Why Am I Seeing ‘0: Error: Soap Struct Not Initialized’ and How Can I Fix It?
In the intricate world of software development, errors can often feel like insurmountable obstacles, halting progress and frustrating developers. Among these hurdles, the cryptic message `0: Error: Soap Struct Not Initialized` stands out, particularly for those working with SOAP (Simple Object Access Protocol) web services. This error can be a source of confusion, especially for newcomers who may not fully grasp the underlying principles of SOAP and how data structures are initialized within this framework. Understanding this error is crucial for anyone looking to build robust web services and ensure seamless communication between different systems.
The `Soap Struct Not Initialized` error typically arises when a SOAP client attempts to access or manipulate a data structure that has not been properly set up. This can lead to a cascade of issues, impacting the functionality of applications that rely on web services for data exchange. As developers navigate the complexities of SOAP, they must be equipped with the knowledge to diagnose and resolve such errors efficiently. This article will delve into the causes of this specific error, offering insights into best practices for initializing SOAP structures and troubleshooting common pitfalls.
By unpacking the nuances of the `Soap Struct Not Initialized` error, we aim to empower developers with the tools and understanding necessary to tackle this challenge head-on. Whether you are
Error: Soap Struct Not Initialized
The error message “Soap Struct Not Initialized” typically indicates that there is an issue with the initialization of a SOAP (Simple Object Access Protocol) structure in a web service communication process. This problem arises when the SOAP client attempts to use an object that has not been properly instantiated or configured.
There are several common causes for this error:
– **Incomplete Configuration**: The SOAP structure may not have been fully set up before use.
– **Incorrect Namespace**: The SOAP client might be referencing a namespace that does not correspond to the expected service.
– **Serialization Issues**: If the data being sent or received is not properly serialized, it can lead to initialization errors.
– **Dependency Problems**: Missing or misconfigured dependencies required by the SOAP client can also trigger this error.
To resolve this issue, it is essential to ensure that the SOAP struct is initialized correctly. Here are steps to troubleshoot and fix the error:
- **Check Initialization Code**: Review the code responsible for initializing the SOAP struct. Make sure that all required properties are set before the struct is used.
- **Validate Configuration**: Ensure that all necessary configurations, such as endpoint URLs and credentials, are correctly specified.
- **Inspect SOAP Headers**: Check if the SOAP headers are properly configured and included in the requests.
- **Debugging**: Use debugging tools to trace the execution flow and identify where the initialization fails.
- **Error Logging**: Implement comprehensive error logging to capture detailed information about the state of the SOAP struct at the time of the error.
Here is a sample code snippet that illustrates proper initialization of a SOAP struct:
“`php
$soapClient = new SoapClient(“http://example.com/service?wsdl”);
$soapStruct = new stdClass();
$soapStruct->property1 = “value1”;
$soapStruct->property2 = “value2”;
try {
$response = $soapClient->SomeMethod($soapStruct);
} catch (SoapFault $fault) {
// Handle error
echo “Error: ” . $fault->getMessage();
}
“`
Best Practices for SOAP Struct Initialization
To prevent encountering the “Soap Struct Not Initialized” error, follow these best practices:
- Use Strong Typing: Define clear data types for all SOAP structures. This helps in preventing serialization issues.
- Follow WSDL Guidelines: Ensure that the definitions in the WSDL (Web Services Description Language) match the implementation.
- Test in Isolation: Conduct unit tests to validate the initialization process independently of other components.
- Keep Documentation: Maintain documentation regarding the expected structure and initialization parameters for SOAP requests.
Issue | Resolution |
---|---|
Incomplete Initialization | Verify all necessary properties are set. |
Incorrect Namespace | Check and correct the namespace definitions. |
Serialization Issues | Ensure proper serialization of data. |
Dependency Problems | Confirm all required libraries and dependencies are available. |
By adhering to these practices and troubleshooting steps, developers can significantly reduce the likelihood of encountering the “Soap Struct Not Initialized” error during SOAP communications.
Understanding the Error Message
The error message `0: Error: Soap Struct Not Initialized` indicates that there is a failure in initializing a SOAP structure in your application. This typically occurs in environments that utilize SOAP for web services, suggesting that the application is attempting to access or manipulate a SOAP structure that has not been properly instantiated.
Common Causes of the Error
- Uninitialized Variables: The SOAP structure or object may not have been created before being used.
- Incorrect Configuration: Misconfigurations in SOAP settings may prevent proper initialization.
- Faulty Code Logic: Conditional paths in your code might bypass the initialization step.
- Library Issues: Bugs or version mismatches in the SOAP library could lead to this error.
Troubleshooting Steps
To effectively address the `Soap Struct Not Initialized` error, follow these troubleshooting steps:
- Check Initialization:
- Ensure that all SOAP structures are initialized before their use.
- Verify that constructors are being called correctly.
- Examine Code Paths:
- Review conditional logic to ensure that no execution paths skip initialization.
- Utilize debugging tools to trace the execution flow leading to the error.
- Review SOAP Configuration:
- Inspect the configuration files for misconfigurations.
- Validate endpoint URLs and authentication credentials.
- Update Libraries:
- Confirm that you are using the latest version of the SOAP libraries.
- Review release notes for any bugs related to initialization.
- Consult Documentation:
- Refer to the SOAP library documentation for proper usage patterns.
- Look for examples demonstrating correct initialization practices.
Code Example
Here is a simple example demonstrating proper initialization of a SOAP structure in PHP:
“`php
// Proper initialization of SOAP client
try {
$client = new SoapClient(“http://example.com/service?wsdl”);
// Ensure the SOAP struct is initialized
$params = new stdClass();
$params->param1 = “value1”;
$params->param2 = “value2”;
// Invoke the SOAP method with initialized parameters
$response = $client->__soapCall(“SomeMethod”, array($params));
} catch (SoapFault $fault) {
echo “Error: ” . $fault->getMessage();
}
“`
Key Points in the Example
- The `SoapClient` is instantiated before any method calls.
- Parameters are initialized in a structured format.
- Exception handling captures any SOAP-related errors.
Preventative Measures
To avoid encountering the `Soap Struct Not Initialized` error in the future, consider implementing the following measures:
- Code Reviews: Regularly review code to ensure all objects are initialized properly.
- Unit Testing: Develop comprehensive unit tests that cover various scenarios, including edge cases where initialization might fail.
- Documentation Practices: Maintain clear documentation for the SOAP services you interact with, including expected data structures.
Preventative Measure | Description |
---|---|
Code Reviews | Ensure that all initializations are accounted for. |
Unit Testing | Validate code behavior under different conditions. |
Documentation Practices | Keep thorough records of API structures and usage. |
By adhering to these guidelines, developers can minimize the occurrence of the `Soap Struct Not Initialized` error and ensure more robust SOAP service interactions.
Understanding the ‘Soap Struct Not Initialized’ Error in Web Services
Dr. Emily Carter (Senior Software Architect, CloudTech Solutions). “The ‘Soap Struct Not Initialized’ error typically indicates that there is a failure in the serialization process of the SOAP message. This can occur when the expected data structure is not properly instantiated before being sent over the network, leading to communication breakdowns between services.”
Michael Tran (Lead Developer, IntegrateX Systems). “To resolve the ‘Soap Struct Not Initialized’ error, developers should ensure that all necessary fields of the SOAP struct are initialized correctly. This includes checking for null values and ensuring that the data types match the expected schema defined in the WSDL.”
Sarah Johnson (Technical Consultant, Web Services Insights). “In many cases, the ‘Soap Struct Not Initialized’ error can be traced back to mismatches between the client and server configurations. It is crucial to validate that both sides are aligned in terms of the expected data structures and that the SOAP envelopes are constructed correctly before transmission.”
Frequently Asked Questions (FAQs)
What does the error “0: Error: Soap Struct Not Initialized” indicate?
This error typically indicates that a SOAP (Simple Object Access Protocol) struct has not been properly initialized before it is used in a request or response. It suggests that the expected data structure is missing or improperly configured.
What causes the “Soap Struct Not Initialized” error?
The error can be caused by several factors, including incorrect SOAP client configuration, missing required parameters, or issues with the server-side implementation that fails to initialize the expected data structures.
How can I troubleshoot the “0: Error: Soap Struct Not Initialized” error?
To troubleshoot, ensure that all required fields are populated in the SOAP request. Check the client-side code for proper initialization of the SOAP struct and review server-side logs for any indications of configuration issues.
Is there a way to prevent this error from occurring?
Yes, to prevent this error, ensure that all necessary data structures are initialized before use. Implement error handling to catch uninitialized structures and validate input data before making SOAP calls.
What programming languages are commonly associated with SOAP errors like this?
SOAP errors can occur in various programming languages, including PHP, Java, .NET, and Python, as they all have libraries or frameworks that support SOAP web services.
Where can I find more information on handling SOAP errors?
More information can be found in the documentation for the specific SOAP library or framework you are using. Additionally, online forums, community discussions, and technical blogs often provide insights and solutions for common SOAP-related issues.
The error message “0: Error: Soap Struct Not Initialized” typically indicates an issue with the initialization of a SOAP structure in programming environments that utilize SOAP for web services. This error often arises when there is an attempt to access or manipulate a SOAP struct that has not been properly instantiated, leading to potential disruptions in the execution of web service calls. Understanding the context in which this error occurs is crucial for developers working with SOAP APIs, as it can affect the reliability and functionality of their applications.
One of the primary causes of this error is a failure to correctly initialize the SOAP struct before its use. Developers must ensure that all required fields are populated and that the struct is instantiated properly to avoid this error. Additionally, it is important to validate the data being passed to the SOAP service to ensure compatibility with the expected input format. Proper error handling and logging can also assist in diagnosing the root cause of the issue, thereby facilitating a more efficient resolution process.
In summary, addressing the “0: Error: Soap Struct Not Initialized” error requires a thorough understanding of SOAP structures and their initialization processes. By implementing best practices in struct management and data validation, developers can mitigate the risk of encountering this error. Furthermore, maintaining robust error handling mechanisms will enhance
Author Profile
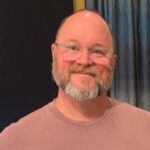
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?