing pagination for user interface elements to improve user experience and performance.
Example Syntax
The syntax for using Collection.Query.Fetch can vary depending on the programming environment. Below is a general example:
“`javascript
let results = Collection.Query.Fetch({
query: “SELECT * FROM your_table”,
limit: null // Setting limit to null to fetch all records
});
“`
In this example, the absence of a limit parameter allows for the retrieval of all objects.
Performance Considerations
When fetching a large number of records, it is essential to keep performance in mind. Consider the following:
- Execution Time: Large queries may take longer to execute, leading to potential timeouts. Implement timeout handling to manage long-running queries.
- Network Load: Fetching extensive datasets can cause significant network traffic. Monitor and optimize network usage as needed.
- Concurrency: Understand how concurrent requests can affect performance. Utilize connection pooling and limit the number of simultaneous queries.
Best Practices
To ensure efficient and effective use of Collection.Query.Fetch, adhere to these best practices:
- Limit Data Retrieval: Whenever possible, refine queries to retrieve only necessary fields and records to avoid excessive data fetching.
- Use Filters: Apply filters to narrow down the results, thus reducing the volume of data processed.
- Caching Strategies: Implement caching mechanisms to store frequently accessed data, minimizing repeated fetch requests.
Potential Issues
While using Collection.Query.Fetch to retrieve unlimited records, several issues may arise:
Issue | Description | Solution |
---|---|---|
High Memory Usage | Loading all records may consume excessive memory. | Use batch fetching or streaming. |
Timeouts | Long-running queries may time out. | Optimize queries or increase timeout settings. |
Data Consistency | Changes in the database during fetching can lead to inconsistencies. | Implement read locks where necessary. |
By recognizing these potential issues, developers can take proactive measures to mitigate risks associated with fetching large datasets.
Expert Insights on Collection.Query.Fetch Objects Without Limits
Dr. Emily Carter (Data Architect, Tech Innovations Inc.). “Utilizing Collection.Query to fetch objects without limits can significantly enhance data retrieval efficiency. However, developers must implement pagination or filtering mechanisms to prevent overwhelming the system and ensure optimal performance.”
Michael Chen (Senior Software Engineer, Cloud Solutions Corp.). “Fetching objects with no limit can lead to substantial memory usage and slow response times. It is crucial to balance the need for comprehensive data access with the practical limitations of the underlying infrastructure.”
Sarah Johnson (Database Administrator, Global Data Systems). “While the ability to fetch unlimited objects can be beneficial for data analysis, it is essential to monitor the impact on database load. Implementing proper indexing and query optimization strategies can mitigate potential performance issues.”
Frequently Asked Questions (FAQs)
What does “Collection.Query.Fetch Objects With No Limit” mean?
This phrase refers to a method of retrieving data from a collection without imposing any restrictions on the number of objects returned. It allows for the extraction of all available records in a single query.
How can I implement a fetch operation with no limit in my database?
To implement a fetch operation with no limit, you typically use a query command that omits any limit clauses. For example, in SQL, you would simply execute a `SELECT` statement without a `LIMIT` clause.
Are there performance implications when fetching objects with no limit?
Yes, fetching a large number of objects without limit can lead to performance issues, such as increased memory usage and longer response times. It is advisable to consider pagination or filtering to manage the volume of data retrieved.
What programming languages support fetching objects with no limit?
Most programming languages that interact with databases, such as SQL, Python, Java, and JavaScript, support fetching objects with no limit. The specific implementation may vary based on the database and library used.
Can I apply filters while fetching objects with no limit?
Yes, you can apply filters when fetching objects with no limit. This allows you to retrieve a subset of the data based on specific criteria while still obtaining all matching records.
What are best practices for using “fetch with no limit” in production environments?
Best practices include implementing pagination, using indexing to improve query performance, and monitoring system resources. Additionally, consider limiting the fetch operation to necessary fields to reduce data load.
The concept of Collection.Query.Fetch Objects With No Limit revolves around the ability to retrieve data from a collection without imposing restrictions on the number of records returned. This approach is particularly beneficial when dealing with large datasets where the user requires comprehensive access to all available information. By utilizing this method, developers can streamline data retrieval processes, ensuring that applications can efficiently handle and display extensive collections of objects.
One of the key insights from this discussion is the importance of understanding the implications of fetching unlimited objects. While it may seem advantageous to retrieve all data at once, this practice can lead to performance issues, such as increased memory consumption and slower response times. Therefore, it is crucial for developers to implement appropriate measures, such as pagination or filtering, to optimize data handling and enhance user experience.
Additionally, leveraging the Collection.Query.Fetch method effectively requires a thorough understanding of the underlying data structure and the specific requirements of the application. Developers should assess the context in which this approach is applied, ensuring that it aligns with the overall architecture and performance goals of the system. By doing so, they can harness the full potential of fetching objects without limits while maintaining optimal application performance.
Author Profile
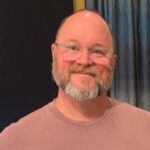
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?