Why Am I Encountering Java Lang OutOfMemoryError: GC Overhead Limit Exceeded?
### Introduction
In the world of Java programming, encountering errors can be a frustrating yet enlightening experience, especially when it comes to memory management. One of the most notorious issues developers face is the `java.lang.OutOfMemoryError: GC overhead limit exceeded`. This error not only signals a significant problem within your application but also serves as a wake-up call to reevaluate how memory is allocated and utilized in your Java environment. Understanding this error is crucial for any developer looking to optimize their applications and ensure smooth performance.
The `OutOfMemoryError: GC overhead limit exceeded` occurs when the Java Virtual Machine (JVM) spends an excessive amount of time performing garbage collection while recovering very little memory. This situation typically arises when the heap space is insufficient for the application’s demands, leading to frequent garbage collection cycles that hinder performance. As the JVM struggles to reclaim memory, it can result in sluggish application behavior, increased latency, and ultimately, application crashes if not addressed promptly.
Navigating the complexities of this error requires a blend of technical insight and practical strategies. Developers must delve into memory profiling, heap analysis, and garbage collection tuning to identify the root causes and implement effective solutions. By understanding the intricacies of memory management in Java, you can not only resolve the `GC overhead limit exceeded
Understanding GC Overhead Limit Exceeded
The `java.lang.OutOfMemoryError: GC overhead limit exceeded` error is a common issue faced by Java developers, particularly when dealing with large data sets or poorly optimized applications. This error occurs when the Java Virtual Machine (JVM) spends excessive time performing garbage collection (GC) without successfully reclaiming sufficient memory. Specifically, the JVM has a built-in threshold that, when exceeded, triggers this error to prevent applications from hanging indefinitely due to memory issues.
When the GC overhead limit is exceeded, the JVM essentially indicates that it is spending more than 98% of its total time in garbage collection and that it is recovering less than 2% of the heap memory. This scenario often leads to application performance degradation, making it critical to understand and address the underlying causes.
Common Causes of the Error
Several factors can contribute to the `GC overhead limit exceeded` error:
- Memory Leaks: Unintentional retention of objects that are no longer needed can lead to increased memory consumption.
- Insufficient Heap Space: The allocated heap space might not be enough for the application’s requirements, leading to frequent garbage collection cycles.
- Large Object Allocation: Allocating large objects or collections can put pressure on the heap, triggering excessive garbage collection.
- High Object Creation Rate: Rapid creation and destruction of objects can overwhelm the garbage collector, making it unable to keep up.
- Inefficient Garbage Collection Strategy: Using an unsuitable garbage collection algorithm for the application’s behavior can lead to performance issues.
Diagnosing the Issue
To diagnose the `GC overhead limit exceeded` error, consider the following steps:
- Monitor Memory Usage: Use tools like Java VisualVM, JConsole, or other profiling tools to monitor heap usage and garbage collection behavior.
- Analyze Heap Dumps: Generate heap dumps during the error occurrence to analyze object retention and identify memory leaks.
- Review GC Logs: Enable GC logging to understand the frequency and duration of garbage collection events. The following JVM options can be used:
-XX:+PrintGCDetails
-XX:+PrintGCDateStamps
-Xloggc:gc.log
- Examine Application Code: Look for patterns in the code that may lead to high object creation or retention.
Preventing and Resolving the Error
To prevent the `GC overhead limit exceeded` error, various strategies can be employed:
- Increase Heap Size: Adjust the heap size using JVM options to provide more memory for the application. Example:
-Xms512m -Xmx2048m
- Optimize Code: Refactor code to reduce object creation and ensure that unnecessary references are cleared.
- Use Efficient Data Structures: Choose appropriate data structures that minimize memory overhead.
- Adjust Garbage Collection Settings: Depending on the application requirements, experiment with different garbage collection strategies (e.g., G1, CMS, ZGC) to find the most effective one.
Garbage Collection Settings Comparison
The following table summarizes different garbage collection algorithms and their ideal use cases:
Garbage Collector | Use Case | Pros | Cons |
---|---|---|---|
Serial GC | Single-threaded applications | Simple and low overhead | Poor performance on multi-core systems |
Parallel GC | Batch processing | Improved throughput | Long pause times |
Concurrent Mark-Sweep (CMS) | Low-latency applications | Shorter pause times | Fragmentation issues |
G1 GC | Large heap sizes | Predictable pause times | Complex tuning |
ZGC | Low-latency applications | Very short pause times | Experimental and not available in all JVMs |
Understanding the OutOfMemoryError
The `OutOfMemoryError` in Java signifies that the Java Virtual Machine (JVM) has exhausted its heap memory space. This error is particularly critical as it can lead to application crashes or degraded performance.
- Types of OutOfMemoryError:
- Java heap space: Indicates that the heap memory is full.
- GC overhead limit exceeded: Occurs when the garbage collector spends too much time trying to reclaim memory but recovers very little.
- PermGen space (Java 7 and earlier): Related to the memory allocated for class definitions and metadata.
- Metaspace (Java 8 and later): Refers to the space used for class metadata.
GC Overhead Limit Exceeded
The `GC overhead limit exceeded` error suggests that the garbage collector is running excessively but failing to free up sufficient memory. By default, if more than 98% of the total time is spent in garbage collection and less than 2% of the heap is recovered, this error is triggered.
- Common Causes:
- Memory leaks due to unintentional object retention.
- Inadequate heap size for the application’s requirements.
- High object churn rate leading to frequent garbage collection cycles.
Identifying the Problem
To effectively address the `OutOfMemoryError`, diagnostics are essential. Key strategies include:
- Heap Dumps: Capture heap dumps when the error occurs to analyze memory usage.
- Profiling Tools: Utilize profiling tools like VisualVM or JProfiler to monitor memory consumption and identify leaks.
- Garbage Collection Logs: Enable GC logging to understand the behavior of the garbage collector.
Tool | Purpose |
---|---|
VisualVM | Monitor memory and CPU usage |
JProfiler | Detailed memory analysis |
YourKit | Profiling and memory leak detection |
Solutions and Best Practices
When faced with the `GC overhead limit exceeded` error, several approaches can help mitigate the issue.
- Increase Heap Size:
- Modify JVM options to allocate more memory:
bash
-Xmx2g # Sets maximum heap size to 2GB
- Optimize Code:
- Reduce object creation frequency.
- Use data structures that are memory efficient.
- Implement proper caching strategies.
- Garbage Collection Tuning:
- Adjust garbage collector settings based on application needs.
- Consider using different garbage collectors (G1, CMS) depending on the application’s characteristics.
- Memory Leak Detection:
- Regularly review code for potential memory leaks.
- Utilize tools such as Eclipse Memory Analyzer (MAT) to analyze heap dumps for unreachable objects.
Recommendations
Applying a combination of these strategies can significantly enhance application stability and performance, reducing the likelihood of encountering the `OutOfMemoryError: GC overhead limit exceeded`. Regular monitoring and proactive management of memory usage are crucial for maintaining a healthy Java application environment.
Expert Insights on Java Lang OutOfMemoryError: GC Overhead Limit Exceeded
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘GC Overhead Limit Exceeded’ error typically indicates that the Java Virtual Machine (JVM) is spending too much time in garbage collection while being unable to reclaim enough memory. This often points to memory leaks or insufficient heap size, necessitating a thorough review of the application’s memory management practices.”
Michael Chen (Lead Java Developer, Cloud Solutions Corp.). “When encountering the ‘OutOfMemoryError: GC overhead limit exceeded’, it is crucial to analyze the heap dump and identify objects that are consuming excessive memory. Tools like VisualVM or Eclipse Memory Analyzer can provide insights into memory usage patterns, allowing for targeted optimizations.”
Sarah Johnson (Java Performance Specialist, OptimizeIT). “Preventing the ‘GC Overhead Limit Exceeded’ error involves both proactive and reactive strategies. Implementing proper caching strategies and optimizing data structures can significantly reduce memory consumption. Additionally, setting appropriate JVM flags for garbage collection can help manage memory more effectively.”
Frequently Asked Questions (FAQs)
What does Java Lang OutOfMemoryError: GC overhead limit exceeded mean?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) and recovering very little memory. It typically occurs when the application is running low on heap space.
What causes the GC overhead limit exceeded error?
The error is often caused by insufficient heap memory allocated to the JVM, memory leaks in the application, or inefficient memory usage patterns that lead to excessive garbage collection cycles.
How can I resolve the GC overhead limit exceeded error?
To resolve this issue, consider increasing the heap size allocated to the JVM using the `-Xmx` option, optimizing the application code to reduce memory consumption, or identifying and fixing memory leaks.
What are the JVM options to increase heap memory?
You can increase the maximum heap size by using the `-Xmx` option, for example, `-Xmx2g` for 2 gigabytes. Additionally, you can set the initial heap size with the `-Xms` option.
How can I monitor memory usage in a Java application?
You can monitor memory usage using tools like Java VisualVM, JConsole, or by enabling GC logging with the `-Xloggc:
Are there any best practices to avoid GC overhead limit exceeded errors?
Yes, best practices include profiling the application to identify memory usage patterns, optimizing data structures, avoiding large object allocations, and regularly reviewing and refactoring code to improve memory efficiency.
The Java `OutOfMemoryError: GC overhead limit exceeded` error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) without reclaiming enough memory. This situation typically arises when the application is running low on heap space, leading the JVM to repeatedly attempt to free up memory but ultimately failing to do so effectively. The error serves as a critical warning that the application is struggling to manage its memory resources, which can significantly impact performance and stability.
Several factors can contribute to this error, including memory leaks, inadequate heap size configuration, or inefficient memory usage patterns in the application. Developers should investigate the memory allocation and usage within their applications to identify potential bottlenecks. Tools such as profilers and heap dump analyzers can assist in diagnosing memory issues, allowing developers to pinpoint the root causes of excessive memory consumption and optimize their code accordingly.
To mitigate the risk of encountering this error, it is essential to monitor the application’s memory usage closely and adjust the JVM’s heap size settings as needed. Increasing the heap size can provide more memory for the application to utilize, potentially alleviating the pressure on the garbage collector. Additionally, implementing best practices for memory management, such as avoiding unnecessary object creation and
Author Profile
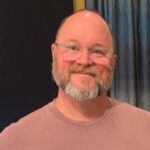
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?