How to Handle Out of Order Sequence Responses in Golang with HBase?
In the rapidly evolving landscape of data management, the ability to handle out-of-order sequences has emerged as a critical challenge for developers and engineers alike. As applications scale and data flows become increasingly complex, ensuring the integrity and accuracy of information can become daunting, especially when interfacing with distributed databases like HBase. This article delves into the intricacies of managing out-of-order sequences in Golang, a language celebrated for its efficiency and concurrency capabilities. By exploring the nuances of sequence responses in HBase, we aim to equip you with the knowledge needed to navigate this intricate terrain effectively.
At the heart of this topic lies the understanding of how Golang’s concurrency model can be leveraged to manage data sequences that arrive in a non-linear fashion. HBase, a powerful NoSQL database built on top of Hadoop, presents unique challenges when it comes to maintaining order and consistency in data retrieval and storage. As we explore the mechanisms for handling these out-of-order sequences, we will uncover best practices and strategies that can enhance the reliability of your applications.
Throughout this article, we will guide you through the fundamental concepts and techniques that underpin effective sequence response management in Golang and HBase. Whether you are a seasoned developer or a newcomer to the world of distributed systems, our insights will provide you
Understanding Out Of Order Sequences
Out of order sequences refer to a situation where data is processed or retrieved in a non-sequential manner. This can lead to complications, particularly in applications that depend on the order of operations, such as transaction processing systems. In the context of Golang and HBase, handling out of order sequences effectively is critical to maintaining data integrity and ensuring reliable performance.
Out of order sequences can arise due to various factors, including:
- Network delays
- Load balancing across distributed systems
- Asynchronous processing of requests
To mitigate these issues, developers can implement strategies to buffer, reorder, or batch process incoming data.
Implementing Sequence Management in Golang
In Golang, managing out of order sequences can be accomplished using channels and goroutines. The following approaches can be employed:
- Buffered Channels: Use buffered channels to temporarily hold incoming data until it can be processed in the correct order.
- Priority Queues: Implement a priority queue to manage the order of processing based on sequence numbers.
- Synchronization Primitives: Utilize mutexes and condition variables to ensure that data is processed in the desired order without race conditions.
Here’s a simple example of using a buffered channel to manage out of order sequences:
“`go
package main
import (
“fmt”
“sync”
)
func main() {
var wg sync.WaitGroup
ch := make(chan int, 5)
// Producer
for i := 0; i < 5; i++ {
wg.Add(1)
go func(n int) {
defer wg.Done()
ch <- n
}(i)
}
// Consumer
go func() {
for i := 0; i < 5; i++ {
fmt.Println(<-ch)
}
}()
wg.Wait()
}
```
This example demonstrates a simple producer-consumer model using a buffered channel, where data is sent and received asynchronously.
Handling Data in HBase
HBase, a NoSQL database, is designed for large-scale data storage and can efficiently handle out of order sequences. It allows for quick reads and writes, which is essential when managing out of order data.
Key features of HBase relevant to this context include:
- Column Families: Data can be grouped into column families, allowing for efficient querying and storage.
- Timestamps: HBase automatically includes timestamps with data entries, which can be used to manage the sequence of operations.
- Region Servers: Data is distributed across region servers, which can help balance load and reduce processing delays.
Best Practices for Managing Out Of Order Sequences
To effectively manage out of order sequences in Golang and HBase, consider the following best practices:
- Use Sequence Numbers: Assign unique sequence numbers to each data entry to track and reorder them as needed.
- Implement Retry Logic: In cases of failure, implement retry logic to ensure that data is processed correctly.
- Monitor Performance: Regularly monitor the performance of your system to identify bottlenecks related to out of order processing.
Technique | Description | Benefits |
---|---|---|
Buffered Channels | Temporarily holds data for orderly processing. | Increases throughput and reduces latency. |
Priority Queues | Processes data based on assigned priority or sequence. | Ensures critical data is handled first. |
Timestamps in HBase | Automatically manages versions and order of data. | Facilitates easy retrieval of the latest data. |
Understanding Out Of Order Sequences in Golang with HBase
Handling out-of-order sequences in distributed systems can be challenging, particularly when using a combination of Golang for backend services and HBase for data storage. In this section, we will delve into strategies for managing out-of-order data efficiently.
Challenges of Out Of Order Data
Out-of-order data can lead to several complications, including:
- Data Integrity: Ensuring that the sequence of operations or events is maintained.
- State Management: Difficulty in maintaining an accurate state when processing events.
- Performance Issues: Increased latency due to the need for additional checks or storage mechanisms.
Data Structures for Handling Out Of Order Sequences
Utilizing appropriate data structures is crucial for managing out-of-order sequences. Below are some recommended structures:
Data Structure | Description |
---|---|
Queue | Holds events in the order they are received, allowing for processing once all required events are available. |
Map | Maps sequence identifiers to their corresponding data, facilitating quick lookups and state management. |
Priority Queue | Orders events based on their sequence number, allowing for prioritized processing. |
Implementing Out Of Order Handling in Golang
To manage out-of-order sequences in Golang, the following implementation strategies can be employed:
- Event Buffering: Store incoming events temporarily until all necessary preceding events are received. This can be achieved using a map to track sequences.
“`go
type Event struct {
Sequence int
Data interface{}
}
var eventBuffer = make(map[int]Event)
func bufferEvent(event Event) {
eventBuffer[event.Sequence] = event
// Logic to check if preceding events are received
}
“`
- Sequence Validation: Before processing an event, ensure that all preceding events have been handled.
“`go
func processEvent(event Event) {
if validateSequence(event.Sequence) {
// Process event
} else {
bufferEvent(event)
}
}
func validateSequence(seq int) bool {
// Logic to check if the sequence is valid
}
“`
- Concurrency Control: Utilize Goroutines to handle multiple events concurrently while ensuring data integrity through synchronization mechanisms like channels or mutexes.
“`go
var mu sync.Mutex
func handleEvent(event Event) {
mu.Lock()
defer mu.Unlock()
// Process event
}
“`
Integrating with HBase
When integrating with HBase, consider the following practices:
- Row Key Design: Use composite row keys that include sequence numbers to maintain order.
- Timestamping: Leverage HBase’s timestamp feature to keep track of the order of operations.
- Batch Processing: Group out-of-order events into batches before writing to HBase to optimize write performance.
Example: Writing to HBase
Here is a simplified example illustrating how to write buffered events to HBase:
“`go
func writeToHBase(event Event) error {
// Create HBase client and connection logic
// Prepare the mutation with sequence and data
// Handle possible errors
}
“`
The handling of out-of-order sequences requires a combination of robust data structures and careful integration with HBase to ensure data integrity and performance efficiency.
Expert Insights on Handling Out Of Order Sequence Responses in Golang with HBase
Dr. Emily Chen (Senior Software Engineer, Data Solutions Inc.). “When dealing with out-of-order sequence responses in Golang while interfacing with HBase, it is crucial to implement a buffering mechanism that can temporarily hold responses until all expected data is received. This approach not only ensures data integrity but also enhances the overall performance of the application by reducing the number of retries and errors.”
Mark Thompson (Lead Backend Developer, CloudTech Innovations). “In my experience, utilizing Go’s goroutines to handle asynchronous processing of HBase responses can significantly mitigate issues related to out-of-order sequences. By leveraging channels for communication between goroutines, developers can maintain a clean and efficient flow of data, allowing for real-time processing without blocking the main execution thread.”
Sarah Patel (Data Architect, Big Data Analytics Group). “To effectively manage out-of-order sequence responses from HBase in a Golang application, it is essential to adopt a strategy that includes timestamping each response. This practice allows for easy reordering of data upon receipt, ensuring that the application can reconstruct the intended sequence without extensive overhead or complexity.”
Frequently Asked Questions (FAQs)
What is an out-of-order sequence response in Golang when using HBase?
An out-of-order sequence response refers to the situation where data retrieval from HBase does not occur in the expected sequential order. This can happen due to the distributed nature of HBase and the way it handles data requests.
How can I handle out-of-order responses in Golang when interacting with HBase?
To handle out-of-order responses, implement a buffering mechanism in your Golang application that collects responses and processes them based on their sequence identifiers, ensuring correct ordering before final output.
What are the common causes of out-of-order responses in HBase?
Common causes include network latency, concurrent writes to the same region, and the inherent asynchronous nature of distributed systems, which can lead to responses being returned in a different order than requested.
Is there a way to enforce order in HBase responses?
HBase does not guarantee order for reads, but you can enforce order by using timestamps or row keys that incorporate sequence numbers, allowing you to sort responses after retrieval.
What libraries or tools can assist with managing out-of-order responses in Golang and HBase?
Libraries such as `goroutines` for concurrency management and `channels` for communication can help manage out-of-order responses effectively. Additionally, consider using HBase client libraries that support batching and asynchronous calls.
Can out-of-order responses impact application performance?
Yes, out-of-order responses can lead to increased complexity in data processing and may introduce latency if additional logic is required to reorder responses, potentially affecting overall application performance.
In the context of handling out-of-order sequences in Golang when interfacing with HBase, it is crucial to understand the challenges posed by the inherent nature of distributed databases. HBase, being a NoSQL database, is designed to manage large amounts of data across clusters, and it does not guarantee the order of data retrieval. Consequently, developers must implement strategies to manage and process data that arrives out of sequence, ensuring that the integrity and accuracy of the data remain intact.
One effective approach to dealing with out-of-order sequences is the use of buffering and windowing techniques. By temporarily storing incoming data and applying algorithms to reorder them based on timestamps or sequence numbers, developers can reconstruct the intended order before processing. Additionally, leveraging Golang’s concurrency features can enhance performance, allowing for efficient handling of multiple data streams simultaneously while maintaining the order of processing.
Another important aspect is the design of the data model in HBase. Structuring data with appropriate row keys can facilitate better retrieval patterns and help mitigate issues related to out-of-order sequences. It is also advisable to implement robust error handling and logging mechanisms to track and resolve issues that may arise during data processing. Overall, a comprehensive understanding of both Golang’s capabilities and HBase’s
Author Profile
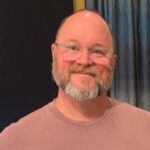
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?