How Do You Print a Number in Python? A Step-by-Step Guide!
In the world of programming, the ability to communicate information is paramount, and one of the most fundamental ways to do this is through outputting data. Whether you’re a novice coder just embarking on your programming journey or a seasoned developer looking to brush up on the basics, understanding how to print a number in Python is an essential skill. Python, known for its simplicity and readability, offers straightforward methods to display data, making it an ideal language for beginners and experts alike.
Printing a number in Python might seem trivial at first glance, but it serves as a gateway to more complex concepts in programming. This basic operation not only helps you verify the results of calculations but also allows you to debug your code effectively. As you delve deeper into Python, you’ll discover that mastering the art of outputting data can enhance your programming prowess and streamline your coding process.
In this article, we will explore the various ways to print numbers in Python, from the simplest commands to more advanced formatting techniques. Whether you’re looking to display integers, floats, or even formatted strings, you’ll find that Python’s versatility makes it easy to convey information clearly and effectively. Join us as we uncover the nuances of this fundamental operation and equip you with the skills to make your Python programs more interactive and informative.
Using the print() Function
The primary method for displaying output in Python is the `print()` function. This versatile function can output various data types, including integers, floats, strings, and more. The syntax is straightforward:
“`python
print(object(s), sep=’ ‘, end=’\n’, file=sys.stdout, flush=)
“`
- object(s): The object(s) to be printed.
- sep: A string inserted between the objects, defaulting to a space.
- end: A string appended after the last object, defaulting to a newline.
- file: An object with a write method, defaults to the standard output.
- flush: A boolean indicating whether to forcibly flush the stream.
Printing Numbers
To print a number in Python, you can simply pass the number as an argument to the `print()` function. Here are examples of printing different types of numbers:
“`python
Integer
print(42)
Float
print(3.14)
Negative number
print(-7)
“`
Each of these commands will output the number directly to the console.
Formatting Output
When printing numbers, you may want to format the output for better readability or to meet specific requirements. Python provides several ways to format numbers:
- f-strings (Python 3.6+): A convenient way to embed expressions inside string literals.
- str.format(): A more traditional method that allows for complex formatting.
- Percent formatting: An older style that uses the `%` operator.
Here’s a comparison of these methods:
Method | Example | Output |
---|---|---|
f-string | name = “Alice”; age = 30; print(f”{name} is {age} years old.”) | Alice is 30 years old. |
str.format() | print(“{} is {} years old.”.format(name, age)) | Alice is 30 years old. |
Percent formatting | print(“%s is %d years old.” % (name, age)) | Alice is 30 years old. |
Using these formatting techniques allows for greater control over how numbers are displayed.
Using Commas for Thousands
When dealing with large numbers, you may want to include commas for better readability. This can be achieved using formatted strings. For instance, you can specify that you want to use a comma as a thousands separator:
“`python
large_number = 1000000
print(f”{large_number:,}”) Output: 1,000,000
“`
This makes it easier to read large numerical values, particularly in financial or statistical applications.
Advanced Printing Techniques
For more advanced printing scenarios, you can customize the output further, such as controlling decimal precision. Here’s how to format a float to two decimal places:
“`python
pi = 3.14159
print(f”{pi:.2f}”) Output: 3.14
“`
This method allows you to present numerical data in a way that suits the context of your application.
Using the print() Function
The primary method to output a number in Python is through the `print()` function. This built-in function allows you to display numbers (as well as strings and other data types) on the console. Here’s how to use it effectively:
“`python
number = 42
print(number)
“`
In this example, the variable `number` holds the integer value `42`, and the `print()` function outputs this value to the console.
Printing Multiple Numbers
If you wish to print multiple numbers at once, you can pass several arguments to the `print()` function. Each argument can be separated by a comma.
“`python
a = 10
b = 20
print(a, b)
“`
This code will output:
“`
10 20
“`
You can also customize the separator between numbers by using the `sep` parameter:
“`python
print(a, b, sep=”, “)
“`
The output will be:
“`
10, 20
“`
Formatted Output
For more control over how numbers are displayed, Python offers formatted strings. You can use f-strings (available in Python 3.6 and later) for this purpose:
“`python
value = 3.14159
print(f”The value of pi is approximately {value:.2f}.”)
“`
This will output:
“`
The value of pi is approximately 3.14.
“`
For older versions of Python, you can use the `format()` method:
“`python
print(“The value of pi is approximately {:.2f}.”.format(value))
“`
Both examples demonstrate how to format floating-point numbers to two decimal places.
Using String Formatting with % Operator
Another way to format numbers is by using the `%` operator, which is an older method but still widely used:
“`python
print(“The value of pi is approximately %.2f.” % value)
“`
This will produce the same output as the previous examples. The `%.2f` indicates that the number should be formatted as a floating-point number with two decimal places.
Printing with Newlines and Tabs
You can enhance the readability of printed output by including newline (`\n`) and tab (`\t`) characters:
“`python
print(“Number 1:\n\t”, a)
print(“Number 2:\n\t”, b)
“`
This results in:
“`
Number 1:
10
Number 2:
20
“`
Using the End Parameter
By default, the `print()` function ends with a newline. However, you can change this behavior using the `end` parameter:
“`python
print(“Hello”, end=”, “)
print(“World!”)
“`
This will output:
“`
Hello, World!
“`
The `end` parameter allows you to specify what to print at the end of the output, providing flexibility in formatting.
Summary of Print Variants
The following table summarizes the various ways to print numbers in Python:
Method | Example Code | Description |
---|---|---|
Basic Print | `print(number)` | Outputs a single number. |
Multiple Arguments | `print(a, b)` | Outputs multiple numbers. |
Custom Separator | `print(a, b, sep=”, “)` | Customizes the separator between outputs. |
f-Strings | `print(f”The value is {number:.2f}”)` | Formats numbers within a string. |
`%` Formatting | `print(“Value: %.2f” % number)` | Old-style formatting for numbers. |
Newline/Tab Characters | `print(“Text\n\t”, number)` | Adds newlines and tabs for formatting. |
Custom End Character | `print(“Hello”, end=”, “)` | Changes what is printed at the end. |
By utilizing these various methods and features of the `print()` function, you can effectively display numbers in Python in a clear and customizable manner.
Expert Insights on Printing Numbers in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Printing numbers in Python is straightforward, utilizing the built-in `print()` function. It is essential for beginners to understand that this function can handle various data types, including integers and floats, making it versatile for different applications.”
Michael Chen (Lead Python Developer, Tech Innovations Inc.). “When printing numbers in Python, developers should consider formatting options. Using formatted strings or the `format()` method enhances the readability of output, especially when dealing with multiple variables or complex expressions.”
Sarah Lopez (Educator and Author, Python Programming for Beginners). “For those new to programming, mastering the `print()` function is a critical first step. It not only demonstrates how to output data but also lays the groundwork for understanding more complex concepts like debugging and logging in software development.”
Frequently Asked Questions (FAQs)
How do I print a number in Python?
To print a number in Python, use the `print()` function. For example, `print(5)` will display the number 5 in the output.
Can I print multiple numbers in one line?
Yes, you can print multiple numbers in one line by separating them with commas within the `print()` function. For instance, `print(1, 2, 3)` will output `1 2 3`.
What if I want to format the printed number?
You can format printed numbers using f-strings or the `format()` method. For example, `print(f”The number is: {5}”)` or `print(“The number is: {}”.format(5))` will include the number in a formatted string.
Is it possible to print numbers with specific decimal places?
Yes, you can specify the number of decimal places using formatted strings. For example, `print(f”{3.14159:.2f}”)` will print `3.14`, rounding to two decimal places.
How can I print a number without a newline at the end?
To print a number without a newline, use the `end` parameter in the `print()` function. For example, `print(5, end=’ ‘)` will print 5 followed by a space instead of a newline.
Can I print numbers in different bases (binary, hexadecimal)?
Yes, you can print numbers in different bases using the `bin()` and `hex()` functions. For example, `print(bin(10))` will output `0b1010` for binary, and `print(hex(10))` will output `0xa` for hexadecimal.
In summary, printing a number in Python is a straightforward process that can be accomplished using the built-in `print()` function. This function is versatile and can handle various data types, including integers, floats, and even strings that represent numbers. By simply passing the number as an argument to `print()`, users can display the desired output on the console, making it an essential tool for debugging and user interaction.
Moreover, Python offers additional formatting options to enhance the presentation of printed numbers. Techniques such as f-strings, the `format()` method, and the older `%` operator allow for precise control over how numbers are displayed, including controlling decimal places, padding, and alignment. Understanding these formatting options can significantly improve the readability of output, especially when dealing with complex data sets or user interfaces.
Ultimately, mastering the `print()` function and its formatting capabilities is crucial for any Python programmer. It not only aids in effective communication of information but also enhances the overall user experience by presenting data in a clear and organized manner. As one progresses in their Python journey, these skills will prove invaluable in both simple scripts and more complex applications.
Author Profile
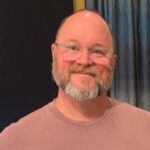
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?