How Can You Train an AI Assistant Using JavaScript?
In the rapidly evolving landscape of technology, artificial intelligence (AI) has emerged as a transformative force, reshaping how we interact with digital tools and services. Among the various programming languages that facilitate AI development, JavaScript stands out due to its versatility and widespread use in web applications. If you’ve ever wondered how to harness the power of AI within your JavaScript projects, you’re in the right place. This article will guide you through the essentials of training an AI assistant using JavaScript, empowering you to create intelligent applications that can understand and respond to user inputs seamlessly.
As we delve into the intricacies of training an AI assistant in JavaScript, it’s essential to grasp the foundational concepts that underpin AI and machine learning. From understanding data preprocessing techniques to exploring various algorithms, you’ll learn how to equip your AI with the ability to learn from experience and improve over time. JavaScript’s unique capabilities, especially in conjunction with libraries like TensorFlow.js and Brain.js, open up a world of possibilities for developers looking to integrate AI functionalities into their applications.
Throughout this article, we’ll explore the critical steps involved in training an AI assistant, including data collection, model training, and implementation. Whether you’re a seasoned developer or a curious beginner, you’ll find valuable insights that will not only enhance your
Setting Up Your JavaScript Environment
To begin training an AI assistant using JavaScript, you need to establish a suitable environment. This typically includes setting up Node.js, which allows you to run JavaScript on the server side, and a package manager like npm or yarn to manage dependencies.
- Install Node.js: Download and install Node.js from the official website. This will also install npm.
- Create a Project Directory: Use the command line to create a new directory for your project and navigate into it:
“`bash
mkdir ai-assistant
cd ai-assistant
“`
- Initialize the Project: Run the following command to create a `package.json` file:
“`bash
npm init -y
“`
- Install Dependencies: Depending on your AI assistant’s requirements, you might need libraries such as TensorFlow.js or Natural. Install them via npm:
“`bash
npm install @tensorflow/tfjs natural
“`
Understanding Data Preparation
Data preparation is a crucial step in training your AI assistant. The quality and structure of your data will significantly affect the performance of your model. Follow these guidelines:
- Data Collection: Gather relevant datasets that your assistant will use to learn. This could include conversation logs, intents, and responses.
- Data Cleaning: Ensure your data is clean by removing duplicates, correcting errors, and filtering irrelevant information.
- Data Formatting: Format your data into a suitable structure. For instance, if you’re training a chatbot, you might want to represent your data as pairs of questions and answers.
A sample data structure could be represented in JSON format:
“`json
[
{
“intent”: “greeting”,
“patterns”: [“Hi”, “Hello”, “How are you?”],
“responses”: [“Hello!”, “Hi there!”, “How can I help you?”]
},
{
“intent”: “goodbye”,
“patterns”: [“Bye”, “See you later”, “Goodbye”],
“responses”: [“Goodbye!”, “See you later!”, “Have a great day!”]
}
]
“`
Model Training and Evaluation
Once your data is prepared, the next step is to train your AI model. Here’s how to approach this:
- Choose a Model: Depending on your requirements, select a machine learning model. For chatbots, recurrent neural networks (RNNs) or transformers are popular choices.
- Training the Model: Use TensorFlow.js to build and train your model. Here’s a simplified example:
“`javascript
const tf = require(‘@tensorflow/tfjs’);
// Define your model
const model = tf.sequential();
model.add(tf.layers.dense({ units: 10, activation: ‘relu’, inputShape: [inputSize] }));
model.add(tf.layers.dense({ units: outputSize, activation: ‘softmax’ }));
model.compile({ optimizer: ‘adam’, loss: ‘categoricalCrossentropy’, metrics: [‘accuracy’] });
// Train the model
await model.fit(trainingData, trainingLabels, { epochs: 100 });
“`
- Evaluate Performance: After training, evaluate your model’s performance using a validation set. You can measure accuracy, precision, and recall.
Metric | Description |
---|---|
Accuracy | Proportion of correct predictions |
Precision | Proportion of true positive predictions in all positive predictions |
Recall | Proportion of true positive predictions in all actual positives |
Implementing the AI Assistant
Once the model is trained and evaluated, the next step is to implement the AI assistant in your application. This involves integrating the model with your front-end or server-side application, depending on your architecture.
- API Development: Create an API endpoint that your application can call to interact with the AI model. You can use frameworks like Express.js to set up your server.
- User Interaction: Design the user interface for users to input queries and receive responses. This can be a web app or a chatbot integrated into messaging platforms.
- Continuous Learning: Implement a mechanism for your AI assistant to learn from new interactions. Store user queries and feedback to retrain your model periodically.
By following these steps, you can effectively train and deploy an AI assistant using JavaScript, ensuring it meets user needs and adapts over time.
Understanding the Basics of AI Training
Training an AI assistant involves several foundational concepts. It is essential to comprehend the data processing, machine learning models, and how these elements integrate into a JavaScript environment.
- Data Collection: Collect diverse datasets relevant to the tasks your AI will perform. This includes:
- Text data from various sources
- User interaction logs
- Domain-specific databases
- Preprocessing: Clean and prepare your data. Common preprocessing steps include:
- Tokenization: Breaking down text into words or phrases
- Normalization: Converting to a common format (e.g., lowercasing)
- Removing stop words: Eliminating common words that add little value
- Feature Extraction: Identify the most relevant features of your dataset. Techniques include:
- Bag-of-Words
- TF-IDF (Term Frequency-Inverse Document Frequency)
Setting Up Your JavaScript Environment
To start training an AI assistant using JavaScript, choose the right tools and libraries.
- Node.js: A JavaScript runtime for server-side programming, essential for handling requests and data processing.
- TensorFlow.js: A library for machine learning that allows you to build and train models directly in JavaScript.
- Natural: A natural language processing (NLP) library in JavaScript designed for text analysis.
Building the AI Model
Creating an AI model involves defining the architecture and training it with the prepared data.
- Model Selection: Choose a model architecture based on your needs:
- Sequential Models: For simple tasks
- Functional API: For more complex architectures involving multiple inputs or outputs
- Training the Model: Use TensorFlow.js to train your model with the following steps:
- Define the model using layers (e.g., Dense, LSTM).
- Compile the model with an optimizer (e.g., Adam) and loss function (e.g., categorical crossentropy).
- Fit the model to your data, specifying the number of epochs and batch size.
“`javascript
const model = tf.sequential();
model.add(tf.layers.dense({ units: 32, activation: ‘relu’, inputShape: [inputSize] }));
model.add(tf.layers.dense({ units: outputSize, activation: ‘softmax’ }));
model.compile({ optimizer: ‘adam’, loss: ‘categoricalCrossentropy’ });
await model.fit(trainingData, trainingLabels, { epochs: 50, batchSize: 32 });
“`
Integrating the AI Assistant
Once the model is trained, you need to integrate it with your application.
- Deployment: Deploy your AI model using frameworks like Express.js to create an API that can serve predictions.
- User Interaction: Implement a user interface (UI) that allows users to interact with your AI assistant. Consider:
- Input fields for user queries
- Display areas for responses
- Feedback mechanisms for improving AI performance
Continuous Learning and Improvement
To enhance the AI assistant over time, implement mechanisms for continuous learning.
- User Feedback: Gather user interactions and feedback to refine model responses.
- Retraining: Periodically retrain your model with new data to keep it up-to-date.
- Performance Monitoring: Track key performance indicators (KPIs) such as accuracy, response time, and user satisfaction to guide improvements.
KPI | Description |
---|---|
Accuracy | Percentage of correct responses |
Response Time | Average time taken to respond |
User Satisfaction | Rating of user experience |
Expert Insights on Training AI Assistants Using JavaScript
Dr. Emily Carter (AI Research Scientist, Tech Innovations Inc.). “Training an AI assistant in JavaScript requires a solid understanding of both machine learning principles and JavaScript frameworks. Utilizing libraries like TensorFlow.js can significantly enhance the model’s capabilities, allowing for real-time data processing and user interaction.”
Mark Thompson (Lead Software Engineer, FutureTech Labs). “When developing an AI assistant in JavaScript, it is crucial to focus on the architecture of the application. Implementing a modular design will facilitate easier updates and maintenance, ensuring the AI can adapt to new requirements over time.”
Linda Zhang (Senior Data Scientist, AI Solutions Group). “Data quality is paramount when training AI assistants. Collecting diverse and representative datasets will improve the assistant’s understanding and response accuracy. Additionally, continuous training and user feedback loops are essential for refining its performance.”
Frequently Asked Questions (FAQs)
What are the basic steps to train an AI assistant in JavaScript?
To train an AI assistant in JavaScript, you should first define the assistant’s purpose, gather training data, preprocess the data, select a suitable machine learning library, implement the model, and then evaluate its performance through testing and refinement.
Which JavaScript libraries are recommended for AI training?
Popular JavaScript libraries for AI training include TensorFlow.js, Brain.js, and Synaptic. These libraries provide tools for neural networks and machine learning algorithms, facilitating the development of intelligent applications.
How can I gather training data for my AI assistant?
Training data can be collected through various methods such as web scraping, utilizing existing datasets, conducting surveys, or generating synthetic data. Ensure the data is relevant and diverse to improve the assistant’s performance.
What is the role of natural language processing (NLP) in training an AI assistant?
Natural language processing is crucial for understanding and generating human language. It allows the AI assistant to interpret user queries, respond appropriately, and improve its conversational abilities through techniques like tokenization, sentiment analysis, and intent recognition.
How do I evaluate the performance of my AI assistant?
Performance can be evaluated using metrics such as accuracy, precision, recall, and F1 score. Additionally, user feedback and real-world testing are essential to assess the assistant’s effectiveness and make necessary adjustments.
Can I integrate my trained AI assistant with other applications?
Yes, integrating your trained AI assistant with other applications is possible. You can use APIs to connect your assistant to web services, chat platforms, or mobile applications, enhancing its functionality and user interaction.
Training an AI assistant in JavaScript involves several key components, including understanding the underlying technologies, selecting appropriate libraries, and implementing effective training methodologies. JavaScript, being a versatile language, allows developers to leverage various frameworks and tools such as TensorFlow.js or Brain.js, which facilitate the creation and training of machine learning models directly in the browser or on the server side. This capability enables developers to build interactive and responsive AI applications that can learn from user interactions.
Moreover, it is essential to gather and preprocess data effectively, as the quality of the training data significantly impacts the performance of the AI assistant. Developers must focus on collecting relevant datasets, cleaning the data, and ensuring it is representative of the tasks the AI will perform. Additionally, implementing proper training algorithms and tuning hyperparameters are crucial steps in refining the model’s accuracy and efficiency.
Finally, continuous evaluation and iteration are vital in the training process. Developers should regularly assess the AI assistant’s performance using metrics such as accuracy, precision, and recall. Feedback loops that incorporate user interactions can further enhance the assistant’s learning process, allowing it to adapt to user preferences and improve over time. By following these guidelines, developers can effectively train AI assistants in JavaScript, leading to more intelligent and
Author Profile
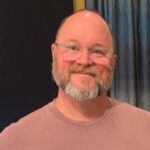
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?