Why Am I Seeing ‘Invalid Character’ and ‘Looking For Beginning Of Value’ Errors in My Code?
In the world of programming and data processing, encountering errors can be a frustrating yet enlightening experience. One such error that developers and data analysts often face is the cryptic message: “Invalid Character ‘ ‘ Looking For Beginning Of Value.” This seemingly innocuous phrase can halt progress and lead to hours of debugging, but understanding its implications is crucial for anyone working with data formats like JSON or XML. As we delve into the intricacies of this error, we will uncover the common pitfalls that lead to its emergence, the contexts in which it typically occurs, and the strategies you can employ to troubleshoot and resolve it effectively.
When dealing with data parsing, the structure and format of the input are paramount. An “Invalid Character” error often indicates that the parser has stumbled upon an unexpected character, in this case, a space, at a critical juncture in the data stream. This can happen for various reasons, such as improper formatting, misplaced punctuation, or even the presence of extraneous whitespace. Understanding the nature of this error is essential for developers who wish to maintain clean, efficient code and ensure that their applications can handle data robustly.
Moreover, the implications of this error extend beyond mere annoyance; they can affect the integrity of data processing workflows and application performance. By recognizing
Understanding JSON Parsing Errors
When working with JSON (JavaScript Object Notation), developers may encounter various parsing errors that can disrupt the functionality of applications. One common error is the “Invalid Character ‘ ‘ Looking For Beginning Of Value.” This message often indicates that there is an unexpected space or whitespace character at the beginning of a JSON value, leading to parsing failures.
To diagnose and fix this error, consider the following approaches:
- Check for Whitespace: Ensure there are no leading or trailing spaces in your JSON data. These can inadvertently be added during string manipulation or file reading.
- Validate JSON Format: Use online JSON validators to check the syntax of your JSON. These tools can help pinpoint the exact location of the error.
- Debugging Logs: Implement logging to capture the JSON data being processed. This can help identify whether the data is malformed before it reaches the parsing stage.
Common Causes of the Error
Several factors can lead to the “Invalid Character” error, including:
- Manual Input Errors: Typographical errors during manual JSON creation can introduce unintended characters.
- File Encoding Issues: Different file encodings (such as UTF-8 vs. UTF-16) may introduce invisible characters that affect parsing.
- Improper Data Handling: When dynamically generating JSON, ensure that all data types are correctly formatted and stringified.
Cause | Description |
---|---|
Whitespace | Leading or trailing spaces in JSON strings or values. |
Encoding | Mismatched character encoding leading to unrecognized characters. |
Data Types | Improperly formatted data types (e.g., numbers as strings). |
Best Practices for Avoiding JSON Parsing Errors
To minimize the occurrence of JSON parsing errors, developers should adhere to several best practices:
- Use Libraries: Utilize well-established libraries for JSON serialization and deserialization, which typically handle many edge cases that may lead to errors.
- Consistent Formatting: Maintain consistent formatting throughout your JSON data to ensure clarity and reduce the likelihood of errors.
- Automated Testing: Implement automated tests that validate JSON data structures before processing them in production environments.
By proactively addressing potential issues and adhering to best practices, developers can streamline the JSON parsing process and reduce the incidence of errors related to invalid characters.
Understanding the Error
The error message `Invalid Character ‘ ‘ Looking For Beginning Of Value` typically arises in programming contexts, particularly when dealing with JSON data. This error indicates that the JSON parser has encountered an unexpected space character where it anticipated a valid starting character for a value, such as a string, number, object, or array.
- Common Causes:
- Leading or trailing whitespace in JSON data.
- Incorrectly formatted JSON strings.
- Misplaced commas or brackets that disrupt the JSON structure.
- Accidental inclusion of characters not permitted in JSON, such as comments or invalid escape sequences.
To effectively address this error, it is crucial to thoroughly validate the JSON structure being parsed.
Identifying the Source of the Error
To pinpoint where the error originates, consider the following steps:
- Check JSON Syntax:
- Use a JSON validator tool to verify the syntax. These tools highlight errors and provide line numbers for quick identification.
- Examine Input Data:
- Review the raw data being parsed. Look for spaces, especially at the beginning or end of strings or objects.
- Log the Raw Input:
- Implement logging to capture the exact JSON string being processed. This can help identify any formatting issues that may not be immediately visible.
- Debugging Tools:
- Utilize debugging tools or IDE features that allow step-by-step execution to observe when the error occurs.
Resolving the Error
Once the source of the error has been identified, follow these guidelines to resolve it:
- Trim Whitespace:
- Ensure that all strings are trimmed of leading and trailing spaces. This can often be accomplished with built-in string functions in most programming languages.
- Correct Formatting:
- Ensure that your JSON follows the correct structure:
- Strings should be enclosed in double quotes.
- Objects are defined with curly braces `{}`.
- Arrays are defined with square brackets `[]`.
- Escape Characters:
- Ensure that any special characters within strings are properly escaped. For instance, use `\”` for quotes within strings.
- Example of a Well-Formatted JSON:
“`json
{
“name”: “John Doe”,
“age”: 30,
“isEmployed”: true,
“skills”: [“JavaScript”, “Python”, “Java”]
}
“`
Tools for Validation
Several tools can help validate and format JSON to avoid such errors:
Tool | Description |
---|---|
JSONLint | A web-based JSON validator and formatter. |
jq | A command-line JSON processor. |
Postman | A tool for API testing that validates JSON responses. |
Online JSON Viewer | A browser-based viewer that formats and validates JSON. |
Using these tools can significantly enhance the development process by catching errors early and ensuring clean data handling.
Understanding the ‘Invalid Character’ Error in Data Parsing
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The ‘Invalid Character’ error often arises when a parser encounters unexpected whitespace or special characters in a data stream. It is crucial to sanitize input data before processing to avoid such issues.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “When dealing with JSON or XML data, the presence of an invalid character like a space can disrupt the entire parsing process. Implementing robust error handling and validation mechanisms can significantly mitigate these risks.”
Linda Garcia (Technical Support Specialist, DataFix Corp.). “Users often overlook the importance of encoding formats. An ‘Invalid Character’ error can stem from mismatched encoding, so ensuring consistency across data sources is essential for smooth data processing.”
Frequently Asked Questions (FAQs)
What does the error “Invalid Character ‘ ‘ Looking For Beginning Of Value” mean?
This error typically indicates that there is an unexpected space character in a JSON input, which prevents the parser from correctly interpreting the data structure.
What causes this error in JSON files?
The error is usually caused by improperly formatted JSON, such as leading or trailing spaces, or incorrect placement of commas and braces, which disrupts the expected syntax.
How can I fix the “Invalid Character ‘ ‘ Looking For Beginning Of Value” error?
To resolve this error, review the JSON structure for any extraneous spaces, ensure proper formatting, and validate the JSON using a JSON validator tool to identify syntax issues.
Is this error specific to certain programming languages?
While this error is commonly associated with languages that utilize JSON, such as JavaScript and Python, it can occur in any environment that processes JSON data if the input is malformed.
Can this error occur in APIs?
Yes, this error can occur when an API receives malformed JSON data in a request or response, leading to parsing failures and resulting in error messages.
What tools can help identify this error in JSON data?
Tools such as JSONLint, Postman, or built-in JSON parsers in programming languages can help identify and correct formatting issues that may lead to this error.
The error message “Invalid Character ‘ ‘ Looking For Beginning Of Value” typically indicates that there is an issue with the format of data being processed, often in the context of JSON parsing. This error arises when a parser encounters an unexpected space or character that disrupts the expected structure of the data. It is crucial to ensure that the data being inputted adheres to the correct syntax, as improper formatting can lead to significant issues in data handling and processing.
One of the main points to consider is the importance of validating data before it is parsed. Implementing thorough validation checks can help catch formatting errors, such as leading or trailing spaces, which may not be immediately visible. Additionally, using tools or libraries designed for JSON validation can streamline the debugging process, allowing developers to identify and rectify issues more efficiently.
Another key takeaway is the necessity of maintaining consistent data formats across applications. When data is exchanged between different systems, discrepancies in formatting can lead to errors like the one mentioned. Establishing clear guidelines for data formatting and ensuring that all systems adhere to these standards can mitigate the risk of encountering such errors in the future.
addressing the “Invalid Character ‘ ‘ Looking For Beginning Of Value” error requires a proactive approach
Author Profile
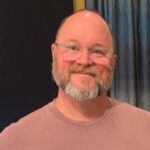
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?