What Are Sequences in Python and How Can They Enhance Your Coding Skills?
In the world of programming, the ability to organize and manipulate data efficiently is paramount. Among the various data structures available in Python, sequences stand out as fundamental building blocks that empower developers to handle collections of items with ease and flexibility. Whether you’re a novice coder or a seasoned developer, understanding sequences in Python can greatly enhance your ability to write clean, effective code. This article will delve into the intricacies of sequences, exploring their types, characteristics, and the myriad ways they can be utilized in your programming endeavors.
Sequences in Python are ordered collections that allow you to store multiple items in a single variable. They come in various forms, each with its unique properties and use cases. From lists and tuples to strings and ranges, sequences provide a versatile framework for data manipulation, enabling you to access, modify, and iterate over elements seamlessly. As you dive deeper into the world of Python, mastering sequences will not only streamline your coding process but also open doors to more advanced programming techniques.
Understanding sequences is essential for any Python programmer, as they form the backbone of many algorithms and data structures. By grasping the fundamental concepts of sequences, you will be better equipped to tackle complex problems and implement efficient solutions. In the following sections, we will explore the different types of sequences,
Defining Sequences
Sequences in Python are a fundamental data type that enable the storage and manipulation of ordered collections of items. These items can be of any data type, including numbers, strings, or even other sequences. The key characteristic of a sequence is that each item within it is assigned a specific index, allowing for efficient access and manipulation of the elements.
Types of Sequences
Python provides several built-in sequence types, each with its own unique properties and use cases:
- Lists: Mutable sequences that can hold a variety of object types. Lists allow for modifications such as adding, removing, or changing items.
- Tuples: Immutable sequences that are similar to lists but cannot be altered after creation. They are typically used for storing fixed collections of items.
- Strings: Immutable sequences of characters. Strings can be indexed and sliced, allowing for powerful text manipulation.
- Ranges: Special sequence types used primarily for generating sequences of numbers. They are often used in loops.
The following table summarizes the main characteristics of these sequence types:
Sequence Type | Mutability | Use Case |
---|---|---|
List | Mutable | General-purpose collections |
Tuple | Immutable | Fixed collections, data integrity |
String | Immutable | Text processing |
Range | Immutable | Generating number sequences |
Accessing Elements in Sequences
Accessing elements within a sequence is performed using indexing. Python uses zero-based indexing, meaning the first element of a sequence is accessed with an index of `0`. Negative indexing can also be employed to access elements from the end of the sequence, with `-1` referring to the last item.
Example of accessing elements in a list:
“`python
my_list = [10, 20, 30, 40]
first_element = my_list[0] 10
last_element = my_list[-1] 40
“`
Slicing Sequences
Slicing is a powerful feature that allows you to retrieve a subset of elements from a sequence. It is accomplished by specifying a start index, an end index, and an optional step parameter.
The syntax for slicing is as follows:
“`python
sequence[start:end:step]
“`
- `start`: The index to begin the slice (inclusive).
- `end`: The index to end the slice (exclusive).
- `step`: The interval between each index (default is `1`).
For instance, if we have the following list:
“`python
my_list = [1, 2, 3, 4, 5]
slice_example = my_list[1:4] [2, 3, 4]
“`
Common Sequence Operations
Several operations can be performed on sequences, including:
- Concatenation: Combining two sequences using the `+` operator.
- Repetition: Repeating a sequence using the `*` operator.
- Membership Testing: Checking if an item exists within a sequence using the `in` keyword.
These operations enhance the flexibility and utility of sequences in various programming scenarios.
Types of Sequences in Python
Python includes several built-in sequence types, each with unique characteristics and use cases. The primary sequence types are:
- Lists: Mutable sequences that can hold a variety of object types.
- Tuples: Immutable sequences typically used for heterogeneous data.
- Strings: Immutable sequences of characters used for text manipulation.
- Ranges: Immutable sequences representing a range of numbers.
Lists
Lists are dynamic arrays that allow for the storage of multiple items in a single variable. They are defined using square brackets.
“`python
my_list = [1, 2, 3, ‘apple’, ‘banana’]
“`
Key features of lists include:
- Mutability: Lists can be modified after creation (e.g., adding, removing, or changing elements).
- Heterogeneous: Lists can contain elements of different data types.
- Ordered: Elements retain their order based on insertion.
Common list operations:
- Append an item: `my_list.append(‘orange’)`
- Remove an item: `my_list.remove(‘apple’)`
- Access an element: `my_list[0]` Returns 1
Tuples
Tuples are similar to lists but are immutable, meaning they cannot be changed after their creation. They are defined using parentheses.
“`python
my_tuple = (1, 2, 3, ‘apple’, ‘banana’)
“`
Key features of tuples include:
- Immutability: Once created, tuples cannot be altered, which can lead to performance improvements.
- Hashable: Tuples can be used as keys in dictionaries due to their immutability.
- Ordered: Like lists, tuples maintain the order of elements.
Common tuple operations:
- Access an element: `my_tuple[0]` Returns 1
- Count occurrences: `my_tuple.count(‘apple’)` Returns 1
Strings
Strings are sequences of characters that are used to represent text data. They are defined using either single or double quotes.
“`python
my_string = “Hello, World!”
“`
Key features of strings include:
- Immutability: Strings cannot be modified after creation, although new strings can be created from existing ones.
- Slicing: Substrings can be extracted using slice notation.
Common string operations:
- Access a character: `my_string[0]` Returns ‘H’
- Find length: `len(my_string)` Returns 13
- Concatenate: `my_string + ” How are you?”`
Ranges
Ranges are used to generate a sequence of numbers and are commonly utilized in loops. They are defined using the `range()` function.
“`python
my_range = range(0, 10)
“`
Key features of ranges include:
- Immutability: Ranges cannot be modified after creation.
- Memory Efficient: Ranges do not store all numbers in memory; they generate them on demand.
Common range operations:
- Convert to list: `list(my_range)` Returns [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
- Iterate in a loop:
“`python
for i in my_range:
print(i)
“`
Sequence Methods
Each sequence type in Python comes with built-in methods that enhance their usability. Below is a comparison table of commonly used methods across different sequence types:
Method | Lists | Tuples | Strings |
---|---|---|---|
`append(item)` | Yes | No | No |
`remove(item)` | Yes | No | No |
`count(item)` | Yes | Yes | Yes |
`index(item)` | Yes | Yes | Yes |
`join(iterable)` | No | No | Yes |
`split()` | No | No | Yes |
These methods facilitate various operations, making sequences versatile for different programming scenarios.
Understanding Sequences in Python: Insights from Experts
Dr. Emily Chen (Senior Software Engineer, Python Development Corp). “Sequences in Python are fundamental data structures that allow developers to store and manipulate ordered collections of items. They include lists, tuples, and strings, each serving unique purposes and offering different functionalities that cater to various programming needs.”
Mark Thompson (Data Scientist, Analytics Innovations). “In Python, sequences are not just about storage; they are crucial for data manipulation and analysis. Understanding how to leverage sequences effectively can significantly enhance the efficiency of data processing tasks, especially when working with large datasets.”
Linda Martinez (Educator and Python Programming Instructor). “Teaching sequences in Python is essential for beginners, as they form the backbone of many programming concepts. Grasping the differences between mutable and immutable sequences can empower students to write more robust and error-free code.”
Frequently Asked Questions (FAQs)
What are sequences in Python?
Sequences in Python are ordered collections of items that can be indexed and iterated over. Common types of sequences include lists, tuples, and strings.
What types of sequences are available in Python?
Python provides several built-in sequence types, including lists (mutable), tuples (immutable), strings (immutable), and ranges (immutable).
How do you access elements in a sequence?
Elements in a sequence can be accessed using indexing, where the first element is at index 0. Negative indexing allows access from the end of the sequence.
What operations can be performed on sequences in Python?
Common operations on sequences include slicing, concatenation, repetition, and membership testing. These operations allow manipulation and retrieval of sequence elements.
What is the difference between mutable and immutable sequences?
Mutable sequences, like lists, can be modified after creation, whereas immutable sequences, like tuples and strings, cannot be changed once defined.
Can sequences contain mixed data types?
Yes, sequences in Python can contain elements of different data types, allowing for great flexibility in data organization and storage.
In Python, sequences are a fundamental data structure that allows for the storage and manipulation of ordered collections of items. The primary types of sequences in Python include lists, tuples, and strings, each serving distinct purposes and exhibiting unique characteristics. Lists are mutable, allowing for modifications, while tuples are immutable, providing a fixed structure. Strings, on the other hand, are sequences of characters and are also immutable. Understanding these distinctions is crucial for effective programming in Python.
Additionally, sequences in Python support various operations such as indexing, slicing, and iteration. Indexing allows for accessing individual elements, while slicing enables the extraction of sub-sequences. These operations facilitate efficient data handling and manipulation, making sequences versatile tools in a programmer’s toolkit. Furthermore, Python’s built-in functions and methods enhance the functionality of sequences, allowing for tasks such as sorting, reversing, and concatenation.
In summary, sequences in Python are essential for organizing and managing data. Their diverse types and the operations they support make them invaluable for a wide range of programming tasks. By mastering sequences, developers can write more efficient and effective code, ultimately leading to better software solutions.
Author Profile
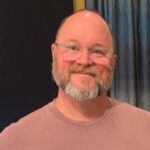
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?