How Can You Call an Async Function from a Non-Async Context?
In the ever-evolving landscape of programming, asynchronous programming has emerged as a powerful paradigm that allows developers to write code that can perform tasks concurrently, improving efficiency and responsiveness. However, as developers embrace the benefits of asynchronous functions, they often encounter a common challenge: how to call an async function from a non-async context. This seemingly straightforward task can lead to confusion and frustration, especially for those new to the async/await syntax. In this article, we’ll demystify this process, providing you with the insights and strategies needed to seamlessly integrate async functions into your codebase, regardless of the surrounding context.
Understanding how to bridge the gap between asynchronous and synchronous code is crucial for any developer looking to harness the full potential of modern programming languages. Async functions allow for non-blocking operations, enabling applications to remain responsive while executing time-consuming tasks. However, when you find yourself in a situation where you need to invoke these async functions from a traditional synchronous context, the intricacies of JavaScript’s event loop and promise handling come into play. This article will guide you through the various approaches to achieve this, ensuring that you can effectively manage your code’s flow without sacrificing performance or readability.
As we delve deeper into this topic, we will explore practical techniques and best practices for calling
Understanding Async and Non-Async Functions
When working with JavaScript, it’s essential to comprehend the distinction between asynchronous and synchronous functions. Asynchronous functions, defined using the `async` keyword, allow for non-blocking operations, meaning they can execute without freezing the execution of the program. In contrast, non-async functions execute synchronously, completing one operation before starting another.
To effectively call an async function from a non-async context, developers can utilize various methods, including immediately invoking the async function, using Promises, or employing an IIFE (Immediately Invoked Function Expression).
Calling an Async Function Directly
An async function can be called directly from a non-async function, but it returns a Promise. This requires handling the Promise to access the result of the async operation.
Example:
“`javascript
async function fetchData() {
const response = await fetch(‘https://api.example.com/data’);
return response.json();
}
function callAsyncFunction() {
const result = fetchData(); // returns a Promise
result.then(data => console.log(data)).catch(error => console.error(error));
}
“`
In the above code, the `fetchData` function is called, and since it is asynchronous, it returns a Promise. The `then` method is used to handle the resolved value.
Using an IIFE to Handle Async Operations
An Immediately Invoked Function Expression (IIFE) can be an effective strategy to call an async function within a non-async context. This approach allows for using `await` inside an async function without modifying the surrounding function’s structure.
Example:
“`javascript
function callAsyncFunction() {
(async () => {
const data = await fetchData();
console.log(data);
})();
}
“`
In this snippet, the async function is wrapped in parentheses and invoked immediately, providing access to `await`.
Handling Errors in Async Calls
Error handling is critical when working with async functions, especially when calling them from non-async contexts. Using `try/catch` blocks within an async IIFE is a common practice to manage errors effectively.
Example:
“`javascript
function callAsyncFunction() {
(async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(‘Error fetching data:’, error);
}
})();
}
“`
This structure allows for catching and logging errors that may occur during the async operation.
Comparison of Calling Methods
Method | Description | Error Handling |
---|---|---|
Direct Call | Calls async function directly, returns a Promise | Requires `.then()` |
IIFE | Uses an IIFE to call async function | Can use `try/catch` |
By selecting the appropriate method for calling async functions from non-async contexts, developers can create more efficient and manageable code, ensuring proper control over asynchronous operations and their corresponding error management.
Understanding Async and Non-Async Functions
In JavaScript, functions can be categorized as synchronous (blocking) or asynchronous (non-blocking). Synchronous functions execute in sequence, meaning each function must complete before the next begins. Conversely, asynchronous functions allow the program to continue executing while waiting for an operation to complete. This asynchronous behavior is crucial in scenarios involving network requests or file operations where waiting for a response could lead to performance bottlenecks.
Key Characteristics:
- Synchronous Functions:
- Execute in order.
- Block the thread until completion.
- Asynchronous Functions:
- Can be executed out of order.
- Use callbacks, promises, or async/await syntax.
- Enable non-blocking operations.
Calling Async Functions from Non-Async Context
To invoke an asynchronous function from a non-async context, you can use different strategies depending on the JavaScript environment and the specific requirements of your application. The most common methods include using Promises or handling callbacks.
**Using Promises:**
When an async function is called, it returns a Promise. You can handle this Promise using `.then()` and `.catch()` methods.
“`javascript
async function fetchData() {
// Simulate a network request
return “Data fetched”;
}
function nonAsyncFunction() {
fetchData()
.then(data => {
console.log(data); // Output: Data fetched
})
.catch(error => {
console.error(“Error:”, error);
});
}
“`
**Using Callbacks:**
If you prefer to use a callback approach, you can pass a function to handle the result of the async operation.
“`javascript
async function fetchData(callback) {
const data = await new Promise(resolve => setTimeout(() => resolve(“Data fetched”), 1000));
callback(data);
}
function nonAsyncFunction() {
fetchData(data => {
console.log(data); // Output: Data fetched
});
}
“`
Utilizing IIFE for Async Calls
An Immediately Invoked Function Expression (IIFE) can also be utilized to call async functions from a non-async context. This method allows you to define and execute an asynchronous function immediately.
“`javascript
async function fetchData() {
return “Data fetched”;
}
function nonAsyncFunction() {
(async () => {
const data = await fetchData();
console.log(data); // Output: Data fetched
})();
}
“`
Handling Errors in Async Calls
Error handling is critical when dealing with asynchronous functions. Using `try/catch` blocks within an async function or `.catch()` with Promises can help manage errors effectively.
**Example using try/catch:**
“`javascript
async function fetchData() {
throw new Error(“Failed to fetch data”);
}
function nonAsyncFunction() {
(async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(“Error:”, error.message); // Output: Error: Failed to fetch data
}
})();
}
“`
**Error Handling with Promises:**
“`javascript
function nonAsyncFunction() {
fetchData()
.then(data => {
console.log(data);
})
.catch(error => {
console.error(“Error:”, error.message); // Output: Error: Failed to fetch data
});
}
“`
This structured approach ensures that you can effectively manage async calls from non-async contexts while keeping your code clean and understandable.
Expert Insights on Calling Async Functions from Non-Async Contexts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively call an async function from a non-async context, one must utilize the `Promise` constructor or the `then()` method. This allows the non-async function to handle the asynchronous operation without blocking the main thread, ensuring smooth execution.”
Michael Chen (JavaScript Architect, CodeCraft Solutions). “Using an IIFE (Immediately Invoked Function Expression) is a practical approach to invoke async functions within non-async code. By wrapping the async call in an IIFE, developers can manage the asynchronous flow while maintaining a clean code structure.”
Sarah Thompson (Lead Developer, NextGen Web Technologies). “It is crucial to handle potential errors when calling async functions from non-async contexts. Implementing try-catch blocks within an async function ensures that any exceptions are caught, preventing unhandled promise rejections that could disrupt the application.”
Frequently Asked Questions (FAQs)
What does it mean to call an async function from a non-async context?
Calling an async function from a non-async context refers to executing an asynchronous function without being within an async function itself. This can lead to challenges in handling promises and managing the asynchronous flow of the program.
How can I call an async function from a non-async function?
You can call an async function from a non-async function by using the `.then()` method to handle the promise returned by the async function. Alternatively, you can use an IIFE (Immediately Invoked Function Expression) that is async to encapsulate the call.
What is an IIFE and how is it used to call async functions?
An IIFE is a JavaScript function that runs as soon as it is defined. To call an async function from a non-async context, you can define an async IIFE and invoke it immediately, allowing you to use `await` within its scope.
Can I use the `await` keyword in a non-async function?
No, the `await` keyword can only be used within an async function. Attempting to use `await` in a non-async function will result in a syntax error.
What are the potential issues when calling async functions from non-async contexts?
Potential issues include unhandled promise rejections, difficulty in managing execution flow, and challenges in error handling. It can also lead to confusion regarding the timing of operations and their results.
Is there a way to handle errors when calling async functions from non-async functions?
Yes, you can handle errors by using the `.catch()` method on the promise returned by the async function. This allows you to manage exceptions and ensure that errors are properly caught and handled.
In modern programming, particularly within JavaScript, the ability to call asynchronous functions from non-asynchronous contexts is a critical skill. Asynchronous functions, defined with the `async` keyword, allow for operations that may take time to complete—such as network requests or file I/O—without blocking the execution of other code. However, these functions return promises, which can complicate their integration into synchronous code structures. Understanding how to effectively manage this interaction is essential for maintaining responsive applications.
One common approach to invoke an async function from a non-async context is to use the `.then()` method of the promise returned by the async function. This allows developers to handle the result of the async operation once it completes. Alternatively, using an immediately invoked async function expression (IIFE) can also provide a clean solution, encapsulating the async call and allowing for the use of `await` within its scope. Both techniques emphasize the importance of promise handling in JavaScript and the need for careful error management through `.catch()` or try-catch blocks in async functions.
Key takeaways include the importance of understanding promise resolution and the implications of blocking versus non-blocking operations in application design. Developers should familiarize themselves with the various methods of handling async
Author Profile
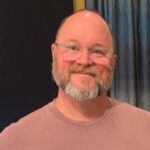
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?