What Does ‘While True’ Mean in Python: Understanding Its Purpose and Usage?
### Introduction
In the world of programming, the ability to control the flow of execution is paramount, and Python offers a variety of tools to help developers achieve this. Among these tools, the `while` loop stands out as a powerful construct for creating repetitive tasks. But what happens when we combine it with the expression `True`? This seemingly simple phrase can unlock a realm of possibilities, enabling programmers to create infinite loops that can run until explicitly interrupted. Whether you’re a novice eager to grasp the fundamentals or an experienced coder looking to refine your skills, understanding the implications of `while True` in Python is essential.
The `while True` statement serves as a cornerstone for building loops that continue indefinitely, allowing for dynamic and responsive programming. This construct is particularly useful in scenarios where the termination condition cannot be predetermined, such as waiting for user input, processing data streams, or managing ongoing tasks in applications. However, with great power comes great responsibility; using `while True` without proper control can lead to unresponsive programs or excessive resource consumption.
As we delve deeper into the mechanics of `while True`, we will explore its applications, best practices, and potential pitfalls. By the end of this journey, you’ll not only understand how to implement this powerful loop effectively but also appreciate the nuances
Understanding the While True Loop
The `while True` loop in Python is a control flow statement that allows the execution of a block of code repeatedly as long as the condition evaluates to true. In this case, since the condition is simply `True`, the loop will continue indefinitely until it is explicitly broken out of with a `break` statement or interrupted by an external factor, such as a keyboard interrupt.
### Use Cases for While True
The `while True` loop is particularly useful in scenarios where the exact number of iterations is not known beforehand. Common use cases include:
- User Input Handling: Continuously prompting the user for input until they provide a specific command to exit.
- Server Listening: Keeping a server active to listen for incoming requests.
- Game Loops: Maintaining the game state and updating it until the player decides to quit.
### Example of While True
Here is a simple example demonstrating a `while True` loop that asks for user input:
python
while True:
user_input = input(“Type ‘exit’ to quit: “)
if user_input.lower() == ‘exit’:
print(“Exiting the loop.”)
break
else:
print(f”You entered: {user_input}”)
In this example, the loop will keep prompting the user for input until they type ‘exit’.
### Important Considerations
When using `while True`, it’s crucial to ensure that there is a clear exit strategy to prevent the program from running indefinitely. Here are a few important points to consider:
- Performance: An infinite loop without a break statement can lead to high CPU usage and can crash the program.
- Debugging: If a loop runs indefinitely, debugging can become challenging. Always ensure that exit conditions are well-defined.
- Resource Management: Consider resource management in long-running loops, particularly when dealing with file operations or network connections.
### Loop Control Statements
In addition to the `break` statement, the `while True` loop can also utilize `continue` to skip the rest of the code within the loop and return to the beginning:
python
while True:
user_input = input(“Type a number (or ‘exit’ to quit): “)
if user_input.lower() == ‘exit’:
break
if not user_input.isdigit():
print(“That’s not a valid number. Try again.”)
continue
print(f”You entered the number: {user_input}”)
In this code snippet, if the user enters a non-numeric input, the loop prompts them again without executing the print statement.
Statement | Description |
---|---|
while True | Initiates an infinite loop. |
break | Exits the loop immediately. |
continue | Skips the rest of the current iteration and proceeds to the next iteration. |
By understanding how to effectively use `while True`, programmers can create robust applications that handle various tasks efficiently.
Understanding the While True Loop in Python
The `while True` loop in Python is a fundamental construct used to create infinite loops. This type of loop will continue to execute indefinitely until it is broken by a specific condition or an external interruption. Here’s a deeper look into its structure and usage:
### Structure of a While True Loop
A `while True` loop is structured as follows:
python
while True:
# Code to be executed repeatedly
### Key Components
- Condition: The condition is always `True`, which means the loop does not have a natural termination point.
- Loop Body: Contains the code that will be executed on each iteration.
### Common Use Cases
- Event Listening: Often used in applications that require continuous monitoring or listening for events, such as server applications.
- User Input: Useful for repeatedly prompting the user for input until valid data is provided.
- Game Loops: Frequently employed in game development to keep the game running until a certain condition is met (like quitting the game).
### Example of a While True Loop
Here’s a simple example demonstrating its use:
python
while True:
user_input = input(“Enter ‘exit’ to quit: “)
if user_input.lower() == ‘exit’:
break
print(f”You entered: {user_input}”)
In this example, the loop will repeatedly ask the user for input until the user types ‘exit’, at which point the `break` statement will terminate the loop.
### Considerations and Best Practices
Using `while True` requires careful consideration to avoid unintentional infinite loops that can freeze or crash your program. Here are some best practices:
- Include a Break Condition: Always ensure there is a mechanism to exit the loop, like a `break` statement.
- Limit Resource Usage: Avoid CPU overutilization by including `sleep()` statements or conditions that reduce the loop’s frequency, especially in event-driven programming.
- Error Handling: Implement try-except blocks within the loop to gracefully handle any potential exceptions that may arise during execution.
### Comparison with Other Loop Structures
Loop Type | Description | Use Cases |
---|---|---|
`for` loop | Iterates over a sequence or range. | Processing lists or ranges. |
`while` loop | Continues until a specific condition fails. | General-purpose looping. |
`while True` | Infinite loop until explicitly broken. | Event listening, user input. |
`while True` is a powerful tool in Python that allows for repeated execution of a block of code. It is essential, however, to manage its execution carefully to maintain control over the program’s flow and prevent unintended consequences.
Understanding the Significance of While True in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘while True’ construct in Python is a fundamental aspect of creating infinite loops, which are essential for scenarios where continuous execution is necessary, such as in server processes or real-time data monitoring systems.”
Michael Thompson (Lead Python Developer, CodeCraft Solutions). “Using ‘while True’ can be both powerful and risky. It allows for creating loops that run indefinitely until a specific condition is met, but developers must ensure they implement proper exit conditions to prevent unintentional infinite loops that can crash applications.”
Sarah Lee (Computer Science Professor, University of Technology). “In educational settings, ‘while True’ serves as an excellent teaching tool for illustrating concepts of control flow and loop termination. It encourages students to think critically about conditions and the importance of breaking out of loops to maintain program efficiency.”
Frequently Asked Questions (FAQs)
What does “while True” mean in Python?
“while True” is a loop construct that creates an infinite loop in Python. It continues to execute the block of code within it until explicitly broken out of using a break statement or an exception occurs.
How do I exit a “while True” loop in Python?
You can exit a “while True” loop by using the `break` statement. This statement terminates the loop and transfers control to the next statement following the loop.
What are common use cases for “while True” in Python?
Common use cases for “while True” include continuously reading input from users, polling for events, or running a server that needs to handle requests indefinitely until a shutdown command is issued.
Can “while True” lead to performance issues in Python?
Yes, “while True” can lead to performance issues if not managed properly. An infinite loop without a proper exit condition or delay can consume excessive CPU resources, potentially freezing or crashing the program.
Is it possible to use “while True” with conditions inside the loop?
Yes, you can use conditions inside a “while True” loop to control the flow of execution. For example, you can check for certain conditions and use a break statement to exit the loop when those conditions are met.
What is the difference between “while True” and “for” loops in Python?
The primary difference is that “while True” creates an infinite loop that requires an explicit exit condition, while “for” loops iterate over a sequence (like a list or range) a finite number of times. “for” loops are typically used when the number of iterations is known beforehand.
The phrase “while True” in Python is a construct used to create an infinite loop. This loop continues to execute indefinitely until it is explicitly broken out of, usually through a break statement or an external interruption. It is commonly utilized in scenarios where a program needs to repeatedly perform a task until a certain condition is met, such as waiting for user input or continuously processing data until a termination signal is received.
One of the key aspects of using “while True” is the necessity for a mechanism to exit the loop. Without a proper exit strategy, the loop will run endlessly, which can lead to unresponsive programs or high CPU usage. Therefore, it is crucial to implement conditions that will allow the loop to terminate gracefully. This could involve checking for specific user commands, monitoring system states, or incorporating timeouts.
Additionally, “while True” loops can be particularly useful in event-driven programming, where the program needs to listen for events or messages continuously. They are also prevalent in server applications, where the server must remain active to handle incoming requests. However, developers should always be cautious when implementing such loops to avoid performance issues and ensure that the program remains efficient and responsive.
Author Profile
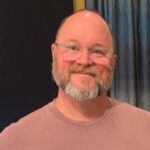
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?