What Is ‘I’ in Python: Understanding Its Role and Usage?
What Is I In Python: Understanding the Power of Iteration
In the world of programming, the letter “I” often stands as a symbol of iteration, a fundamental concept that drives many algorithms and processes. Python, renowned for its simplicity and elegance, utilizes iteration in a variety of ways, making it a crucial aspect for developers to grasp. Whether you’re a seasoned coder or just starting your journey, understanding how “I” operates within Python can significantly enhance your coding efficiency and effectiveness. This article will delve into the nuances of iteration in Python, exploring its applications, best practices, and the powerful tools the language offers.
At its core, iteration in Python allows developers to traverse through data structures, execute repetitive tasks, and manage sequences with ease. The letter “I” often represents an index or iterator in loops, playing a pivotal role in controlling the flow of data. From traditional `for` and `while` loops to the more advanced generator expressions, Python provides a rich set of features that empower programmers to write clean and efficient code. Understanding how to leverage these iteration techniques can lead to more elegant solutions and improved performance in your applications.
As we explore the intricacies of iteration in Python, we will uncover the various constructs available, their syntax, and the scenarios in which
Understanding the ‘I’ in Python
In Python, ‘I’ commonly refers to the imaginary unit in complex numbers. Python provides support for complex numbers natively, allowing you to perform mathematical operations involving imaginary numbers seamlessly. The imaginary unit is represented as ‘j’ in Python, but when discussing mathematics, it is often referred to as ‘i’.
Complex numbers in Python are written in the form of `a + bj`, where `a` is the real part and `b` is the imaginary part. For example, `3 + 4j` represents a complex number where `3` is the real part and `4` is the imaginary part.
Creating Complex Numbers
To create complex numbers in Python, you can directly define them using the `complex()` function or by using the `j` suffix. Here are a couple of methods:
- Using the `complex()` function:
“`python
z1 = complex(3, 4) Creates a complex number 3 + 4j
“`
- Using the `j` suffix:
“`python
z2 = 3 + 4j Creates the same complex number
“`
Both methods yield the same result, and you can use either depending on your preference.
Operations on Complex Numbers
Python allows various operations on complex numbers, including addition, subtraction, multiplication, and division. Here are some examples:
“`python
z1 = 3 + 4j
z2 = 1 + 2j
Addition
result_add = z1 + z2 (3 + 4j) + (1 + 2j) = 4 + 6j
Subtraction
result_sub = z1 – z2 (3 + 4j) – (1 + 2j) = 2 + 2j
Multiplication
result_mul = z1 * z2 (3 + 4j) * (1 + 2j) = 3 + 10j – 8 = -5 + 10j
Division
result_div = z1 / z2 (3 + 4j) / (1 + 2j) = (3*1 + 4*2) / (1*1 + 2*2) + ((4*1 – 3*2) / (1*1 + 2*2))j = 2.0 – 1.0j
“`
Properties of Complex Numbers
Complex numbers have unique properties that are often used in advanced mathematical computations. Here are some key properties:
- Magnitude: The magnitude of a complex number `z = a + bj` is calculated as:
\[
z | = \sqrt{a^2 + b^2} |
---|
\]
- Conjugate: The conjugate of a complex number `z` is given by `z_conjugate = a – bj`. This is useful in division and finding the magnitude.
Table of Complex Number Functions in Python
Function | Description |
---|---|
real | Returns the real part of a complex number. |
imag | Returns the imaginary part of a complex number. |
conjugate() | Returns the conjugate of a complex number. |
abs() | Returns the magnitude of the complex number. |
By leveraging these features, you can efficiently work with complex numbers in Python for various applications, including engineering, physics, and computer science.
Understanding the `I` in Python
In the context of Python, the letter `I` does not denote a specific feature or function within the language. However, it can refer to several concepts or libraries where `I` might be part of an identifier, a variable name, or a term used in programming paradigms. Below are some relevant interpretations of `I` in Python programming.
Common Uses of `I` in Python
- Variable Naming: In many coding conventions, `i` is often used as a variable name in loops and iterations. This practice stems from its use in mathematics and programming to represent an index or an integer.
- Complex Numbers: In Python, complex numbers can be expressed using `j` instead of `i`, which is common in mathematics. However, when discussing complex numbers in terms of electrical engineering, `I` may refer to the imaginary unit.
- In Libraries: Certain libraries use `I` in their naming conventions or as part of their functions. For instance:
- NumPy: While `numpy` primarily uses `j` for imaginary parts, you may see `I` in discussions about complex number representation.
- Pandas: In data manipulation, `I` could be a column name or a prefix in DataFrame manipulations.
Example: Using `i` as an Iteration Variable
A common Python snippet that utilizes `i` as an iteration variable is as follows:
“`python
for i in range(5):
print(i)
“`
This code block outputs:
“`
0
1
2
3
4
“`
Here, `i` serves as an index in the loop, showcasing its conventional use in programming.
Comparison of Variable Naming Conventions
Variable Name | Typical Usage | Context |
---|---|---|
`i` | Loop index | Iteration |
`j` | Nested loop index | Iteration |
`I` | Imaginary unit (in theory) | Complex numbers (rarely) |
Conclusion on `I` in Python Contexts
Although `I` does not have a specific, universally accepted meaning in Python, understanding its common usages helps clarify its role in programming. It is crucial to remain aware of context when encountering this variable, as it may vary significantly across different applications and libraries.
Understanding the Role of ‘I’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, ‘i’ is commonly used as a variable name, especially in loops. It serves as a conventional placeholder for an integer index, enhancing code readability and maintaining consistency across various programming practices.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “The use of ‘i’ in Python is not just limited to loops; it also appears in list comprehensions and generator expressions. This simplicity allows developers to focus on the logic of their code without getting bogged down by complex variable names.”
Sarah Thompson (Python Educator, LearnPython.org). “While ‘i’ is a traditional choice for iteration, it is essential to choose variable names that convey meaning. Although ‘i’ is widely accepted, clarity in naming can significantly improve the maintainability of Python code, especially in larger projects.”
Frequently Asked Questions (FAQs)
What is ‘I’ in Python?
‘I’ typically refers to a variable name or an iterator in Python. It is commonly used in loops and comprehensions to represent the current index or element being processed.
Is ‘I’ a reserved keyword in Python?
No, ‘I’ is not a reserved keyword in Python. It can be used as a variable name without any restrictions, although using descriptive names is recommended for code clarity.
What does ‘I’ represent in list comprehensions?
In list comprehensions, ‘I’ often serves as a loop variable that iterates over elements of a sequence, allowing for the creation of new lists based on existing data.
Can ‘I’ be used as an identifier in Python functions?
Yes, ‘I’ can be used as an identifier in functions. However, it is advisable to use more descriptive names to enhance code readability and maintainability.
How does ‘I’ work in for loops in Python?
In for loops, ‘I’ can be defined as the loop variable, which takes on the value of each element in the iterable on each iteration, enabling operations on those elements.
Is there a specific convention for naming variables like ‘I’ in Python?
While there is no strict rule, the convention in Python suggests using lowercase letters for variable names, such as ‘i’, especially for loop counters, to adhere to PEP 8 style guidelines.
In Python, the keyword `I` does not have a specific meaning or function. However, it is often used as a variable name, particularly in loops or when iterating through data structures. Python is case-sensitive, so `I` is distinct from `i`, which is commonly used as an index in iterations. Understanding the context in which `I` is used is crucial for interpreting its purpose, as it can vary based on the programmer’s intent.
Moreover, Python’s flexibility allows developers to choose variable names that are meaningful within the context of their code. While `I` might be utilized in some examples or tutorials, it is generally advisable to use descriptive variable names that enhance code readability and maintainability. This practice supports better collaboration among developers and aids in the long-term management of codebases.
In summary, while `I` itself does not represent a built-in function or keyword in Python, its usage as a variable name is prevalent. Developers should prioritize clarity and context when naming variables, ensuring that their code remains understandable and effective for anyone who may work with it in the future. By adhering to these principles, programmers can contribute to a more robust coding environment.
Author Profile
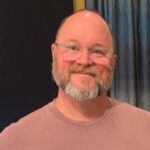
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?