How Can You Safely Ignore Warnings in Python?
### How To Ignore Warnings In Python
In the world of programming, warnings can often feel like pesky distractions, interrupting the flow of your code with messages that may not always be relevant to your immediate tasks. Python, with its robust ecosystem and extensive libraries, generates warnings for various reasons—be it deprecated functions, potential issues in your code, or even best practices that you might choose to overlook. While these warnings serve a purpose, there are times when you might want to silence them to maintain focus on your project. This article delves into the art of ignoring warnings in Python, empowering you to take control of your coding environment.
Understanding how to manage warnings effectively is crucial for developers who want to streamline their workflow. Python provides built-in mechanisms to filter and suppress warnings, allowing you to customize your experience based on the context of your work. Whether you’re running a complex data analysis or developing a web application, knowing how to ignore irrelevant warnings can enhance your productivity and reduce unnecessary clutter in your console output.
Moreover, while it’s essential to recognize the importance of warnings in maintaining code quality, there are legitimate scenarios where ignoring them becomes necessary. This article will guide you through the methods available in Python to suppress these messages, ensuring that you can focus on what truly matters—
Using the Warnings Module
Python provides a built-in module called `warnings` that allows developers to manage warning messages. This module gives you control over how warnings are issued, filtered, and ignored. You can choose to ignore specific warnings or even suppress all warnings entirely, depending on your requirements.
To ignore warnings, you can use the `filterwarnings` function. Here’s how you can implement it:
python
import warnings
# Ignore all warnings
warnings.filterwarnings(“ignore”)
# Ignore a specific warning category
warnings.filterwarnings(“ignore”, category=UserWarning)
The `filterwarnings` method takes several parameters, including the action to take and the category of warning to ignore. The action can be:
- `”ignore”`: Suppresses the warning.
- `”always”`: Always show the warning.
- `”default”`: Show the warning once per location where it was issued.
- `”error”`: Turn the warning into an exception.
Suppressing Warnings in Context
Sometimes, you may want to ignore warnings only within a specific block of code rather than globally. The `warnings` module provides a context manager for this purpose.
Here’s an example:
python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
# Code that may produce warnings
result = some_function_that_warns()
This method allows you to temporarily suppress warnings for the duration of the `with` block, ensuring that the rest of your code remains unaffected.
Ignoring Specific Warnings
If you want to ignore specific warnings, you can capture their message and filter them accordingly. For example:
python
import warnings
def my_function():
warnings.warn(“This is a warning”, UserWarning)
# Ignoring a specific warning message
with warnings.catch_warnings(record=True) as w:
warnings.simplefilter(“always”)
my_function()
for warning in w:
if “This is a warning” in str(warning.message):
continue # Ignore this specific warning
print(warning.message)
This approach can be particularly useful when you need to maintain visibility of other warnings while ignoring only the ones you deem unnecessary.
Table of Warning Filters
Here is a summary of the common warning filters you can apply using the `warnings` module:
Action | Description |
---|---|
ignore | Suppress the warning |
always | Always show the warning |
default | Show the warning once per location |
error | Convert the warning into an exception |
By utilizing these techniques, you can effectively manage warnings in your Python applications, ensuring that they do not clutter your output or hinder your development process.
Understanding Warnings in Python
Warnings in Python serve as alerts for potentially problematic code that may not necessarily cause an error but could lead to issues in the future. Python’s built-in `warnings` module provides a framework to issue warnings, which can help developers identify deprecated features or bad practices. However, in certain situations, developers may want to suppress these warnings.
Suppressing Warnings Using the Warnings Module
To ignore warnings in Python, you can utilize the `warnings` module. This module allows you to customize how warnings are handled, including the option to ignore them altogether.
Here’s how to ignore warnings:
python
import warnings
# Ignore all warnings
warnings.filterwarnings(“ignore”)
This code snippet sets the filter to ignore all warnings throughout the program. It is important to note that suppressing all warnings might lead to overlooking significant issues in the code.
Selective Ignoring of Warnings
In some cases, you may want to ignore only specific types of warnings. The `filterwarnings` method allows for more granular control:
python
# Ignore a specific warning type
warnings.filterwarnings(“ignore”, category=DeprecationWarning)
### Common Warning Categories:
- `DeprecationWarning`: Alerts about deprecated features.
- `SyntaxWarning`: Warns about dubious syntax.
- `RuntimeWarning`: Indicates runtime issues.
By specifying the category, you can focus on ignoring only those warnings that are not relevant to your current development needs.
Using Context Managers to Suppress Warnings
Another approach to suppress warnings temporarily is by using a context manager. This is useful when you want to ignore warnings only in a specific block of code:
python
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
# Code that generates warnings
Within this block, any warnings that would normally be raised will be suppressed, allowing for cleaner output without permanently changing the warning filter for the entire script.
Command-Line Options to Ignore Warnings
Python also provides command-line options that can be used to ignore warnings when executing a script. This can be useful for quick testing without modifying the code.
You can run your script with the following command:
bash
python -W ignore script.py
This will ignore all warnings generated by `script.py` during execution.
Best Practices for Ignoring Warnings
While suppressing warnings may be necessary at times, it is advisable to follow best practices:
- Use selective filtering: Avoid suppressing all warnings. Instead, target specific categories that are not relevant.
- Review warnings regularly: Periodically check suppressed warnings to ensure important issues are not being overlooked.
- Document reasons for suppression: If you choose to ignore certain warnings, document the reasons clearly in your code for future reference.
By adhering to these best practices, you maintain code quality while managing warnings effectively.
Expert Insights on Ignoring Warnings in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Ignoring warnings in Python can be a double-edged sword. While it may streamline development in the short term, it is crucial to understand the underlying issues that these warnings indicate. Developers should prioritize addressing the root causes rather than simply silencing the warnings.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “In certain scenarios, such as during rapid prototyping or when working with legacy code, it may be necessary to ignore warnings. However, I recommend using the `warnings` module to selectively filter warnings rather than suppressing them entirely, as this allows for better control and awareness of potential issues.”
Sarah Thompson (Data Scientist, Analytics Hub). “Ignoring warnings can lead to unexpected behavior in data-driven applications. It is essential to document any warnings that are intentionally ignored, as this practice aids in maintaining code quality and facilitates future debugging efforts.”
Frequently Asked Questions (FAQs)
How can I suppress warnings in Python?
You can suppress warnings in Python by using the `warnings` module. Specifically, you can call `warnings.filterwarnings(‘ignore’)` to ignore all warnings or specify particular warning categories to suppress.
Is it safe to ignore warnings in Python?
Ignoring warnings can be safe in certain contexts, especially if you are aware of the implications. However, it is generally advisable to address the underlying issues causing the warnings to ensure code reliability and maintainability.
Can I ignore specific types of warnings in Python?
Yes, you can ignore specific warning types by using the `warnings.filterwarnings()` function. For example, `warnings.filterwarnings(‘ignore’, category=UserWarning)` will suppress only `UserWarning` messages.
How do I temporarily ignore warnings in a specific block of code?
You can temporarily ignore warnings in a specific block of code by using the `warnings` module’s context manager. For example:
python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(‘ignore’)
# Your code here
What is the difference between warnings and exceptions in Python?
Warnings are notifications about potential issues in your code that do not stop execution, while exceptions are errors that occur during execution and can halt the program unless handled. Warnings indicate something that may need attention, whereas exceptions indicate a failure that requires resolution.
Where can I find more information about handling warnings in Python?
You can find more information about handling warnings in the official Python documentation under the `warnings` module section. It provides detailed guidance on filtering, customizing, and managing warnings effectively.
In Python, warnings serve as important indicators of potential issues in code execution. However, there are scenarios where developers may wish to suppress these warnings to maintain a clean output or focus on critical errors. The primary method for ignoring warnings involves utilizing the built-in `warnings` module, which provides functions such as `warnings.filterwarnings()` to control the visibility of specific warnings or all warnings altogether. This flexibility allows developers to tailor the warning output to their needs while ensuring that significant issues are still addressed appropriately.
Another approach to managing warnings is through context management. By employing the `warnings.catch_warnings()` context manager, developers can temporarily alter warning settings within a specific block of code. This method is particularly useful when dealing with legacy code or third-party libraries that may generate numerous warnings, allowing for a more streamlined output without permanently suppressing warnings across the entire application.
It is crucial to exercise caution when ignoring warnings, as they often provide valuable insights into potential problems that could affect the functionality or performance of the code. Ignoring warnings indiscriminately can lead to overlooking critical issues that may result in runtime errors or unexpected behavior. Therefore, while it is possible to suppress warnings, developers should carefully consider the implications and ensure that they are not compromising the
Author Profile
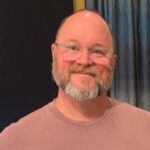
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?