Can You Do It in Python? Exploring the Language’s Versatile Capabilities
Can You Do In Python: Unlocking the Power of Versatility
In the ever-evolving landscape of programming languages, Python stands out as a beacon of versatility and accessibility. Whether you are a seasoned developer or a curious beginner, the question “Can you do it in Python?” resonates with a sense of possibility and exploration. From web development to data science, machine learning to automation, Python has carved a niche that allows users to tackle a myriad of tasks with elegance and efficiency. This article delves into the remarkable capabilities of Python, showcasing its role as a tool that empowers innovation and creativity.
Python’s rich ecosystem of libraries and frameworks makes it a go-to choice for a wide range of applications. Its straightforward syntax and dynamic typing lower the barrier to entry, enabling individuals from diverse backgrounds to harness its potential. As we navigate through the various domains where Python excels, you will discover how its unique features facilitate rapid development and robust solutions. The language’s community-driven approach further enhances its adaptability, ensuring that it continues to evolve in response to the needs of its users.
As we embark on this journey through the versatile world of Python, prepare to be inspired by the endless possibilities that await. From automating mundane tasks to building complex systems, Python equips you with the tools to transform
Data Manipulation
Python excels in data manipulation, making it a preferred language for data scientists and analysts. Libraries such as Pandas and NumPy provide powerful tools for managing and analyzing data.
Pandas allows for easy data manipulation with data frames, enabling operations like filtering, grouping, and merging datasets. Here are some key functionalities:
- Data Cleaning: Handle missing values and remove duplicates.
- Data Transformation: Apply functions to columns or rows.
- Merging and Joining: Combine multiple datasets using different keys.
NumPy, on the other hand, focuses on numerical data, providing array objects for efficient computation. Its capabilities include:
- Vectorization: Perform operations on entire arrays without explicit loops.
- Mathematical Functions: Use built-in functions for statistical calculations.
Web Development
Python is widely used in web development, with frameworks like Flask and Django simplifying the process of building robust web applications.
Django is a high-level web framework that encourages rapid development. It includes:
- Built-in Admin Interface: Manage application data with an easy-to-use interface.
- ORM (Object-Relational Mapping): Interact with databases using Python objects.
- Security Features: Protect against common security threats like SQL injection.
Flask is a micro-framework that provides flexibility and simplicity. Its features include:
- Lightweight: Minimalistic approach allows for easy customization.
- Modular: Use extensions to add functionality as needed.
Framework | Use Case | Key Feature |
---|---|---|
Django | Full-fledged applications | Built-in admin interface |
Flask | Microservices and APIs | Lightweight and modular |
Machine Learning
Python’s rich ecosystem for machine learning includes libraries like scikit-learn, TensorFlow, and Keras. These libraries provide tools for building and deploying machine learning models efficiently.
Scikit-learn offers:
- Supervised Learning: Implement algorithms for classification and regression.
- Unsupervised Learning: Utilize clustering and dimensionality reduction techniques.
- Model Evaluation: Tools for assessing model performance using cross-validation.
TensorFlow and Keras are designed for deep learning applications:
- TensorFlow: A robust platform that supports large-scale neural networks.
- Keras: A high-level API for building and training deep learning models with ease.
Automation and Scripting
Python is particularly strong in automation and scripting tasks, making it invaluable for repetitive tasks and workflow automation. Libraries such as `os`, `shutil`, and `subprocess` facilitate file and process management.
Key automation tasks include:
- File Operations: Create, read, update, and delete files and directories.
- Process Management: Start and stop external applications.
- Web Scraping: Use libraries like Beautiful Soup and Scrapy to extract data from websites.
With Python, users can write scripts that automate mundane tasks, improving efficiency and productivity.
Data Analysis and Visualization
Python excels in data analysis and visualization, making it a preferred choice for data scientists and analysts. Libraries like Pandas, NumPy, and Matplotlib facilitate efficient data manipulation and graphical representation.
– **Pandas**: Provides data structures like DataFrames for handling tabular data.
– **NumPy**: Offers support for large, multi-dimensional arrays and matrices, along with mathematical functions.
– **Matplotlib**: A plotting library for creating static, animated, and interactive visualizations.
**Example of Data Manipulation with Pandas**:
“`python
import pandas as pd
Load dataset
data = pd.read_csv(‘data.csv’)
Data overview
print(data.describe())
Data filtering
filtered_data = data[data[‘column_name’] > 100]
“`
Common Visualization Types:
Visualization Type | Library | Description |
---|---|---|
Line Plot | Matplotlib | Shows trends over time |
Bar Chart | Matplotlib | Compares quantities across categories |
Scatter Plot | Seaborn | Displays relationships between variables |
Web Development
Python is widely used for web development, with frameworks like Flask and Django streamlining the process of building scalable web applications.
- Flask: A lightweight framework that provides simplicity and flexibility.
- Django: A high-level framework that promotes rapid development and clean, pragmatic design.
Basic Flask Application Example:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
Django Project Structure:
Component | Description |
---|---|
Models | Define the data structure |
Views | Handle the logic and user requests |
Templates | HTML files that define the UI |
Machine Learning and AI
Python is the leading language for machine learning and artificial intelligence, aided by libraries such as TensorFlow, Keras, and Scikit-Learn.
- TensorFlow: An open-source framework for large-scale machine learning.
- Keras: A high-level neural networks API, simplifying TensorFlow usage.
- Scikit-Learn: A library for classical machine learning algorithms.
Machine Learning Model Example:
“`python
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
Data preparation
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
Model training
model = RandomForestClassifier()
model.fit(X_train, y_train)
Model evaluation
accuracy = model.score(X_test, y_test)
print(f’Accuracy: {accuracy}’)
“`
Scripting and Automation
Python’s simplicity and readability make it ideal for scripting and automation tasks. It can be used to automate repetitive tasks, manage files, and interact with web services.
- File Handling: Automate file operations like reading, writing, and renaming.
- Web Scraping: Libraries like Beautiful Soup and Scrapy allow for extracting data from web pages.
- Task Scheduling: Use libraries like `schedule` or `APScheduler` to automate tasks at specified intervals.
Example of Web Scraping:
“`python
import requests
from bs4 import BeautifulSoup
Fetch page
response = requests.get(‘https://example.com’)
soup = BeautifulSoup(response.text, ‘html.parser’)
Extract information
titles = [title.text for title in soup.find_all(‘h1’)]
“`
Game Development
Python also finds its applications in game development, with libraries such as Pygame providing the necessary tools to create games.
- Pygame: A set of Python modules designed for writing video games, offering functionalities for graphics and sound.
Basic Pygame Example:
“`python
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running =
pygame.quit()
“`
Scientific Computing
Python serves as a powerful tool for scientific computing, supported by libraries like SciPy and SymPy, which facilitate complex calculations and symbolic mathematics.
- SciPy: Builds on NumPy and provides additional functionality for optimization, integration, and statistics.
- SymPy: A library for symbolic mathematics that allows for algebraic manipulations and calculus.
Example of Numerical Integration:
“`python
from scipy.integrate import quad
Define function
def f(x):
return x**2
Compute integral
result, error = quad(f, 0, 1)
print(f’Integral result: {result}’)
“`
Exploring the Versatility of Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Corp). “Python’s extensive libraries and frameworks make it an ideal choice for data analysis, machine learning, and artificial intelligence. Its simplicity allows data scientists to focus on solving complex problems rather than grappling with intricate syntax.”
Michael Chen (Lead Software Engineer, FutureTech Solutions). “In web development, Python’s frameworks like Django and Flask enable rapid application development. This efficiency, combined with Python’s scalability, ensures that applications can grow alongside user demands without requiring a complete rewrite.”
Sarah Patel (Cybersecurity Analyst, SecureNet). “Python is increasingly being utilized in cybersecurity for automating tasks such as network scanning and vulnerability assessment. Its versatility allows security professionals to develop scripts that can adapt to various environments and threats.”
Frequently Asked Questions (FAQs)
Can you do data analysis in Python?
Yes, Python is widely used for data analysis due to its powerful libraries such as Pandas, NumPy, and Matplotlib, which facilitate data manipulation, statistical analysis, and visualization.
Can you automate tasks in Python?
Yes, Python is an excellent choice for automation. Libraries like Selenium for web automation and PyAutoGUI for GUI automation allow users to automate repetitive tasks effectively.
Can you develop web applications in Python?
Yes, Python supports web development through frameworks such as Django and Flask, enabling developers to create robust web applications efficiently.
Can you perform machine learning in Python?
Yes, Python is a leading language for machine learning, with libraries like Scikit-learn, TensorFlow, and Keras providing tools for building and deploying machine learning models.
Can you create games in Python?
Yes, Python can be used to create games using libraries like Pygame, which provides functionalities for game development, including graphics, sound, and user input handling.
Can you work with databases in Python?
Yes, Python can interact with various databases using libraries such as SQLAlchemy and SQLite3, allowing for seamless database management and data manipulation.
In summary, Python is a versatile programming language that enables users to perform a wide array of tasks across various domains. Its simplicity and readability make it an excellent choice for beginners, while its powerful libraries and frameworks cater to advanced users. From web development and data analysis to artificial intelligence and automation, Python’s capabilities are extensive, allowing developers to create complex applications with relative ease.
Moreover, Python’s strong community support and extensive documentation provide invaluable resources for both novice and experienced programmers. The language’s compatibility with numerous platforms and its ability to integrate with other languages further enhance its utility. As a result, Python continues to be a leading choice for developers looking to build innovative solutions in today’s fast-paced technological landscape.
Key takeaways from the discussion around what you can do in Python include the importance of leveraging its libraries, such as NumPy for numerical computations, Pandas for data manipulation, and TensorFlow for machine learning. Additionally, understanding Python’s role in web frameworks like Django and Flask can significantly enhance one’s ability to develop robust web applications. Overall, Python’s flexibility and extensive ecosystem make it a powerful tool for a wide range of programming needs.
Author Profile
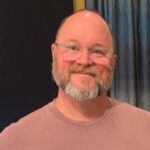
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?