Why Is My Set Object Not Subscriptable in Python?
In the world of Python programming, encountering errors is an inevitable part of the learning process. One such error that often leaves developers scratching their heads is the “Set Object Is Not Subscriptable” message. This seemingly cryptic phrase can halt your code in its tracks, but understanding its implications is crucial for anyone looking to master Python. Whether you’re a seasoned coder or a budding enthusiast, unraveling the mystery behind this error can enhance your coding skills and deepen your grasp of Python’s data structures.
At its core, the “Set Object Is Not Subscriptable” error arises from a fundamental misunderstanding of how sets operate in Python. Unlike lists or dictionaries, sets are designed for unique, unordered collections of items, which means they lack the indexing capabilities that allow for item retrieval via a specific position. This limitation can lead to frustrating moments when you attempt to access elements in a way that is familiar with other data types but incompatible with sets.
Understanding the nuances of Python’s data structures is essential for effective programming. By delving into the reasons behind this error, you can not only troubleshoot your own code but also gain insights into the broader principles of Python’s design. In the following sections, we will explore the nature of sets, common pitfalls, and best practices to
Understanding the Error Message
The error message “Set object is not subscriptable” typically arises in programming languages like Python when a user attempts to access elements of a set using indexing or key-based access. Unlike lists or dictionaries, sets are unordered collections of unique items, meaning they do not support indexing.
To clarify, here are the main characteristics of a set:
- Unordered: Elements do not have a fixed position.
- Unique: No duplicate elements are allowed.
- Mutable: Elements can be added or removed.
When you attempt to use square brackets (e.g., `set_variable[index]`) to access an element, Python raises the “TypeError: ‘set’ object is not subscriptable.” This is because the set does not maintain an index for its items.
Common Causes of the Error
Several scenarios can lead to the “Set object is not subscriptable” error:
- Incorrect Data Type Usage: Attempting to index a set instead of a list or dictionary.
- Misunderstanding Set Behavior: Assuming sets behave like lists or tuples due to their similar syntax.
- Code Logic Errors: Writing code that mistakenly assumes the existence of an index for set items.
Examples of the Error
To illustrate, consider the following examples:
“`python
Example 1: Attempting to access a set element by index
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError: ‘set’ object is not subscriptable
“`
“`python
Example 2: Attempting to use a set in a way similar to a list
my_set = {10, 20, 30}
for i in range(len(my_set)):
print(my_set[i]) Raises TypeError: ‘set’ object is not subscriptable
“`
Correct Ways to Access Set Elements
Since sets do not support indexing, alternative methods must be employed to access their elements:
- Looping through the Set: You can iterate over the elements directly.
- Using the `in` Keyword: Check for the existence of an element.
- Converting to a List: If index-based access is essential, convert the set to a list first.
Access Methods Comparison
The following table summarizes different methods to access elements in a set versus a list:
Data Structure | Access Method | Example |
---|---|---|
Set | Looping |
for item in my_set: print(item)
|
Set | Membership Check |
if 10 in my_set: print("Exists")
|
List | Indexing |
my_list[0]
|
By recognizing the limitations of sets and employing the appropriate access methods, programmers can effectively avoid the “Set object is not subscriptable” error.
Understanding the Error Message
The error message “Set object is not subscriptable” typically arises in Python when an attempt is made to index or slice a set object, which is not supported. Sets in Python are unordered collections of unique elements, and they do not support indexing or slicing like lists or tuples.
Common Causes of the Error
- Indexing a Set: Attempting to access elements via an index, e.g., `my_set[0]`.
- Slicing a Set: Trying to slice a set, e.g., `my_set[1:3]`.
- Using a Set in a Context that Requires Subscriptable Objects: Passing a set where a list or tuple is expected.
Example Scenarios
- Indexing Attempt:
“`python
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError
“`
- Slicing Attempt:
“`python
my_set = {1, 2, 3}
print(my_set[1:3]) Raises TypeError
“`
How to Resolve the Error
To resolve the “Set object is not subscriptable” error, consider the following approaches:
- Convert to List: If you need to access elements by index, convert the set to a list.
“`python
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list[0]) Now this works
“`
- Iterate Over the Set: If the goal is to access elements, use a loop to iterate over the set.
“`python
for item in my_set:
print(item)
“`
- Use Membership Tests: Instead of indexing, utilize the `in` keyword to check for the presence of an element in the set.
“`python
if 2 in my_set:
print(“2 is in the set”)
“`
Alternative Data Structures
If you frequently need index access or ordered collections, consider using alternative data structures:
Data Structure | Characteristics |
---|---|
List | Ordered, allows duplicates, supports indexing |
Tuple | Ordered, immutable, supports indexing |
Dictionary | Key-value pairs, unordered, keys are unique |
Collections. OrderedDict | Ordered, allows duplicates, supports indexing by key |
Using these alternatives can help prevent the subscriptable error while achieving your data handling needs more effectively.
Best Practices
- Always be aware of the data structure’s properties you are working with.
- Use sets primarily for membership tests and unique collections, avoiding direct indexing.
- Document your code to clarify the choice of data structures, especially when collaborating with others.
Understanding the ‘Set Object Is Not Subscriptable’ Error in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The ‘Set Object Is Not Subscriptable’ error in Python occurs because sets are unordered collections of unique elements. Attempting to access elements using an index, as one would with lists or tuples, leads to this error. It is crucial for developers to understand the data structures they are working with to avoid such pitfalls.”
James Liu (Lead Software Engineer, CodeCraft Solutions). “When encountering the ‘Set Object Is Not Subscriptable’ error, it is often a sign that a developer is misusing the set data type. Instead of trying to access elements by index, one should consider converting the set to a list or using iteration to access elements. This highlights the importance of choosing the right data structure for the task at hand.”
Linda Green (Python Educator, Coding Academy). “This error serves as a reminder of the fundamental differences between mutable and immutable data types in Python. Educating new programmers about the characteristics of sets, including their inability to be indexed, is essential for fostering good coding practices and preventing common mistakes.”
Frequently Asked Questions (FAQs)
What does the error “Set Object Is Not Subscriptable” mean?
This error indicates that you are attempting to access elements of a set using indexing or subscript notation, which is not supported because sets are unordered collections in Python.
How can I resolve the “Set Object Is Not Subscriptable” error?
To resolve this error, you should avoid using indexing with sets. Instead, consider converting the set to a list or using iteration methods to access its elements.
Can I convert a set to a list to access elements by index?
Yes, you can convert a set to a list using the `list()` function, allowing you to access its elements by index. However, remember that the order of elements in a set is not guaranteed.
Are there alternatives to using indexing with sets?
Yes, you can use loops, set comprehensions, or the `in` keyword to check for membership without needing to access elements by index.
What are the common scenarios that lead to this error?
Common scenarios include mistakenly trying to access elements of a set as if it were a list or tuple, such as using syntax like `my_set[0]`.
Is this error specific to Python, or does it occur in other programming languages?
This error is primarily associated with Python due to its specific handling of data types. Other programming languages may have similar concepts but may not produce the same error message.
The error message “Set Object Is Not Subscriptable” typically arises in Python programming when a developer attempts to access elements of a set using indexing or slicing. Sets in Python are unordered collections of unique elements, which means they do not support indexing like lists or tuples. This fundamental characteristic of sets is crucial for developers to understand to avoid such errors in their code.
When encountering this error, it is important to recognize that sets are designed for membership testing and eliminating duplicate entries rather than for ordered access to elements. To retrieve elements from a set, one must use iteration or conversion to a different data structure, such as a list or tuple, that supports indexing. This understanding can help prevent similar issues in future coding endeavors.
the “Set Object Is Not Subscriptable” error serves as a reminder of the distinct properties of Python data structures. By adhering to the intended use of sets and leveraging appropriate methods for accessing their elements, developers can enhance their coding efficiency and reduce the likelihood of encountering such errors. Mastery of these concepts is essential for effective programming in Python.
Author Profile
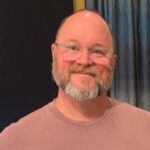
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?