How Can You Check the Status of a Kubernetes Node Using Golang?
In the ever-evolving landscape of cloud-native technologies, Kubernetes stands out as a powerful orchestration tool that simplifies the management of containerized applications. As organizations increasingly rely on Kubernetes to streamline their deployment processes, understanding the health and status of nodes within a cluster becomes paramount. For developers and DevOps engineers, having the ability to programmatically check the status of Kubernetes nodes can lead to more efficient monitoring, troubleshooting, and resource management. In this article, we’ll explore how to harness the power of Go, a language known for its simplicity and performance, to query and retrieve the status of Kubernetes nodes effectively.
Kubernetes nodes are the backbone of any cluster, serving as the worker machines that run applications. Each node’s status can provide crucial insights into the overall health of the cluster, indicating whether nodes are ready, not ready, or experiencing issues. By leveraging the Kubernetes API, developers can interact with the cluster to fetch this vital information. Using Go, with its robust libraries and straightforward syntax, makes it an ideal choice for building tools that can seamlessly integrate with Kubernetes and automate the status-checking process.
In this article, we will guide you through the steps to create a Go application that retrieves the status of Kubernetes nodes. We will touch upon the necessary libraries, the structure of the
Setting Up the Kubernetes Client
To interact with Kubernetes using Go, you first need to set up a Kubernetes client. The official Go client for Kubernetes is `client-go`. You can install it using the following command:
“`bash
go get k8s.io/client-go@latest
“`
This library allows you to communicate with your Kubernetes cluster programmatically. Ensure that you also have the necessary dependencies such as `k8s.io/apimachinery` and `k8s.io/kube-openapi`.
Configuring the Client
Once you have the client installed, you must configure it to connect to your Kubernetes cluster. This is typically done by loading the configuration from your `kubeconfig` file, which is usually located at `~/.kube/config`. Here’s how you can set it up in your Go application:
“`go
import (
“context”
“fmt”
“os”
“path/filepath”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
)
func getKubeClient() (*kubernetes.Clientset, error) {
kubeconfig := filepath.Join(os.Getenv(“HOME”), “.kube”, “config”)
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
return nil, err
}
return kubernetes.NewForConfig(config)
}
“`
This function will return a Kubernetes clientset that you can use to interact with the cluster.
Retrieving Node Status
With the client properly configured, you can now retrieve the status of nodes in your Kubernetes cluster. The following example demonstrates how to list all nodes and their statuses:
“`go
func getNodeStatus(clientset *kubernetes.Clientset) {
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
fmt.Printf(“Error retrieving nodes: %v\n”, err)
return
}
for _, node := range nodes.Items {
fmt.Printf(“Name: %s, Status: %s\n”, node.Name, node.Status.Conditions)
}
}
“`
In this function, we retrieve a list of nodes and iterate through them, printing each node’s name and status.
Understanding Node Status Structure
The status of each node contains several conditions that provide insights into the node’s health and availability. The conditions are represented as a list of `NodeCondition` objects, which include fields such as:
- Type: The type of condition (e.g., Ready, DiskPressure, MemoryPressure).
- Status: The status of the condition (True, , Unknown).
- LastHeartbeatTime: The last time the node reported its status.
- Reason: A brief description of the last transition.
Here is an example table illustrating the possible values for node conditions:
Condition Type | Status | Reason |
---|---|---|
Ready | True | KubeletReady |
DiskPressure | ||
MemoryPressure | ||
NetworkUnavailable |
By analyzing these conditions, you can effectively monitor the health of your Kubernetes nodes and take appropriate actions based on their statuses.
Setting Up the Kubernetes Client in Go
To interact with Kubernetes using Go, you must set up the Kubernetes client. This can be done using the official Kubernetes Go client library. Ensure you have the necessary dependencies in your project.
- Install the Kubernetes client-go package:
“`bash
go get k8s.io/client-go@latest
“`
- Import the required packages in your Go file:
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
“`
Creating a Kubernetes Client
You need to create a Kubernetes client to interact with the cluster. This requires a kubeconfig file, which is typically located at `~/.kube/config`.
“`go
// Load kubeconfig
config, err := clientcmd.BuildConfigFromFlags(“”, “/path/to/kubeconfig”)
if err != nil {
panic(err.Error())
}
// Create a clientset
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err.Error())
}
“`
Fetching Node Status
Once you have the clientset, you can retrieve the status of nodes in your Kubernetes cluster. The following function demonstrates how to get and print the status of all nodes.
“`go
func getNodeStatus(clientset *kubernetes.Clientset) {
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
panic(err.Error())
}
for _, node := range nodes.Items {
fmt.Printf(“Name: %s\n”, node.Name)
fmt.Printf(“Status: %s\n”, node.Status.Conditions[0].Status)
fmt.Printf(“Roles: %v\n”, node.Labels[“kubernetes.io/role”])
fmt.Println()
}
}
“`
Understanding Node Conditions
Node conditions provide a snapshot of the node’s status. The main conditions include:
- Ready: Indicates whether the node is ready to accept pods.
- MemoryPressure: Indicates if the node is under memory pressure.
- DiskPressure: Indicates if the node is under disk pressure.
- PIDPressure: Indicates if the node has run out of process IDs.
- NetworkUnavailable: Indicates if the network is unavailable.
You can enhance the function to display all conditions of each node:
“`go
for _, condition := range node.Status.Conditions {
fmt.Printf(“Condition Type: %s, Status: %s\n”, condition.Type, condition.Status)
}
“`
Running the Application
Ensure your Go application has access to the Kubernetes cluster, and then run your application:
“`bash
go run main.go
“`
This will display the status of each node in the cluster, including their conditions and roles, providing a comprehensive overview of the cluster’s health. Adjust the kubeconfig path as necessary to point to your Kubernetes context.
Expert Insights on Retrieving Kubernetes Node Status with Golang
Dr. Emily Chen (Cloud Infrastructure Architect, Tech Innovations Inc.). “Utilizing Golang to interact with the Kubernetes API is a powerful approach for retrieving node status. The Go client library for Kubernetes provides robust tools that simplify this process, allowing developers to efficiently manage and monitor their clusters.”
Michael Torres (Senior DevOps Engineer, CloudSync Solutions). “When querying the status of Kubernetes nodes using Golang, it is essential to handle the API responses effectively. Implementing error handling and ensuring proper authentication will lead to more reliable and secure applications.”
Sarah Patel (Kubernetes Consultant, Open Source Experts). “For developers looking to get the status of Kubernetes nodes, leveraging the client-go library in Golang is highly recommended. It not only streamlines the process but also ensures that your application adheres to best practices in Kubernetes resource management.”
Frequently Asked Questions (FAQs)
How can I retrieve the status of a Kubernetes node using Golang?
You can retrieve the status of a Kubernetes node by utilizing the Kubernetes client-go library. First, set up a client configuration, then use the client to access the nodes and fetch their statuses.
What is the client-go library in Golang?
The client-go library is the official Go client for interacting with Kubernetes. It provides APIs and utilities to communicate with the Kubernetes API server, allowing developers to manage Kubernetes resources programmatically.
What permissions are required to access node status in Kubernetes?
To access node status, you need to have the appropriate RBAC (Role-Based Access Control) permissions. Typically, you should have at least `get` and `list` permissions on the `nodes` resource.
Can I check the status of multiple nodes at once using Golang?
Yes, you can check the status of multiple nodes by listing all nodes using the client-go library and iterating through the returned list to access each node’s status.
What are some common node statuses in Kubernetes?
Common node statuses include `Ready`, `NotReady`, `Unknown`, and `SchedulingDisabled`. These statuses indicate the health and availability of the nodes in the cluster.
Is it necessary to handle errors when retrieving node status in Golang?
Yes, error handling is crucial when retrieving node status. It ensures that your application can gracefully handle issues such as network failures, permission errors, or API server unavailability.
In summary, obtaining the status of a Kubernetes node using Golang involves leveraging the Kubernetes client-go library, which provides a robust API for interacting with Kubernetes resources. By setting up a client configuration, developers can access the Kubernetes API to retrieve detailed information about nodes, including their conditions, capacity, and utilization. This process typically involves creating a Kubernetes client, querying the node resources, and parsing the returned data to extract the desired status information.
One of the key takeaways from this discussion is the importance of understanding the structure of Kubernetes resources and how to effectively use the client-go library. Familiarity with the Kubernetes API and the Go programming language is essential for efficiently querying and processing node status. Additionally, handling errors and ensuring proper authentication with the Kubernetes cluster are crucial steps in this process.
Furthermore, developers should consider implementing monitoring and alerting mechanisms based on the node status information retrieved. This proactive approach helps in maintaining the health of the Kubernetes cluster and ensures that any issues with node status are promptly addressed. Overall, using Golang to interact with Kubernetes nodes provides a powerful toolset for developers aiming to enhance their cloud-native applications.
Author Profile
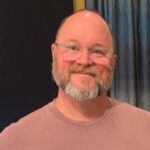
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?