How Can You Efficiently Append Multiple Items to a List in Python?
When it comes to programming in Python, one of the most versatile and frequently used data structures is the list. Lists allow you to store collections of items, making them invaluable for a wide range of applications, from data analysis to game development. However, as your projects grow in complexity, you may find yourself needing to add multiple items to a list at once. Understanding how to efficiently append multiple items to a list can streamline your code and enhance its readability, ultimately making your programming experience smoother and more enjoyable.
In Python, there are several methods available for appending multiple items to a list, each with its own advantages and use cases. Whether you’re pulling data from an external source, merging lists, or simply expanding your existing collection, knowing the right approach can save you time and effort. This topic not only covers the fundamental techniques but also delves into best practices that can help you write cleaner and more efficient code.
As you explore the various methods for appending items, you’ll discover how to leverage Python’s built-in functions and list comprehensions to enhance your coding prowess. By mastering these techniques, you’ll be better equipped to handle complex data structures and improve the overall performance of your applications. Get ready to unlock the full potential of Python lists and elevate your programming skills to new heights!
Using the `extend()` Method
The `extend()` method is a straightforward way to append multiple elements to a list. This method takes an iterable (like another list, tuple, or set) and adds each of its elements to the end of the list. This is particularly useful when you want to combine lists or add multiple items at once.
“`python
Example of using extend()
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
When using `extend()`, it is important to note that the original list is modified in place, and no new list is created.
Using the `+=` Operator
Another method to append multiple items to a list is by using the `+=` operator. This operator is functionally similar to `extend()` and can be used to add elements from an iterable directly to the list.
“`python
Example of using += operator
my_list = [1, 2, 3]
my_list += [4, 5, 6]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
This approach also modifies the original list and allows for an intuitive syntax that can improve code readability.
Using List Comprehensions
List comprehensions provide a powerful way to create new lists by applying an expression to each item in an iterable. While not a direct method for appending to an existing list, you can use a list comprehension to generate new items and then append them using `extend()` or `+=`.
“`python
Example of list comprehension with extend
my_list = [1, 2, 3]
my_list.extend([x for x in range(4, 7)])
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
This technique is particularly useful when you want to transform or filter the items being added.
Using a Loop
In some cases, you may want to append items one by one, particularly if you need to apply additional logic during the process. A simple `for` loop can accomplish this.
“`python
Example of using a loop
my_list = [1, 2, 3]
for item in [4, 5, 6]:
my_list.append(item)
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
This method provides flexibility, allowing for conditional logic or transformations as items are appended.
Comparison of Methods
The following table summarizes the different methods for appending multiple items to a list, highlighting their characteristics and use cases.
Method | Characteristics | Use Case |
---|---|---|
extend() | Modifies the list in place, accepts any iterable | Combining lists or adding multiple elements |
+= Operator | Similar to extend(), intuitive syntax | Appending multiple elements quickly |
List Comprehensions | Creates new items based on existing iterables | Transforming items before appending |
Loop | Allows for custom logic during appending | Appending items conditionally or with transformations |
Each of these methods has its own advantages, and the choice of which to use may depend on the specific requirements of your application or personal coding style.
Methods for Appending Multiple Items
Appending multiple items to a list in Python can be accomplished using several methods, each suited for different scenarios. The most common techniques include using the `extend()` method, list comprehension, and the `+=` operator. Here’s a detailed look at each method.
Using the `extend()` Method
The `extend()` method allows you to add elements from an iterable (like another list) to the end of your list. This method modifies the original list in place.
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
Key Points:
- In-place Modification: The original list is modified, and no new list is created.
- Iterable Requirement: The argument must be an iterable (list, tuple, set, etc.).
Using List Comprehension
List comprehension provides a concise way to create a new list by combining existing lists or generating new elements. To append items, you can create a new list and concatenate it with the original.
“`python
my_list = [1, 2, 3]
additional_items = [4, 5, 6]
my_list = my_list + [item for item in additional_items]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
Advantages:
- Flexibility: You can apply conditions or transformations while appending.
- Readability: The syntax can be more readable and expressive.
Using the `+=` Operator
The `+=` operator is another effective method to append items from an iterable to a list. It functions similarly to the `extend()` method and modifies the original list.
“`python
my_list = [1, 2, 3]
my_list += [4, 5, 6]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
Benefits:
- Simplicity: The syntax is straightforward and intuitive.
- In-place Addition: Like `extend()`, it updates the original list.
Performance Considerations
When choosing a method to append multiple items to a list, it’s essential to consider performance, especially when dealing with large datasets. Here’s a comparison:
Method | Time Complexity | In-place Modification |
---|---|---|
`extend()` | O(k) | Yes |
List Comprehension | O(n) | No |
`+=` | O(k) | Yes |
- `extend()` and `+=` are generally more efficient for large lists, as they do not require creating a new list.
- List comprehension is more flexible but can be slower due to the creation of an intermediate list.
Depending on the specific needs of your code, you can choose any of these methods to append multiple items to a list in Python effectively. Whether you prioritize performance or readability will determine the best choice for your application.
Expert Insights on Appending Multiple Items to a List in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When appending multiple items to a list in Python, utilizing the `extend()` method is often the most efficient approach. This method allows you to add elements from an iterable, making it ideal for merging lists or adding multiple items at once without the overhead of looping.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “For those who prefer a more functional programming style, using list comprehension can also be an effective way to append multiple items. This method not only enhances readability but also allows for the transformation of data as it is added to the list.”
Sarah Lin (Python Educator, LearnPython.org). “Understanding the difference between `append()` and `extend()` is crucial for Python developers. While `append()` adds a single element to the end of a list, `extend()` is specifically designed to add multiple elements, which can significantly streamline your code when working with larger datasets.”
Frequently Asked Questions (FAQs)
How can I append multiple items to a list in Python?
You can append multiple items to a list in Python using the `extend()` method, which adds each element from an iterable to the end of the list. For example, `my_list.extend([item1, item2, item3])` will add `item1`, `item2`, and `item3` to `my_list`.
Is there a difference between append() and extend() in Python?
Yes, `append()` adds its argument as a single element to the end of the list, while `extend()` iterates over its argument and adds each element to the list. For instance, `my_list.append([1, 2])` adds a single list, whereas `my_list.extend([1, 2])` adds the elements `1` and `2` individually.
Can I use the `+=` operator to append multiple items to a list?
Yes, the `+=` operator can be used to extend a list with multiple items. For example, `my_list += [item1, item2]` will add `item1` and `item2` to the end of `my_list`, similar to using the `extend()` method.
What happens if I try to append a list to another list using append()?
Using `append()` to add a list to another list will result in the entire list being added as a single element. For example, `my_list.append([1, 2])` will create a nested list, resulting in `my_list` containing the original elements plus a new sublist.
Can I append items of different data types to a list in Python?
Yes, Python lists are heterogeneous, allowing you to append items of different data types. You can append integers, strings, lists, or any other objects without any restrictions.
What is the best practice for appending a large number of items to a list?
For appending a large number of items, it is generally more efficient to use the `extend()` method or the `+=` operator instead of multiple calls to `append()`. This reduces the number of operations and improves performance.
In Python, appending multiple items to a list can be achieved through various methods, each suited for different scenarios. The most common approaches include using the `extend()` method, the `+=` operator, and the `append()` method in conjunction with unpacking. Understanding these methods allows for efficient list manipulation, catering to the needs of both beginners and experienced programmers.
The `extend()` method is particularly useful when you want to add elements from another iterable, such as a list or a tuple, directly to your existing list. This method modifies the original list in place and is generally preferred for its clarity and efficiency. Alternatively, the `+=` operator provides a concise way to achieve the same result, making it a popular choice for those who favor a more succinct syntax.
For scenarios where you need to append a single item that itself is a collection, the `append()` method can be combined with unpacking using the asterisk (*) operator. This allows you to add multiple elements from an iterable as individual items to the list, thereby enhancing the flexibility of list operations. Overall, choosing the appropriate method depends on the specific requirements of your code and the structure of the data you are working with.
Author Profile
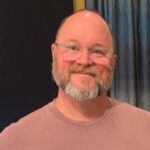
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?